mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
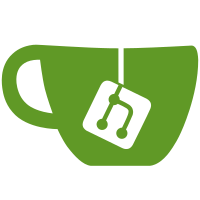
There are no users of MEM_TYPE_ZERO_COPY. Remove all corresponding code, including the "handle" member of struct xdp_buff. rfc->v1: Fixed spelling in commit message. (Björn) Signed-off-by: Björn Töpel <bjorn.topel@intel.com> Signed-off-by: Alexei Starovoitov <ast@kernel.org> Link: https://lore.kernel.org/bpf/20200520192103.355233-13-bjorn.topel@gmail.com
57 lines
1.1 KiB
C
57 lines
1.1 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/* XDP user-space ring structure
|
|
* Copyright(c) 2018 Intel Corporation.
|
|
*/
|
|
|
|
#include <linux/log2.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/overflow.h>
|
|
#include <net/xdp_sock_drv.h>
|
|
|
|
#include "xsk_queue.h"
|
|
|
|
static size_t xskq_get_ring_size(struct xsk_queue *q, bool umem_queue)
|
|
{
|
|
struct xdp_umem_ring *umem_ring;
|
|
struct xdp_rxtx_ring *rxtx_ring;
|
|
|
|
if (umem_queue)
|
|
return struct_size(umem_ring, desc, q->nentries);
|
|
return struct_size(rxtx_ring, desc, q->nentries);
|
|
}
|
|
|
|
struct xsk_queue *xskq_create(u32 nentries, bool umem_queue)
|
|
{
|
|
struct xsk_queue *q;
|
|
gfp_t gfp_flags;
|
|
size_t size;
|
|
|
|
q = kzalloc(sizeof(*q), GFP_KERNEL);
|
|
if (!q)
|
|
return NULL;
|
|
|
|
q->nentries = nentries;
|
|
q->ring_mask = nentries - 1;
|
|
|
|
gfp_flags = GFP_KERNEL | __GFP_ZERO | __GFP_NOWARN |
|
|
__GFP_COMP | __GFP_NORETRY;
|
|
size = xskq_get_ring_size(q, umem_queue);
|
|
|
|
q->ring = (struct xdp_ring *)__get_free_pages(gfp_flags,
|
|
get_order(size));
|
|
if (!q->ring) {
|
|
kfree(q);
|
|
return NULL;
|
|
}
|
|
|
|
return q;
|
|
}
|
|
|
|
void xskq_destroy(struct xsk_queue *q)
|
|
{
|
|
if (!q)
|
|
return;
|
|
|
|
page_frag_free(q->ring);
|
|
kfree(q);
|
|
}
|