mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-26 10:49:33 +00:00
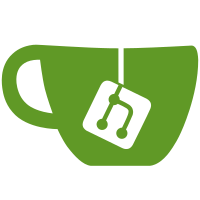
Bus protection is not exclusively done by calling the infracfg misc driver. Make the calls for setting and clearing the bus protection generic so that we can use other blocks for it as well. Signed-off-by: Matthias Brugger <mbrugger@suse.com> Signed-off-by: Enric Balletbo i Serra <enric.balletbo@collabora.com> Link: https://lore.kernel.org/r/20201030113622.201188-6-enric.balletbo@collabora.com Signed-off-by: Matthias Brugger <matthias.bgg@gmail.com>
74 lines
2.2 KiB
C
74 lines
2.2 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* Copyright (c) 2015 Pengutronix, Sascha Hauer <kernel@pengutronix.de>
|
|
*/
|
|
|
|
#include <linux/export.h>
|
|
#include <linux/jiffies.h>
|
|
#include <linux/regmap.h>
|
|
#include <linux/soc/mediatek/infracfg.h>
|
|
#include <asm/processor.h>
|
|
|
|
#define MTK_POLL_DELAY_US 10
|
|
#define MTK_POLL_TIMEOUT (jiffies_to_usecs(HZ))
|
|
|
|
/**
|
|
* mtk_infracfg_set_bus_protection - enable bus protection
|
|
* @infracfg: The infracfg regmap
|
|
* @mask: The mask containing the protection bits to be enabled.
|
|
* @reg_update: The boolean flag determines to set the protection bits
|
|
* by regmap_update_bits with enable register(PROTECTEN) or
|
|
* by regmap_write with set register(PROTECTEN_SET).
|
|
*
|
|
* This function enables the bus protection bits for disabled power
|
|
* domains so that the system does not hang when some unit accesses the
|
|
* bus while in power down.
|
|
*/
|
|
int mtk_infracfg_set_bus_protection(struct regmap *infracfg, u32 mask,
|
|
bool reg_update)
|
|
{
|
|
u32 val;
|
|
int ret;
|
|
|
|
if (reg_update)
|
|
regmap_update_bits(infracfg, INFRA_TOPAXI_PROTECTEN, mask,
|
|
mask);
|
|
else
|
|
regmap_write(infracfg, INFRA_TOPAXI_PROTECTEN_SET, mask);
|
|
|
|
ret = regmap_read_poll_timeout(infracfg, INFRA_TOPAXI_PROTECTSTA1,
|
|
val, (val & mask) == mask,
|
|
MTK_POLL_DELAY_US, MTK_POLL_TIMEOUT);
|
|
|
|
return ret;
|
|
}
|
|
|
|
/**
|
|
* mtk_infracfg_clear_bus_protection - disable bus protection
|
|
* @infracfg: The infracfg regmap
|
|
* @mask: The mask containing the protection bits to be disabled.
|
|
* @reg_update: The boolean flag determines to clear the protection bits
|
|
* by regmap_update_bits with enable register(PROTECTEN) or
|
|
* by regmap_write with clear register(PROTECTEN_CLR).
|
|
*
|
|
* This function disables the bus protection bits previously enabled with
|
|
* mtk_infracfg_set_bus_protection.
|
|
*/
|
|
|
|
int mtk_infracfg_clear_bus_protection(struct regmap *infracfg, u32 mask,
|
|
bool reg_update)
|
|
{
|
|
int ret;
|
|
u32 val;
|
|
|
|
if (reg_update)
|
|
regmap_update_bits(infracfg, INFRA_TOPAXI_PROTECTEN, mask, 0);
|
|
else
|
|
regmap_write(infracfg, INFRA_TOPAXI_PROTECTEN_CLR, mask);
|
|
|
|
ret = regmap_read_poll_timeout(infracfg, INFRA_TOPAXI_PROTECTSTA1,
|
|
val, !(val & mask),
|
|
MTK_POLL_DELAY_US, MTK_POLL_TIMEOUT);
|
|
|
|
return ret;
|
|
}
|