mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-23 19:11:51 +00:00
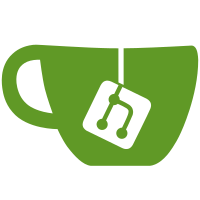
Most discovery/configuration of the nvdimm-subsystem is done via sysfs attributes. However, some nvdimm_bus instances, particularly the ACPI.NFIT bus, define a small set of messages that can be passed to the platform. For convenience we derive the initial libnvdimm-ioctl command formats directly from the NFIT DSM Interface Example formats. ND_CMD_SMART: media health and diagnostics ND_CMD_GET_CONFIG_SIZE: size of the label space ND_CMD_GET_CONFIG_DATA: read label space ND_CMD_SET_CONFIG_DATA: write label space ND_CMD_VENDOR: vendor-specific command passthrough ND_CMD_ARS_CAP: report address-range-scrubbing capabilities ND_CMD_ARS_START: initiate scrubbing ND_CMD_ARS_STATUS: report on scrubbing state ND_CMD_SMART_THRESHOLD: configure alarm thresholds for smart events If a platform later defines different commands than this set it is straightforward to extend support to those formats. Most of the commands target a specific dimm. However, the address-range-scrubbing commands target the bus. The 'commands' attribute in sysfs of an nvdimm_bus, or nvdimm, enumerate the supported commands for that object. Cc: <linux-acpi@vger.kernel.org> Cc: Robert Moore <robert.moore@intel.com> Cc: Rafael J. Wysocki <rafael.j.wysocki@intel.com> Reported-by: Nicholas Moulin <nicholas.w.moulin@linux.intel.com> Acked-by: Christoph Hellwig <hch@lst.de> Signed-off-by: Dan Williams <dan.j.williams@intel.com>
100 lines
2.4 KiB
C
100 lines
2.4 KiB
C
/*
|
|
* NVDIMM Firmware Interface Table - NFIT
|
|
*
|
|
* Copyright(c) 2013-2015 Intel Corporation. All rights reserved.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of version 2 of the GNU General Public License as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*/
|
|
#ifndef __NFIT_H__
|
|
#define __NFIT_H__
|
|
#include <linux/libnvdimm.h>
|
|
#include <linux/types.h>
|
|
#include <linux/uuid.h>
|
|
#include <linux/acpi.h>
|
|
#include <acpi/acuuid.h>
|
|
|
|
#define UUID_NFIT_BUS "2f10e7a4-9e91-11e4-89d3-123b93f75cba"
|
|
#define UUID_NFIT_DIMM "4309ac30-0d11-11e4-9191-0800200c9a66"
|
|
|
|
enum nfit_uuids {
|
|
NFIT_SPA_VOLATILE,
|
|
NFIT_SPA_PM,
|
|
NFIT_SPA_DCR,
|
|
NFIT_SPA_BDW,
|
|
NFIT_SPA_VDISK,
|
|
NFIT_SPA_VCD,
|
|
NFIT_SPA_PDISK,
|
|
NFIT_SPA_PCD,
|
|
NFIT_DEV_BUS,
|
|
NFIT_DEV_DIMM,
|
|
NFIT_UUID_MAX,
|
|
};
|
|
|
|
struct nfit_spa {
|
|
struct acpi_nfit_system_address *spa;
|
|
struct list_head list;
|
|
};
|
|
|
|
struct nfit_dcr {
|
|
struct acpi_nfit_control_region *dcr;
|
|
struct list_head list;
|
|
};
|
|
|
|
struct nfit_bdw {
|
|
struct acpi_nfit_data_region *bdw;
|
|
struct list_head list;
|
|
};
|
|
|
|
struct nfit_memdev {
|
|
struct acpi_nfit_memory_map *memdev;
|
|
struct list_head list;
|
|
};
|
|
|
|
/* assembled tables for a given dimm/memory-device */
|
|
struct nfit_mem {
|
|
struct nvdimm *nvdimm;
|
|
struct acpi_nfit_memory_map *memdev_dcr;
|
|
struct acpi_nfit_memory_map *memdev_pmem;
|
|
struct acpi_nfit_control_region *dcr;
|
|
struct acpi_nfit_data_region *bdw;
|
|
struct acpi_nfit_system_address *spa_dcr;
|
|
struct acpi_nfit_system_address *spa_bdw;
|
|
struct list_head list;
|
|
struct acpi_device *adev;
|
|
unsigned long dsm_mask;
|
|
};
|
|
|
|
struct acpi_nfit_desc {
|
|
struct nvdimm_bus_descriptor nd_desc;
|
|
struct acpi_table_nfit *nfit;
|
|
struct list_head memdevs;
|
|
struct list_head dimms;
|
|
struct list_head spas;
|
|
struct list_head dcrs;
|
|
struct list_head bdws;
|
|
struct nvdimm_bus *nvdimm_bus;
|
|
struct device *dev;
|
|
unsigned long dimm_dsm_force_en;
|
|
};
|
|
|
|
static inline struct acpi_nfit_memory_map *__to_nfit_memdev(
|
|
struct nfit_mem *nfit_mem)
|
|
{
|
|
if (nfit_mem->memdev_dcr)
|
|
return nfit_mem->memdev_dcr;
|
|
return nfit_mem->memdev_pmem;
|
|
}
|
|
|
|
static inline struct acpi_nfit_desc *to_acpi_desc(
|
|
struct nvdimm_bus_descriptor *nd_desc)
|
|
{
|
|
return container_of(nd_desc, struct acpi_nfit_desc, nd_desc);
|
|
}
|
|
#endif /* __NFIT_H__ */
|