mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-15 15:15:47 +00:00
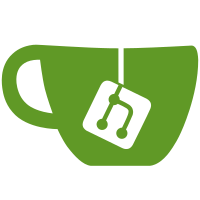
On x86_64, the double fault exception stack is located immediately after
the interrupt stack in memory. This causes confusion in the unwinder
when it tries to unwind through an empty interrupt stack, where the
stack pointer points to the address bordering the two stacks. The
unwinder incorrectly thinks it's running on the double fault stack.
Fix this kind of stack border confusion by never considering the
beginning address of an exception or interrupt stack to be part of the
stack.
Signed-off-by: Josh Poimboeuf <jpoimboe@redhat.com>
Cc: Andy Lutomirski <luto@kernel.org>
Cc: Borislav Petkov <bp@alien8.de>
Cc: Brian Gerst <brgerst@gmail.com>
Cc: Denys Vlasenko <dvlasenk@redhat.com>
Cc: H. Peter Anvin <hpa@zytor.com>
Cc: Jiri Slaby <jslaby@suse.cz>
Cc: Linus Torvalds <torvalds@linux-foundation.org>
Cc: Mike Galbraith <efault@gmx.de>
Cc: Peter Zijlstra <peterz@infradead.org>
Cc: Thomas Gleixner <tglx@linutronix.de>
Cc: live-patching@vger.kernel.org
Fixes: 5fe599e02e
("x86/dumpstack: Add support for unwinding empty IRQ stacks")
Link: http://lkml.kernel.org/r/bcc142160a5104de5c354c21c394c93a0173943f.1499786555.git.jpoimboe@redhat.com
Signed-off-by: Ingo Molnar <mingo@kernel.org>
164 lines
3.6 KiB
C
164 lines
3.6 KiB
C
/*
|
|
* Copyright (C) 1991, 1992 Linus Torvalds
|
|
* Copyright (C) 2000, 2001, 2002 Andi Kleen, SuSE Labs
|
|
*/
|
|
#include <linux/sched/debug.h>
|
|
#include <linux/kallsyms.h>
|
|
#include <linux/kprobes.h>
|
|
#include <linux/uaccess.h>
|
|
#include <linux/hardirq.h>
|
|
#include <linux/kdebug.h>
|
|
#include <linux/export.h>
|
|
#include <linux/ptrace.h>
|
|
#include <linux/kexec.h>
|
|
#include <linux/sysfs.h>
|
|
#include <linux/bug.h>
|
|
#include <linux/nmi.h>
|
|
|
|
#include <asm/stacktrace.h>
|
|
|
|
const char *stack_type_name(enum stack_type type)
|
|
{
|
|
if (type == STACK_TYPE_IRQ)
|
|
return "IRQ";
|
|
|
|
if (type == STACK_TYPE_SOFTIRQ)
|
|
return "SOFTIRQ";
|
|
|
|
return NULL;
|
|
}
|
|
|
|
static bool in_hardirq_stack(unsigned long *stack, struct stack_info *info)
|
|
{
|
|
unsigned long *begin = (unsigned long *)this_cpu_read(hardirq_stack);
|
|
unsigned long *end = begin + (THREAD_SIZE / sizeof(long));
|
|
|
|
/*
|
|
* This is a software stack, so 'end' can be a valid stack pointer.
|
|
* It just means the stack is empty.
|
|
*/
|
|
if (stack <= begin || stack > end)
|
|
return false;
|
|
|
|
info->type = STACK_TYPE_IRQ;
|
|
info->begin = begin;
|
|
info->end = end;
|
|
|
|
/*
|
|
* See irq_32.c -- the next stack pointer is stored at the beginning of
|
|
* the stack.
|
|
*/
|
|
info->next_sp = (unsigned long *)*begin;
|
|
|
|
return true;
|
|
}
|
|
|
|
static bool in_softirq_stack(unsigned long *stack, struct stack_info *info)
|
|
{
|
|
unsigned long *begin = (unsigned long *)this_cpu_read(softirq_stack);
|
|
unsigned long *end = begin + (THREAD_SIZE / sizeof(long));
|
|
|
|
/*
|
|
* This is a software stack, so 'end' can be a valid stack pointer.
|
|
* It just means the stack is empty.
|
|
*/
|
|
if (stack <= begin || stack > end)
|
|
return false;
|
|
|
|
info->type = STACK_TYPE_SOFTIRQ;
|
|
info->begin = begin;
|
|
info->end = end;
|
|
|
|
/*
|
|
* The next stack pointer is stored at the beginning of the stack.
|
|
* See irq_32.c.
|
|
*/
|
|
info->next_sp = (unsigned long *)*begin;
|
|
|
|
return true;
|
|
}
|
|
|
|
int get_stack_info(unsigned long *stack, struct task_struct *task,
|
|
struct stack_info *info, unsigned long *visit_mask)
|
|
{
|
|
if (!stack)
|
|
goto unknown;
|
|
|
|
task = task ? : current;
|
|
|
|
if (in_task_stack(stack, task, info))
|
|
goto recursion_check;
|
|
|
|
if (task != current)
|
|
goto unknown;
|
|
|
|
if (in_hardirq_stack(stack, info))
|
|
goto recursion_check;
|
|
|
|
if (in_softirq_stack(stack, info))
|
|
goto recursion_check;
|
|
|
|
goto unknown;
|
|
|
|
recursion_check:
|
|
/*
|
|
* Make sure we don't iterate through any given stack more than once.
|
|
* If it comes up a second time then there's something wrong going on:
|
|
* just break out and report an unknown stack type.
|
|
*/
|
|
if (visit_mask) {
|
|
if (*visit_mask & (1UL << info->type)) {
|
|
printk_deferred_once(KERN_WARNING "WARNING: stack recursion on stack type %d\n", info->type);
|
|
goto unknown;
|
|
}
|
|
*visit_mask |= 1UL << info->type;
|
|
}
|
|
|
|
return 0;
|
|
|
|
unknown:
|
|
info->type = STACK_TYPE_UNKNOWN;
|
|
return -EINVAL;
|
|
}
|
|
|
|
void show_regs(struct pt_regs *regs)
|
|
{
|
|
int i;
|
|
|
|
show_regs_print_info(KERN_EMERG);
|
|
__show_regs(regs, !user_mode(regs));
|
|
|
|
/*
|
|
* When in-kernel, we also print out the stack and code at the
|
|
* time of the fault..
|
|
*/
|
|
if (!user_mode(regs)) {
|
|
unsigned int code_prologue = code_bytes * 43 / 64;
|
|
unsigned int code_len = code_bytes;
|
|
unsigned char c;
|
|
u8 *ip;
|
|
|
|
show_trace_log_lvl(current, regs, NULL, KERN_EMERG);
|
|
|
|
pr_emerg("Code:");
|
|
|
|
ip = (u8 *)regs->ip - code_prologue;
|
|
if (ip < (u8 *)PAGE_OFFSET || probe_kernel_address(ip, c)) {
|
|
/* try starting at IP */
|
|
ip = (u8 *)regs->ip;
|
|
code_len = code_len - code_prologue + 1;
|
|
}
|
|
for (i = 0; i < code_len; i++, ip++) {
|
|
if (ip < (u8 *)PAGE_OFFSET ||
|
|
probe_kernel_address(ip, c)) {
|
|
pr_cont(" Bad EIP value.");
|
|
break;
|
|
}
|
|
if (ip == (u8 *)regs->ip)
|
|
pr_cont(" <%02x>", c);
|
|
else
|
|
pr_cont(" %02x", c);
|
|
}
|
|
}
|
|
pr_cont("\n");
|
|
}
|