mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-21 16:29:59 +00:00
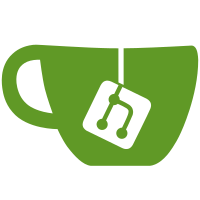
Check that it is not needed and remove, fixing up some fallout for places where it was only serving to get something else. Cc: Adrian Hunter <adrian.hunter@intel.com> Cc: Jiri Olsa <jolsa@kernel.org> Cc: Namhyung Kim <namhyung@kernel.org> Link: https://lkml.kernel.org/n/tip-9h6dg6lsqe2usyqjh5rrues4@git.kernel.org Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
83 lines
1.3 KiB
C
83 lines
1.3 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
|
|
#include "helpline.h"
|
|
#include "ui.h"
|
|
|
|
char ui_helpline__current[512];
|
|
|
|
static void nop_helpline__pop(void)
|
|
{
|
|
}
|
|
|
|
static void nop_helpline__push(const char *msg __maybe_unused)
|
|
{
|
|
}
|
|
|
|
static int nop_helpline__show(const char *fmt __maybe_unused,
|
|
va_list ap __maybe_unused)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static struct ui_helpline default_helpline_fns = {
|
|
.pop = nop_helpline__pop,
|
|
.push = nop_helpline__push,
|
|
.show = nop_helpline__show,
|
|
};
|
|
|
|
struct ui_helpline *helpline_fns = &default_helpline_fns;
|
|
|
|
void ui_helpline__pop(void)
|
|
{
|
|
helpline_fns->pop();
|
|
}
|
|
|
|
void ui_helpline__push(const char *msg)
|
|
{
|
|
helpline_fns->push(msg);
|
|
}
|
|
|
|
void ui_helpline__vpush(const char *fmt, va_list ap)
|
|
{
|
|
char *s;
|
|
|
|
if (vasprintf(&s, fmt, ap) < 0)
|
|
vfprintf(stderr, fmt, ap);
|
|
else {
|
|
ui_helpline__push(s);
|
|
free(s);
|
|
}
|
|
}
|
|
|
|
void ui_helpline__fpush(const char *fmt, ...)
|
|
{
|
|
va_list ap;
|
|
|
|
va_start(ap, fmt);
|
|
ui_helpline__vpush(fmt, ap);
|
|
va_end(ap);
|
|
}
|
|
|
|
void ui_helpline__puts(const char *msg)
|
|
{
|
|
ui_helpline__pop();
|
|
ui_helpline__push(msg);
|
|
}
|
|
|
|
int ui_helpline__vshow(const char *fmt, va_list ap)
|
|
{
|
|
return helpline_fns->show(fmt, ap);
|
|
}
|
|
|
|
void ui_helpline__printf(const char *fmt, ...)
|
|
{
|
|
va_list ap;
|
|
|
|
ui_helpline__pop();
|
|
va_start(ap, fmt);
|
|
ui_helpline__vpush(fmt, ap);
|
|
va_end(ap);
|
|
}
|