mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-15 23:25:07 +00:00
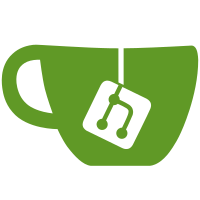
No need to call addr2line since they don't have such information. Signed-off-by: Namhyung Kim <namhyung@kernel.org> Reviewed-by: Jiri Olsa <jolsa@redhat.com> Cc: Ingo Molnar <mingo@kernel.org> Cc: Jiri Olsa <jolsa@redhat.com> Cc: Paul Mackerras <paulus@samba.org> Cc: Peter Zijlstra <a.p.zijlstra@chello.nl> Link: http://lkml.kernel.org/r/1378876173-13363-6-git-send-email-namhyung@kernel.org Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
90 lines
1.6 KiB
C
90 lines
1.6 KiB
C
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
|
|
#include <linux/kernel.h>
|
|
|
|
#include "util/util.h"
|
|
#include "util/debug.h"
|
|
|
|
static int addr2line(const char *dso_name, unsigned long addr,
|
|
char **file, unsigned int *line_nr)
|
|
{
|
|
FILE *fp;
|
|
char cmd[PATH_MAX];
|
|
char *filename = NULL;
|
|
size_t len;
|
|
char *sep;
|
|
int ret = 0;
|
|
|
|
scnprintf(cmd, sizeof(cmd), "addr2line -e %s %016"PRIx64,
|
|
dso_name, addr);
|
|
|
|
fp = popen(cmd, "r");
|
|
if (fp == NULL) {
|
|
pr_warning("popen failed for %s\n", dso_name);
|
|
return 0;
|
|
}
|
|
|
|
if (getline(&filename, &len, fp) < 0 || !len) {
|
|
pr_warning("addr2line has no output for %s\n", dso_name);
|
|
goto out;
|
|
}
|
|
|
|
sep = strchr(filename, '\n');
|
|
if (sep)
|
|
*sep = '\0';
|
|
|
|
if (!strcmp(filename, "??:0")) {
|
|
pr_debug("no debugging info in %s\n", dso_name);
|
|
free(filename);
|
|
goto out;
|
|
}
|
|
|
|
sep = strchr(filename, ':');
|
|
if (sep) {
|
|
*sep++ = '\0';
|
|
*file = filename;
|
|
*line_nr = strtoul(sep, NULL, 0);
|
|
ret = 1;
|
|
}
|
|
out:
|
|
pclose(fp);
|
|
return ret;
|
|
}
|
|
|
|
char *get_srcline(const char *dso_name, unsigned long addr)
|
|
{
|
|
char *file;
|
|
unsigned line;
|
|
char *srcline = SRCLINE_UNKNOWN;
|
|
size_t size;
|
|
|
|
if (dso_name[0] == '[')
|
|
goto out;
|
|
|
|
if (!strncmp(dso_name, "/tmp/perf-", 10))
|
|
goto out;
|
|
|
|
if (!addr2line(dso_name, addr, &file, &line))
|
|
goto out;
|
|
|
|
/* just calculate actual length */
|
|
size = snprintf(NULL, 0, "%s:%u", file, line) + 1;
|
|
|
|
srcline = malloc(size);
|
|
if (srcline)
|
|
snprintf(srcline, size, "%s:%u", file, line);
|
|
else
|
|
srcline = SRCLINE_UNKNOWN;
|
|
|
|
free(file);
|
|
out:
|
|
return srcline;
|
|
}
|
|
|
|
void free_srcline(char *srcline)
|
|
{
|
|
if (srcline && strcmp(srcline, SRCLINE_UNKNOWN) != 0)
|
|
free(srcline);
|
|
}
|