mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-21 01:51:18 +00:00
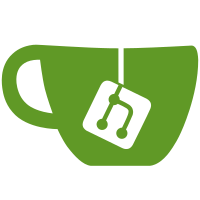
This patch applies the semantic patch: @@ expression I, P, SP; @@ I = devm_iio_device_alloc(P, SP); ... - I->dev.parent = P; It updates 302 files and does 307 deletions. This semantic patch also removes some comments like '/* Establish that the iio_dev is a child of the i2c device */' But this is is only done in case where the block is left empty. The patch does not seem to cover all cases. It looks like in some cases a different variable is used in some cases to assign the parent, but it points to the same reference. In other cases, the block covered by ... may be just too big to be covered by the semantic patch. However, this looks pretty good as well, as it does cover a big bulk of the drivers that should remove the parent assignment. Signed-off-by: Alexandru Ardelean <alexandru.ardelean@analog.com> Signed-off-by: Jonathan Cameron <Jonathan.Cameron@huawei.com>
96 lines
2.3 KiB
C
96 lines
2.3 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Azoteq IQS620AT Temperature Sensor
|
|
*
|
|
* Copyright (C) 2019 Jeff LaBundy <jeff@labundy.com>
|
|
*/
|
|
|
|
#include <linux/device.h>
|
|
#include <linux/iio/iio.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/mfd/iqs62x.h>
|
|
#include <linux/module.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/regmap.h>
|
|
|
|
#define IQS620_TEMP_UI_OUT 0x1A
|
|
|
|
#define IQS620_TEMP_SCALE 1000
|
|
#define IQS620_TEMP_OFFSET (-100)
|
|
|
|
static int iqs620_temp_read_raw(struct iio_dev *indio_dev,
|
|
struct iio_chan_spec const *chan,
|
|
int *val, int *val2, long mask)
|
|
{
|
|
struct iqs62x_core *iqs62x = iio_device_get_drvdata(indio_dev);
|
|
int ret;
|
|
__le16 val_buf;
|
|
|
|
switch (mask) {
|
|
case IIO_CHAN_INFO_RAW:
|
|
ret = regmap_raw_read(iqs62x->regmap, IQS620_TEMP_UI_OUT,
|
|
&val_buf, sizeof(val_buf));
|
|
if (ret)
|
|
return ret;
|
|
|
|
*val = le16_to_cpu(val_buf);
|
|
return IIO_VAL_INT;
|
|
|
|
case IIO_CHAN_INFO_SCALE:
|
|
*val = IQS620_TEMP_SCALE;
|
|
return IIO_VAL_INT;
|
|
|
|
case IIO_CHAN_INFO_OFFSET:
|
|
*val = IQS620_TEMP_OFFSET;
|
|
return IIO_VAL_INT;
|
|
|
|
default:
|
|
return -EINVAL;
|
|
}
|
|
}
|
|
|
|
static const struct iio_info iqs620_temp_info = {
|
|
.read_raw = &iqs620_temp_read_raw,
|
|
};
|
|
|
|
static const struct iio_chan_spec iqs620_temp_channels[] = {
|
|
{
|
|
.type = IIO_TEMP,
|
|
.info_mask_separate = BIT(IIO_CHAN_INFO_RAW) |
|
|
BIT(IIO_CHAN_INFO_SCALE) |
|
|
BIT(IIO_CHAN_INFO_OFFSET),
|
|
},
|
|
};
|
|
|
|
static int iqs620_temp_probe(struct platform_device *pdev)
|
|
{
|
|
struct iqs62x_core *iqs62x = dev_get_drvdata(pdev->dev.parent);
|
|
struct iio_dev *indio_dev;
|
|
|
|
indio_dev = devm_iio_device_alloc(&pdev->dev, 0);
|
|
if (!indio_dev)
|
|
return -ENOMEM;
|
|
|
|
iio_device_set_drvdata(indio_dev, iqs62x);
|
|
|
|
indio_dev->modes = INDIO_DIRECT_MODE;
|
|
indio_dev->channels = iqs620_temp_channels;
|
|
indio_dev->num_channels = ARRAY_SIZE(iqs620_temp_channels);
|
|
indio_dev->name = iqs62x->dev_desc->dev_name;
|
|
indio_dev->info = &iqs620_temp_info;
|
|
|
|
return devm_iio_device_register(&pdev->dev, indio_dev);
|
|
}
|
|
|
|
static struct platform_driver iqs620_temp_platform_driver = {
|
|
.driver = {
|
|
.name = "iqs620at-temp",
|
|
},
|
|
.probe = iqs620_temp_probe,
|
|
};
|
|
module_platform_driver(iqs620_temp_platform_driver);
|
|
|
|
MODULE_AUTHOR("Jeff LaBundy <jeff@labundy.com>");
|
|
MODULE_DESCRIPTION("Azoteq IQS620AT Temperature Sensor");
|
|
MODULE_LICENSE("GPL");
|
|
MODULE_ALIAS("platform:iqs620at-temp");
|