mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-25 11:55:37 +00:00
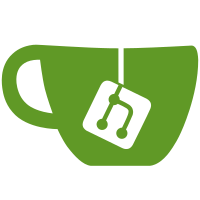
This `clang-analyzer` check flags the use of memset(), suggesting a more secure version of the API, such as memset_s(), which does not exist in the kernel: warning: Call to function 'memset' is insecure as it does not provide security checks introduced in the C11 standard. Replace with analogous functions that support length arguments or provides boundary checks such as 'memset_s' in case of C11 [clang-analyzer-security.insecureAPI.DeprecatedOrUnsafeBufferHandling] Signed-off-by: Guru Das Srinagesh <quic_gurus@quicinc.com> Reviewed-by: Nick Desaulniers <ndesaulniers@google.com> Signed-off-by: Masahiro Yamada <masahiroy@kernel.org>
75 lines
2 KiB
Python
Executable file
75 lines
2 KiB
Python
Executable file
#!/usr/bin/env python3
|
|
# SPDX-License-Identifier: GPL-2.0
|
|
#
|
|
# Copyright (C) Google LLC, 2020
|
|
#
|
|
# Author: Nathan Huckleberry <nhuck@google.com>
|
|
#
|
|
"""A helper routine run clang-tidy and the clang static-analyzer on
|
|
compile_commands.json.
|
|
"""
|
|
|
|
import argparse
|
|
import json
|
|
import multiprocessing
|
|
import os
|
|
import subprocess
|
|
import sys
|
|
|
|
|
|
def parse_arguments():
|
|
"""Set up and parses command-line arguments.
|
|
Returns:
|
|
args: Dict of parsed args
|
|
Has keys: [path, type]
|
|
"""
|
|
usage = """Run clang-tidy or the clang static-analyzer on a
|
|
compilation database."""
|
|
parser = argparse.ArgumentParser(description=usage)
|
|
|
|
type_help = "Type of analysis to be performed"
|
|
parser.add_argument("type",
|
|
choices=["clang-tidy", "clang-analyzer"],
|
|
help=type_help)
|
|
path_help = "Path to the compilation database to parse"
|
|
parser.add_argument("path", type=str, help=path_help)
|
|
|
|
return parser.parse_args()
|
|
|
|
|
|
def init(l, a):
|
|
global lock
|
|
global args
|
|
lock = l
|
|
args = a
|
|
|
|
|
|
def run_analysis(entry):
|
|
# Disable all checks, then re-enable the ones we want
|
|
checks = "-checks=-*,"
|
|
if args.type == "clang-tidy":
|
|
checks += "linuxkernel-*"
|
|
else:
|
|
checks += "clang-analyzer-*"
|
|
checks += ",-clang-analyzer-security.insecureAPI.DeprecatedOrUnsafeBufferHandling"
|
|
p = subprocess.run(["clang-tidy", "-p", args.path, checks, entry["file"]],
|
|
stdout=subprocess.PIPE,
|
|
stderr=subprocess.STDOUT,
|
|
cwd=entry["directory"])
|
|
with lock:
|
|
sys.stderr.buffer.write(p.stdout)
|
|
|
|
|
|
def main():
|
|
args = parse_arguments()
|
|
|
|
lock = multiprocessing.Lock()
|
|
pool = multiprocessing.Pool(initializer=init, initargs=(lock, args))
|
|
# Read JSON data into the datastore variable
|
|
with open(args.path, "r") as f:
|
|
datastore = json.load(f)
|
|
pool.map(run_analysis, datastore)
|
|
|
|
|
|
if __name__ == "__main__":
|
|
main()
|