mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-31 16:38:12 +00:00
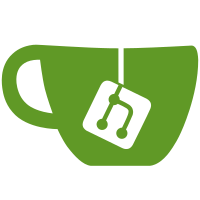
It is better to use get_task_comm() instead of the open coded string copy as we do in other places. struct elf_prpsinfo is used to dump the task information in userspace coredump or kernel vmcore. Below is the verification of vmcore, crash> ps PID PPID CPU TASK ST %MEM VSZ RSS COMM 0 0 0 ffffffff9d21a940 RU 0.0 0 0 [swapper/0] > 0 0 1 ffffa09e40f85e80 RU 0.0 0 0 [swapper/1] > 0 0 2 ffffa09e40f81f80 RU 0.0 0 0 [swapper/2] > 0 0 3 ffffa09e40f83f00 RU 0.0 0 0 [swapper/3] > 0 0 4 ffffa09e40f80000 RU 0.0 0 0 [swapper/4] > 0 0 5 ffffa09e40f89f80 RU 0.0 0 0 [swapper/5] 0 0 6 ffffa09e40f8bf00 RU 0.0 0 0 [swapper/6] > 0 0 7 ffffa09e40f88000 RU 0.0 0 0 [swapper/7] > 0 0 8 ffffa09e40f8de80 RU 0.0 0 0 [swapper/8] > 0 0 9 ffffa09e40f95e80 RU 0.0 0 0 [swapper/9] > 0 0 10 ffffa09e40f91f80 RU 0.0 0 0 [swapper/10] > 0 0 11 ffffa09e40f93f00 RU 0.0 0 0 [swapper/11] > 0 0 12 ffffa09e40f90000 RU 0.0 0 0 [swapper/12] > 0 0 13 ffffa09e40f9bf00 RU 0.0 0 0 [swapper/13] > 0 0 14 ffffa09e40f98000 RU 0.0 0 0 [swapper/14] > 0 0 15 ffffa09e40f9de80 RU 0.0 0 0 [swapper/15] It works well as expected. Some comments are added to explain why we use the hard-coded 16. Link: https://lkml.kernel.org/r/20211120112738.45980-5-laoar.shao@gmail.com Suggested-by: Kees Cook <keescook@chromium.org> Signed-off-by: Yafang Shao <laoar.shao@gmail.com> Reviewed-by: David Hildenbrand <david@redhat.com> Cc: Mathieu Desnoyers <mathieu.desnoyers@efficios.com> Cc: Arnaldo Carvalho de Melo <arnaldo.melo@gmail.com> Cc: Andrii Nakryiko <andrii.nakryiko@gmail.com> Cc: Michal Miroslaw <mirq-linux@rere.qmqm.pl> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Steven Rostedt <rostedt@goodmis.org> Cc: Matthew Wilcox <willy@infradead.org> Cc: David Hildenbrand <david@redhat.com> Cc: Al Viro <viro@zeniv.linux.org.uk> Cc: Kees Cook <keescook@chromium.org> Cc: Petr Mladek <pmladek@suse.com> Cc: Alexei Starovoitov <alexei.starovoitov@gmail.com> Cc: Andrii Nakryiko <andrii@kernel.org> Cc: Dennis Dalessandro <dennis.dalessandro@cornelisnetworks.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
66 lines
1.4 KiB
C
66 lines
1.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _LINUX_ELFCORE_COMPAT_H
|
|
#define _LINUX_ELFCORE_COMPAT_H
|
|
|
|
#include <linux/elf.h>
|
|
#include <linux/elfcore.h>
|
|
#include <linux/compat.h>
|
|
|
|
/*
|
|
* Make sure these layouts match the linux/elfcore.h native definitions.
|
|
*/
|
|
|
|
struct compat_elf_siginfo
|
|
{
|
|
compat_int_t si_signo;
|
|
compat_int_t si_code;
|
|
compat_int_t si_errno;
|
|
};
|
|
|
|
struct compat_elf_prstatus_common
|
|
{
|
|
struct compat_elf_siginfo pr_info;
|
|
short pr_cursig;
|
|
compat_ulong_t pr_sigpend;
|
|
compat_ulong_t pr_sighold;
|
|
compat_pid_t pr_pid;
|
|
compat_pid_t pr_ppid;
|
|
compat_pid_t pr_pgrp;
|
|
compat_pid_t pr_sid;
|
|
struct old_timeval32 pr_utime;
|
|
struct old_timeval32 pr_stime;
|
|
struct old_timeval32 pr_cutime;
|
|
struct old_timeval32 pr_cstime;
|
|
};
|
|
|
|
struct compat_elf_prpsinfo
|
|
{
|
|
char pr_state;
|
|
char pr_sname;
|
|
char pr_zomb;
|
|
char pr_nice;
|
|
compat_ulong_t pr_flag;
|
|
__compat_uid_t pr_uid;
|
|
__compat_gid_t pr_gid;
|
|
compat_pid_t pr_pid, pr_ppid, pr_pgrp, pr_sid;
|
|
/*
|
|
* The hard-coded 16 is derived from TASK_COMM_LEN, but it can't be
|
|
* changed as it is exposed to userspace. We'd better make it hard-coded
|
|
* here.
|
|
*/
|
|
char pr_fname[16];
|
|
char pr_psargs[ELF_PRARGSZ];
|
|
};
|
|
|
|
#ifdef CONFIG_ARCH_HAS_ELFCORE_COMPAT
|
|
#include <asm/elfcore-compat.h>
|
|
#endif
|
|
|
|
struct compat_elf_prstatus
|
|
{
|
|
struct compat_elf_prstatus_common common;
|
|
compat_elf_gregset_t pr_reg;
|
|
compat_int_t pr_fpvalid;
|
|
};
|
|
|
|
#endif /* _LINUX_ELFCORE_COMPAT_H */
|