mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 08:58:07 +00:00
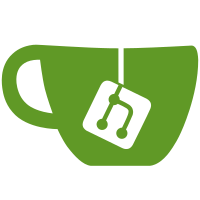
After commit63d0434
("KVM: x86: move kvm_create_vcpu_debugfs after last failure point") we are creating the pre-vCPU debugfs files after the creation of the vCPU file descriptor. This makes it possible for userspace to reach kvm_vcpu_release before kvm_create_vcpu_debugfs has finished. The vcpu->debugfs_dentry then does not have any associated inode anymore, and this causes a NULL-pointer dereference in debugfs_create_file. The solution is simply to avoid removing the files; they are cleaned up when the VM file descriptor is closed (and that must be after KVM_CREATE_VCPU returns). We can stop storing the dentry in struct kvm_vcpu too, because it is not needed anywhere after kvm_create_vcpu_debugfs returns. Reported-by: syzbot+705f4401d5a93a59b87d@syzkaller.appspotmail.com Fixes:63d0434837
("KVM: x86: move kvm_create_vcpu_debugfs after last failure point") Signed-off-by: Paolo Bonzini <pbonzini@redhat.com>
64 lines
1.8 KiB
C
64 lines
1.8 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* Kernel-based Virtual Machine driver for Linux
|
|
*
|
|
* Copyright 2016 Red Hat, Inc. and/or its affiliates.
|
|
*/
|
|
#include <linux/kvm_host.h>
|
|
#include <linux/debugfs.h>
|
|
#include "lapic.h"
|
|
|
|
static int vcpu_get_timer_advance_ns(void *data, u64 *val)
|
|
{
|
|
struct kvm_vcpu *vcpu = (struct kvm_vcpu *) data;
|
|
*val = vcpu->arch.apic->lapic_timer.timer_advance_ns;
|
|
return 0;
|
|
}
|
|
|
|
DEFINE_SIMPLE_ATTRIBUTE(vcpu_timer_advance_ns_fops, vcpu_get_timer_advance_ns, NULL, "%llu\n");
|
|
|
|
static int vcpu_get_tsc_offset(void *data, u64 *val)
|
|
{
|
|
struct kvm_vcpu *vcpu = (struct kvm_vcpu *) data;
|
|
*val = vcpu->arch.tsc_offset;
|
|
return 0;
|
|
}
|
|
|
|
DEFINE_SIMPLE_ATTRIBUTE(vcpu_tsc_offset_fops, vcpu_get_tsc_offset, NULL, "%lld\n");
|
|
|
|
static int vcpu_get_tsc_scaling_ratio(void *data, u64 *val)
|
|
{
|
|
struct kvm_vcpu *vcpu = (struct kvm_vcpu *) data;
|
|
*val = vcpu->arch.tsc_scaling_ratio;
|
|
return 0;
|
|
}
|
|
|
|
DEFINE_SIMPLE_ATTRIBUTE(vcpu_tsc_scaling_fops, vcpu_get_tsc_scaling_ratio, NULL, "%llu\n");
|
|
|
|
static int vcpu_get_tsc_scaling_frac_bits(void *data, u64 *val)
|
|
{
|
|
*val = kvm_tsc_scaling_ratio_frac_bits;
|
|
return 0;
|
|
}
|
|
|
|
DEFINE_SIMPLE_ATTRIBUTE(vcpu_tsc_scaling_frac_fops, vcpu_get_tsc_scaling_frac_bits, NULL, "%llu\n");
|
|
|
|
void kvm_arch_create_vcpu_debugfs(struct kvm_vcpu *vcpu, struct dentry *debugfs_dentry)
|
|
{
|
|
debugfs_create_file("tsc-offset", 0444, debugfs_dentry, vcpu,
|
|
&vcpu_tsc_offset_fops);
|
|
|
|
if (lapic_in_kernel(vcpu))
|
|
debugfs_create_file("lapic_timer_advance_ns", 0444,
|
|
debugfs_dentry, vcpu,
|
|
&vcpu_timer_advance_ns_fops);
|
|
|
|
if (kvm_has_tsc_control) {
|
|
debugfs_create_file("tsc-scaling-ratio", 0444,
|
|
debugfs_dentry, vcpu,
|
|
&vcpu_tsc_scaling_fops);
|
|
debugfs_create_file("tsc-scaling-ratio-frac-bits", 0444,
|
|
debugfs_dentry, vcpu,
|
|
&vcpu_tsc_scaling_frac_fops);
|
|
}
|
|
}
|