mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-28 23:24:50 +00:00
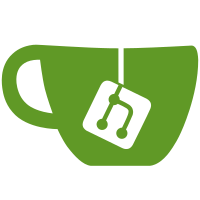
There are a lot of embedded systems that run most or all of their functionality in init, running as root:root. For these systems, supporting multiple users is not necessary. This patch adds a new symbol, CONFIG_MULTIUSER, that makes support for non-root users, non-root groups, and capabilities optional. It is enabled under CONFIG_EXPERT menu. When this symbol is not defined, UID and GID are zero in any possible case and processes always have all capabilities. The following syscalls are compiled out: setuid, setregid, setgid, setreuid, setresuid, getresuid, setresgid, getresgid, setgroups, getgroups, setfsuid, setfsgid, capget, capset. Also, groups.c is compiled out completely. In kernel/capability.c, capable function was moved in order to avoid adding two ifdef blocks. This change saves about 25 KB on a defconfig build. The most minimal kernels have total text sizes in the high hundreds of kB rather than low MB. (The 25k goes down a bit with allnoconfig, but not that much. The kernel was booted in Qemu. All the common functionalities work. Adding users/groups is not possible, failing with -ENOSYS. Bloat-o-meter output: add/remove: 7/87 grow/shrink: 19/397 up/down: 1675/-26325 (-24650) [akpm@linux-foundation.org: coding-style fixes] Signed-off-by: Iulia Manda <iulia.manda21@gmail.com> Reviewed-by: Josh Triplett <josh@joshtriplett.org> Acked-by: Geert Uytterhoeven <geert@linux-m68k.org> Tested-by: Paul E. McKenney <paulmck@linux.vnet.ibm.com> Reviewed-by: Paul E. McKenney <paulmck@linux.vnet.ibm.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
277 lines
5.9 KiB
C
277 lines
5.9 KiB
C
/*
|
|
* Supplementary group IDs
|
|
*/
|
|
#include <linux/cred.h>
|
|
#include <linux/export.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/security.h>
|
|
#include <linux/syscalls.h>
|
|
#include <linux/user_namespace.h>
|
|
#include <asm/uaccess.h>
|
|
|
|
struct group_info *groups_alloc(int gidsetsize)
|
|
{
|
|
struct group_info *group_info;
|
|
int nblocks;
|
|
int i;
|
|
|
|
nblocks = (gidsetsize + NGROUPS_PER_BLOCK - 1) / NGROUPS_PER_BLOCK;
|
|
/* Make sure we always allocate at least one indirect block pointer */
|
|
nblocks = nblocks ? : 1;
|
|
group_info = kmalloc(sizeof(*group_info) + nblocks*sizeof(gid_t *), GFP_USER);
|
|
if (!group_info)
|
|
return NULL;
|
|
group_info->ngroups = gidsetsize;
|
|
group_info->nblocks = nblocks;
|
|
atomic_set(&group_info->usage, 1);
|
|
|
|
if (gidsetsize <= NGROUPS_SMALL)
|
|
group_info->blocks[0] = group_info->small_block;
|
|
else {
|
|
for (i = 0; i < nblocks; i++) {
|
|
kgid_t *b;
|
|
b = (void *)__get_free_page(GFP_USER);
|
|
if (!b)
|
|
goto out_undo_partial_alloc;
|
|
group_info->blocks[i] = b;
|
|
}
|
|
}
|
|
return group_info;
|
|
|
|
out_undo_partial_alloc:
|
|
while (--i >= 0) {
|
|
free_page((unsigned long)group_info->blocks[i]);
|
|
}
|
|
kfree(group_info);
|
|
return NULL;
|
|
}
|
|
|
|
EXPORT_SYMBOL(groups_alloc);
|
|
|
|
void groups_free(struct group_info *group_info)
|
|
{
|
|
if (group_info->blocks[0] != group_info->small_block) {
|
|
int i;
|
|
for (i = 0; i < group_info->nblocks; i++)
|
|
free_page((unsigned long)group_info->blocks[i]);
|
|
}
|
|
kfree(group_info);
|
|
}
|
|
|
|
EXPORT_SYMBOL(groups_free);
|
|
|
|
/* export the group_info to a user-space array */
|
|
static int groups_to_user(gid_t __user *grouplist,
|
|
const struct group_info *group_info)
|
|
{
|
|
struct user_namespace *user_ns = current_user_ns();
|
|
int i;
|
|
unsigned int count = group_info->ngroups;
|
|
|
|
for (i = 0; i < count; i++) {
|
|
gid_t gid;
|
|
gid = from_kgid_munged(user_ns, GROUP_AT(group_info, i));
|
|
if (put_user(gid, grouplist+i))
|
|
return -EFAULT;
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
/* fill a group_info from a user-space array - it must be allocated already */
|
|
static int groups_from_user(struct group_info *group_info,
|
|
gid_t __user *grouplist)
|
|
{
|
|
struct user_namespace *user_ns = current_user_ns();
|
|
int i;
|
|
unsigned int count = group_info->ngroups;
|
|
|
|
for (i = 0; i < count; i++) {
|
|
gid_t gid;
|
|
kgid_t kgid;
|
|
if (get_user(gid, grouplist+i))
|
|
return -EFAULT;
|
|
|
|
kgid = make_kgid(user_ns, gid);
|
|
if (!gid_valid(kgid))
|
|
return -EINVAL;
|
|
|
|
GROUP_AT(group_info, i) = kgid;
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
/* a simple Shell sort */
|
|
static void groups_sort(struct group_info *group_info)
|
|
{
|
|
int base, max, stride;
|
|
int gidsetsize = group_info->ngroups;
|
|
|
|
for (stride = 1; stride < gidsetsize; stride = 3 * stride + 1)
|
|
; /* nothing */
|
|
stride /= 3;
|
|
|
|
while (stride) {
|
|
max = gidsetsize - stride;
|
|
for (base = 0; base < max; base++) {
|
|
int left = base;
|
|
int right = left + stride;
|
|
kgid_t tmp = GROUP_AT(group_info, right);
|
|
|
|
while (left >= 0 && gid_gt(GROUP_AT(group_info, left), tmp)) {
|
|
GROUP_AT(group_info, right) =
|
|
GROUP_AT(group_info, left);
|
|
right = left;
|
|
left -= stride;
|
|
}
|
|
GROUP_AT(group_info, right) = tmp;
|
|
}
|
|
stride /= 3;
|
|
}
|
|
}
|
|
|
|
/* a simple bsearch */
|
|
int groups_search(const struct group_info *group_info, kgid_t grp)
|
|
{
|
|
unsigned int left, right;
|
|
|
|
if (!group_info)
|
|
return 0;
|
|
|
|
left = 0;
|
|
right = group_info->ngroups;
|
|
while (left < right) {
|
|
unsigned int mid = (left+right)/2;
|
|
if (gid_gt(grp, GROUP_AT(group_info, mid)))
|
|
left = mid + 1;
|
|
else if (gid_lt(grp, GROUP_AT(group_info, mid)))
|
|
right = mid;
|
|
else
|
|
return 1;
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
/**
|
|
* set_groups - Change a group subscription in a set of credentials
|
|
* @new: The newly prepared set of credentials to alter
|
|
* @group_info: The group list to install
|
|
*/
|
|
void set_groups(struct cred *new, struct group_info *group_info)
|
|
{
|
|
put_group_info(new->group_info);
|
|
groups_sort(group_info);
|
|
get_group_info(group_info);
|
|
new->group_info = group_info;
|
|
}
|
|
|
|
EXPORT_SYMBOL(set_groups);
|
|
|
|
/**
|
|
* set_current_groups - Change current's group subscription
|
|
* @group_info: The group list to impose
|
|
*
|
|
* Validate a group subscription and, if valid, impose it upon current's task
|
|
* security record.
|
|
*/
|
|
int set_current_groups(struct group_info *group_info)
|
|
{
|
|
struct cred *new;
|
|
|
|
new = prepare_creds();
|
|
if (!new)
|
|
return -ENOMEM;
|
|
|
|
set_groups(new, group_info);
|
|
return commit_creds(new);
|
|
}
|
|
|
|
EXPORT_SYMBOL(set_current_groups);
|
|
|
|
SYSCALL_DEFINE2(getgroups, int, gidsetsize, gid_t __user *, grouplist)
|
|
{
|
|
const struct cred *cred = current_cred();
|
|
int i;
|
|
|
|
if (gidsetsize < 0)
|
|
return -EINVAL;
|
|
|
|
/* no need to grab task_lock here; it cannot change */
|
|
i = cred->group_info->ngroups;
|
|
if (gidsetsize) {
|
|
if (i > gidsetsize) {
|
|
i = -EINVAL;
|
|
goto out;
|
|
}
|
|
if (groups_to_user(grouplist, cred->group_info)) {
|
|
i = -EFAULT;
|
|
goto out;
|
|
}
|
|
}
|
|
out:
|
|
return i;
|
|
}
|
|
|
|
bool may_setgroups(void)
|
|
{
|
|
struct user_namespace *user_ns = current_user_ns();
|
|
|
|
return ns_capable(user_ns, CAP_SETGID) &&
|
|
userns_may_setgroups(user_ns);
|
|
}
|
|
|
|
/*
|
|
* SMP: Our groups are copy-on-write. We can set them safely
|
|
* without another task interfering.
|
|
*/
|
|
|
|
SYSCALL_DEFINE2(setgroups, int, gidsetsize, gid_t __user *, grouplist)
|
|
{
|
|
struct group_info *group_info;
|
|
int retval;
|
|
|
|
if (!may_setgroups())
|
|
return -EPERM;
|
|
if ((unsigned)gidsetsize > NGROUPS_MAX)
|
|
return -EINVAL;
|
|
|
|
group_info = groups_alloc(gidsetsize);
|
|
if (!group_info)
|
|
return -ENOMEM;
|
|
retval = groups_from_user(group_info, grouplist);
|
|
if (retval) {
|
|
put_group_info(group_info);
|
|
return retval;
|
|
}
|
|
|
|
retval = set_current_groups(group_info);
|
|
put_group_info(group_info);
|
|
|
|
return retval;
|
|
}
|
|
|
|
/*
|
|
* Check whether we're fsgid/egid or in the supplemental group..
|
|
*/
|
|
int in_group_p(kgid_t grp)
|
|
{
|
|
const struct cred *cred = current_cred();
|
|
int retval = 1;
|
|
|
|
if (!gid_eq(grp, cred->fsgid))
|
|
retval = groups_search(cred->group_info, grp);
|
|
return retval;
|
|
}
|
|
|
|
EXPORT_SYMBOL(in_group_p);
|
|
|
|
int in_egroup_p(kgid_t grp)
|
|
{
|
|
const struct cred *cred = current_cred();
|
|
int retval = 1;
|
|
|
|
if (!gid_eq(grp, cred->egid))
|
|
retval = groups_search(cred->group_info, grp);
|
|
return retval;
|
|
}
|
|
|
|
EXPORT_SYMBOL(in_egroup_p);
|