mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-21 16:29:59 +00:00
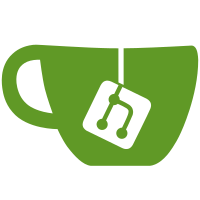
At the cost of an extra pointer, we can avoid the O(logN) cost of finding the first element in the tree (smallest node), which is something required for any of the strlist or intlist traversals (XXX_for_each_entry()). There are a number of users in perf of these (particularly strlists), including probes, and buildid. Signed-off-by: Davidlohr Bueso <dbueso@suse.de> Tested-by: Arnaldo Carvalho de Melo <acme@redhat.com> Cc: Jiri Olsa <jolsa@kernel.org> Cc: Namhyung Kim <namhyung@kernel.org> Link: http://lkml.kernel.org/r/20181206191819.30182-5-dave@stgolabs.net Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
50 lines
1.4 KiB
C
50 lines
1.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef __PERF_RBLIST_H
|
|
#define __PERF_RBLIST_H
|
|
|
|
#include <linux/rbtree.h>
|
|
#include <stdbool.h>
|
|
|
|
/*
|
|
* create node structs of the form:
|
|
* struct my_node {
|
|
* struct rb_node rb_node;
|
|
* ... my data ...
|
|
* };
|
|
*
|
|
* create list structs of the form:
|
|
* struct mylist {
|
|
* struct rblist rblist;
|
|
* ... my data ...
|
|
* };
|
|
*/
|
|
|
|
struct rblist {
|
|
struct rb_root_cached entries;
|
|
unsigned int nr_entries;
|
|
|
|
int (*node_cmp)(struct rb_node *rbn, const void *entry);
|
|
struct rb_node *(*node_new)(struct rblist *rlist, const void *new_entry);
|
|
void (*node_delete)(struct rblist *rblist, struct rb_node *rb_node);
|
|
};
|
|
|
|
void rblist__init(struct rblist *rblist);
|
|
void rblist__exit(struct rblist *rblist);
|
|
void rblist__delete(struct rblist *rblist);
|
|
int rblist__add_node(struct rblist *rblist, const void *new_entry);
|
|
void rblist__remove_node(struct rblist *rblist, struct rb_node *rb_node);
|
|
struct rb_node *rblist__find(struct rblist *rblist, const void *entry);
|
|
struct rb_node *rblist__findnew(struct rblist *rblist, const void *entry);
|
|
struct rb_node *rblist__entry(const struct rblist *rblist, unsigned int idx);
|
|
|
|
static inline bool rblist__empty(const struct rblist *rblist)
|
|
{
|
|
return rblist->nr_entries == 0;
|
|
}
|
|
|
|
static inline unsigned int rblist__nr_entries(const struct rblist *rblist)
|
|
{
|
|
return rblist->nr_entries;
|
|
}
|
|
|
|
#endif /* __PERF_RBLIST_H */
|