mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-22 08:49:45 +00:00
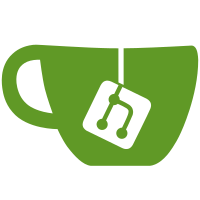
kernel.h is being used as a dump for all kinds of stuff for a long time. Here is the attempt to start cleaning it up by splitting out mathematical helpers. At the same time convert users in header and lib folder to use new header. Though for time being include new header back to kernel.h to avoid twisted indirected includes for existing users. [sfr@canb.auug.org.au: fix powerpc build] Link: https://lkml.kernel.org/r/20201029150809.13059608@canb.auug.org.au Link: https://lkml.kernel.org/r/20201028173212.41768-1-andriy.shevchenko@linux.intel.com Signed-off-by: Andy Shevchenko <andriy.shevchenko@linux.intel.com> Cc: "Paul E. McKenney" <paulmck@kernel.org> Cc: Trond Myklebust <trond.myklebust@hammerspace.com> Cc: Jeff Layton <jlayton@kernel.org> Cc: Rasmus Villemoes <linux@rasmusvillemoes.dk> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
194 lines
4.5 KiB
C
194 lines
4.5 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/* bit search implementation
|
|
*
|
|
* Copyright (C) 2004 Red Hat, Inc. All Rights Reserved.
|
|
* Written by David Howells (dhowells@redhat.com)
|
|
*
|
|
* Copyright (C) 2008 IBM Corporation
|
|
* 'find_last_bit' is written by Rusty Russell <rusty@rustcorp.com.au>
|
|
* (Inspired by David Howell's find_next_bit implementation)
|
|
*
|
|
* Rewritten by Yury Norov <yury.norov@gmail.com> to decrease
|
|
* size and improve performance, 2015.
|
|
*/
|
|
|
|
#include <linux/bitops.h>
|
|
#include <linux/bitmap.h>
|
|
#include <linux/export.h>
|
|
#include <linux/math.h>
|
|
#include <linux/minmax.h>
|
|
#include <linux/swab.h>
|
|
|
|
#if !defined(find_next_bit) || !defined(find_next_zero_bit) || \
|
|
!defined(find_next_bit_le) || !defined(find_next_zero_bit_le) || \
|
|
!defined(find_next_and_bit)
|
|
/*
|
|
* This is a common helper function for find_next_bit, find_next_zero_bit, and
|
|
* find_next_and_bit. The differences are:
|
|
* - The "invert" argument, which is XORed with each fetched word before
|
|
* searching it for one bits.
|
|
* - The optional "addr2", which is anded with "addr1" if present.
|
|
*/
|
|
static unsigned long _find_next_bit(const unsigned long *addr1,
|
|
const unsigned long *addr2, unsigned long nbits,
|
|
unsigned long start, unsigned long invert, unsigned long le)
|
|
{
|
|
unsigned long tmp, mask;
|
|
|
|
if (unlikely(start >= nbits))
|
|
return nbits;
|
|
|
|
tmp = addr1[start / BITS_PER_LONG];
|
|
if (addr2)
|
|
tmp &= addr2[start / BITS_PER_LONG];
|
|
tmp ^= invert;
|
|
|
|
/* Handle 1st word. */
|
|
mask = BITMAP_FIRST_WORD_MASK(start);
|
|
if (le)
|
|
mask = swab(mask);
|
|
|
|
tmp &= mask;
|
|
|
|
start = round_down(start, BITS_PER_LONG);
|
|
|
|
while (!tmp) {
|
|
start += BITS_PER_LONG;
|
|
if (start >= nbits)
|
|
return nbits;
|
|
|
|
tmp = addr1[start / BITS_PER_LONG];
|
|
if (addr2)
|
|
tmp &= addr2[start / BITS_PER_LONG];
|
|
tmp ^= invert;
|
|
}
|
|
|
|
if (le)
|
|
tmp = swab(tmp);
|
|
|
|
return min(start + __ffs(tmp), nbits);
|
|
}
|
|
#endif
|
|
|
|
#ifndef find_next_bit
|
|
/*
|
|
* Find the next set bit in a memory region.
|
|
*/
|
|
unsigned long find_next_bit(const unsigned long *addr, unsigned long size,
|
|
unsigned long offset)
|
|
{
|
|
return _find_next_bit(addr, NULL, size, offset, 0UL, 0);
|
|
}
|
|
EXPORT_SYMBOL(find_next_bit);
|
|
#endif
|
|
|
|
#ifndef find_next_zero_bit
|
|
unsigned long find_next_zero_bit(const unsigned long *addr, unsigned long size,
|
|
unsigned long offset)
|
|
{
|
|
return _find_next_bit(addr, NULL, size, offset, ~0UL, 0);
|
|
}
|
|
EXPORT_SYMBOL(find_next_zero_bit);
|
|
#endif
|
|
|
|
#if !defined(find_next_and_bit)
|
|
unsigned long find_next_and_bit(const unsigned long *addr1,
|
|
const unsigned long *addr2, unsigned long size,
|
|
unsigned long offset)
|
|
{
|
|
return _find_next_bit(addr1, addr2, size, offset, 0UL, 0);
|
|
}
|
|
EXPORT_SYMBOL(find_next_and_bit);
|
|
#endif
|
|
|
|
#ifndef find_first_bit
|
|
/*
|
|
* Find the first set bit in a memory region.
|
|
*/
|
|
unsigned long find_first_bit(const unsigned long *addr, unsigned long size)
|
|
{
|
|
unsigned long idx;
|
|
|
|
for (idx = 0; idx * BITS_PER_LONG < size; idx++) {
|
|
if (addr[idx])
|
|
return min(idx * BITS_PER_LONG + __ffs(addr[idx]), size);
|
|
}
|
|
|
|
return size;
|
|
}
|
|
EXPORT_SYMBOL(find_first_bit);
|
|
#endif
|
|
|
|
#ifndef find_first_zero_bit
|
|
/*
|
|
* Find the first cleared bit in a memory region.
|
|
*/
|
|
unsigned long find_first_zero_bit(const unsigned long *addr, unsigned long size)
|
|
{
|
|
unsigned long idx;
|
|
|
|
for (idx = 0; idx * BITS_PER_LONG < size; idx++) {
|
|
if (addr[idx] != ~0UL)
|
|
return min(idx * BITS_PER_LONG + ffz(addr[idx]), size);
|
|
}
|
|
|
|
return size;
|
|
}
|
|
EXPORT_SYMBOL(find_first_zero_bit);
|
|
#endif
|
|
|
|
#ifndef find_last_bit
|
|
unsigned long find_last_bit(const unsigned long *addr, unsigned long size)
|
|
{
|
|
if (size) {
|
|
unsigned long val = BITMAP_LAST_WORD_MASK(size);
|
|
unsigned long idx = (size-1) / BITS_PER_LONG;
|
|
|
|
do {
|
|
val &= addr[idx];
|
|
if (val)
|
|
return idx * BITS_PER_LONG + __fls(val);
|
|
|
|
val = ~0ul;
|
|
} while (idx--);
|
|
}
|
|
return size;
|
|
}
|
|
EXPORT_SYMBOL(find_last_bit);
|
|
#endif
|
|
|
|
#ifdef __BIG_ENDIAN
|
|
|
|
#ifndef find_next_zero_bit_le
|
|
unsigned long find_next_zero_bit_le(const void *addr, unsigned
|
|
long size, unsigned long offset)
|
|
{
|
|
return _find_next_bit(addr, NULL, size, offset, ~0UL, 1);
|
|
}
|
|
EXPORT_SYMBOL(find_next_zero_bit_le);
|
|
#endif
|
|
|
|
#ifndef find_next_bit_le
|
|
unsigned long find_next_bit_le(const void *addr, unsigned
|
|
long size, unsigned long offset)
|
|
{
|
|
return _find_next_bit(addr, NULL, size, offset, 0UL, 1);
|
|
}
|
|
EXPORT_SYMBOL(find_next_bit_le);
|
|
#endif
|
|
|
|
#endif /* __BIG_ENDIAN */
|
|
|
|
unsigned long find_next_clump8(unsigned long *clump, const unsigned long *addr,
|
|
unsigned long size, unsigned long offset)
|
|
{
|
|
offset = find_next_bit(addr, size, offset);
|
|
if (offset == size)
|
|
return size;
|
|
|
|
offset = round_down(offset, 8);
|
|
*clump = bitmap_get_value8(addr, offset);
|
|
|
|
return offset;
|
|
}
|
|
EXPORT_SYMBOL(find_next_clump8);
|