mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-22 17:01:14 +00:00
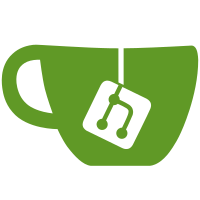
Currently IIO only supports buffer mode for capture devices like ADCs. Add support for buffered mode for output devices like DACs. The output buffer implementation is analogous to the input buffer implementation. Instead of using read() to get data from the buffer write() is used to copy data into the buffer. poll() with POLLOUT will wakeup if there is space available. Drivers can remove data from a buffer using iio_pop_from_buffer(), the function can e.g. called from a trigger handler to write the data to hardware. A buffer can only be either a output buffer or an input, but not both. So, for a device that has an ADC and DAC path, this will mean 2 IIO buffers (one for each direction). The direction of the buffer is decided by the new direction field of the iio_buffer struct and should be set after allocating and before registering it. Co-developed-by: Lars-Peter Clausen <lars@metafoo.de> Signed-off-by: Lars-Peter Clausen <lars@metafoo.de> Co-developed-by: Alexandru Ardelean <alexandru.ardelean@analog.com> Signed-off-by: Alexandru Ardelean <alexandru.ardelean@analog.com> Signed-off-by: Mihail Chindris <mihail.chindris@analog.com> Link: https://lore.kernel.org/r/20211007080035.2531-2-mihail.chindris@analog.com Signed-off-by: Jonathan Cameron <Jonathan.Cameron@huawei.com>
58 lines
1.8 KiB
C
58 lines
1.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/* The industrial I/O core - generic buffer interfaces.
|
|
*
|
|
* Copyright (c) 2008 Jonathan Cameron
|
|
*/
|
|
|
|
#ifndef _IIO_BUFFER_GENERIC_H_
|
|
#define _IIO_BUFFER_GENERIC_H_
|
|
#include <linux/sysfs.h>
|
|
#include <linux/iio/iio.h>
|
|
|
|
struct iio_buffer;
|
|
|
|
enum iio_buffer_direction {
|
|
IIO_BUFFER_DIRECTION_IN,
|
|
IIO_BUFFER_DIRECTION_OUT,
|
|
};
|
|
|
|
int iio_push_to_buffers(struct iio_dev *indio_dev, const void *data);
|
|
|
|
int iio_pop_from_buffer(struct iio_buffer *buffer, void *data);
|
|
|
|
/**
|
|
* iio_push_to_buffers_with_timestamp() - push data and timestamp to buffers
|
|
* @indio_dev: iio_dev structure for device.
|
|
* @data: sample data
|
|
* @timestamp: timestamp for the sample data
|
|
*
|
|
* Pushes data to the IIO device's buffers. If timestamps are enabled for the
|
|
* device the function will store the supplied timestamp as the last element in
|
|
* the sample data buffer before pushing it to the device buffers. The sample
|
|
* data buffer needs to be large enough to hold the additional timestamp
|
|
* (usually the buffer should be indio->scan_bytes bytes large).
|
|
*
|
|
* Returns 0 on success, a negative error code otherwise.
|
|
*/
|
|
static inline int iio_push_to_buffers_with_timestamp(struct iio_dev *indio_dev,
|
|
void *data, int64_t timestamp)
|
|
{
|
|
if (indio_dev->scan_timestamp) {
|
|
size_t ts_offset = indio_dev->scan_bytes / sizeof(int64_t) - 1;
|
|
((int64_t *)data)[ts_offset] = timestamp;
|
|
}
|
|
|
|
return iio_push_to_buffers(indio_dev, data);
|
|
}
|
|
|
|
int iio_push_to_buffers_with_ts_unaligned(struct iio_dev *indio_dev,
|
|
const void *data, size_t data_sz,
|
|
int64_t timestamp);
|
|
|
|
bool iio_validate_scan_mask_onehot(struct iio_dev *indio_dev,
|
|
const unsigned long *mask);
|
|
|
|
int iio_device_attach_buffer(struct iio_dev *indio_dev,
|
|
struct iio_buffer *buffer);
|
|
|
|
#endif /* _IIO_BUFFER_GENERIC_H_ */
|