mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-22 18:41:06 +00:00
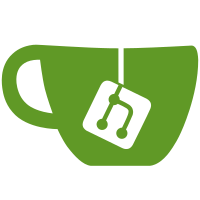
The .remove() callback for a platform driver returns an int which makes many driver authors wrongly assume it's possible to do error handling by returning an error code. However the value returned is ignored (apart from emitting a warning) and this typically results in resource leaks. To improve here there is a quest to make the remove callback return void. In the first step of this quest all drivers are converted to .remove_new(), which already returns void. Eventually after all drivers are converted, .remove_new() will be renamed to .remove(). Trivially convert this driver from always returning zero in the remove callback to the void returning variant. Signed-off-by: Uwe Kleine-König <u.kleine-koenig@pengutronix.de> Link: https://lore.kernel.org/r/20231104211501.3676352-26-u.kleine-koenig@pengutronix.de Signed-off-by: Sebastian Reichel <sebastian.reichel@collabora.com>
80 lines
2 KiB
C
80 lines
2 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* Force-disables a regulator to power down a device
|
|
*
|
|
* Michael Klein <michael@fossekall.de>
|
|
*
|
|
* Copyright (C) 2020 Michael Klein
|
|
*
|
|
* Based on the gpio-poweroff driver.
|
|
*/
|
|
#include <linux/delay.h>
|
|
#include <linux/module.h>
|
|
#include <linux/of.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/pm.h>
|
|
#include <linux/regulator/consumer.h>
|
|
|
|
#define TIMEOUT_MS 3000
|
|
|
|
/*
|
|
* Hold configuration here, cannot be more than one instance of the driver
|
|
* since pm_power_off itself is global.
|
|
*/
|
|
static struct regulator *cpu_regulator;
|
|
|
|
static void regulator_poweroff_do_poweroff(void)
|
|
{
|
|
if (cpu_regulator && regulator_is_enabled(cpu_regulator))
|
|
regulator_force_disable(cpu_regulator);
|
|
|
|
/* give it some time */
|
|
mdelay(TIMEOUT_MS);
|
|
|
|
WARN_ON(1);
|
|
}
|
|
|
|
static int regulator_poweroff_probe(struct platform_device *pdev)
|
|
{
|
|
/* If a pm_power_off function has already been added, leave it alone */
|
|
if (pm_power_off != NULL) {
|
|
dev_err(&pdev->dev,
|
|
"%s: pm_power_off function already registered\n",
|
|
__func__);
|
|
return -EBUSY;
|
|
}
|
|
|
|
cpu_regulator = devm_regulator_get(&pdev->dev, "cpu");
|
|
if (IS_ERR(cpu_regulator))
|
|
return PTR_ERR(cpu_regulator);
|
|
|
|
pm_power_off = ®ulator_poweroff_do_poweroff;
|
|
return 0;
|
|
}
|
|
|
|
static void regulator_poweroff_remove(struct platform_device *pdev)
|
|
{
|
|
if (pm_power_off == ®ulator_poweroff_do_poweroff)
|
|
pm_power_off = NULL;
|
|
}
|
|
|
|
static const struct of_device_id of_regulator_poweroff_match[] = {
|
|
{ .compatible = "regulator-poweroff", },
|
|
{},
|
|
};
|
|
MODULE_DEVICE_TABLE(of, of_regulator_poweroff_match);
|
|
|
|
static struct platform_driver regulator_poweroff_driver = {
|
|
.probe = regulator_poweroff_probe,
|
|
.remove_new = regulator_poweroff_remove,
|
|
.driver = {
|
|
.name = "poweroff-regulator",
|
|
.of_match_table = of_regulator_poweroff_match,
|
|
},
|
|
};
|
|
|
|
module_platform_driver(regulator_poweroff_driver);
|
|
|
|
MODULE_AUTHOR("Michael Klein <michael@fossekall.de>");
|
|
MODULE_DESCRIPTION("Regulator poweroff driver");
|
|
MODULE_ALIAS("platform:poweroff-regulator");
|