mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-20 01:20:54 +00:00
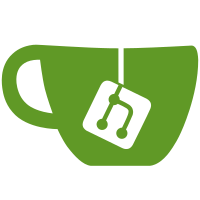
Add MicroLZMA support in order to maximize compression ratios for specific scenarios. For example, it's useful for low-end embedded boards and as a secondary algorithm in a file for specific access patterns. MicroLZMA is a new container format for raw LZMA1, which was created by Lasse Collin aiming to minimize old LZMA headers and get rid of unnecessary EOPM (end of payload marker) as well as to enable fixed-sized output compression, especially for 4KiB pclusters. Similar to LZ4, inplace I/O approach is used to minimize runtime memory footprint when dealing with I/O. Overlapped decompression is handled with 1) bounced buffer for data under processing or 2) extra short-lived pages from the on-stack pagepool which will be shared in the same read request (128KiB for example). Link: https://lore.kernel.org/r/20211010213145.17462-8-xiang@kernel.org Acked-by: Chao Yu <chao@kernel.org> Signed-off-by: Gao Xiang <hsiangkao@linux.alibaba.com>
97 lines
2.8 KiB
C
97 lines
2.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* Copyright (C) 2019 HUAWEI, Inc.
|
|
* https://www.huawei.com/
|
|
*/
|
|
#ifndef __EROFS_FS_COMPRESS_H
|
|
#define __EROFS_FS_COMPRESS_H
|
|
|
|
#include "internal.h"
|
|
|
|
struct z_erofs_decompress_req {
|
|
struct super_block *sb;
|
|
struct page **in, **out;
|
|
|
|
unsigned short pageofs_out;
|
|
unsigned int inputsize, outputsize;
|
|
|
|
/* indicate the algorithm will be used for decompression */
|
|
unsigned int alg;
|
|
bool inplace_io, partial_decoding;
|
|
};
|
|
|
|
struct z_erofs_decompressor {
|
|
int (*decompress)(struct z_erofs_decompress_req *rq,
|
|
struct list_head *pagepool);
|
|
char *name;
|
|
};
|
|
|
|
/* some special page->private (unsigned long, see below) */
|
|
#define Z_EROFS_SHORTLIVED_PAGE (-1UL << 2)
|
|
#define Z_EROFS_PREALLOCATED_PAGE (-2UL << 2)
|
|
|
|
/*
|
|
* For all pages in a pcluster, page->private should be one of
|
|
* Type Last 2bits page->private
|
|
* short-lived page 00 Z_EROFS_SHORTLIVED_PAGE
|
|
* preallocated page (tryalloc) 00 Z_EROFS_PREALLOCATED_PAGE
|
|
* cached/managed page 00 pointer to z_erofs_pcluster
|
|
* online page (file-backed, 01/10/11 sub-index << 2 | count
|
|
* some pages can be used for inplace I/O)
|
|
*
|
|
* page->mapping should be one of
|
|
* Type page->mapping
|
|
* short-lived page NULL
|
|
* preallocated page NULL
|
|
* cached/managed page non-NULL or NULL (invalidated/truncated page)
|
|
* online page non-NULL
|
|
*
|
|
* For all managed pages, PG_private should be set with 1 extra refcount,
|
|
* which is used for page reclaim / migration.
|
|
*/
|
|
|
|
/*
|
|
* short-lived pages are pages directly from buddy system with specific
|
|
* page->private (no need to set PagePrivate since these are non-LRU /
|
|
* non-movable pages and bypass reclaim / migration code).
|
|
*/
|
|
static inline bool z_erofs_is_shortlived_page(struct page *page)
|
|
{
|
|
if (page->private != Z_EROFS_SHORTLIVED_PAGE)
|
|
return false;
|
|
|
|
DBG_BUGON(page->mapping);
|
|
return true;
|
|
}
|
|
|
|
static inline bool z_erofs_put_shortlivedpage(struct list_head *pagepool,
|
|
struct page *page)
|
|
{
|
|
if (!z_erofs_is_shortlived_page(page))
|
|
return false;
|
|
|
|
/* short-lived pages should not be used by others at the same time */
|
|
if (page_ref_count(page) > 1) {
|
|
put_page(page);
|
|
} else {
|
|
/* follow the pcluster rule above. */
|
|
set_page_private(page, 0);
|
|
list_add(&page->lru, pagepool);
|
|
}
|
|
return true;
|
|
}
|
|
|
|
#define MNGD_MAPPING(sbi) ((sbi)->managed_cache->i_mapping)
|
|
static inline bool erofs_page_is_managed(const struct erofs_sb_info *sbi,
|
|
struct page *page)
|
|
{
|
|
return page->mapping == MNGD_MAPPING(sbi);
|
|
}
|
|
|
|
int z_erofs_decompress(struct z_erofs_decompress_req *rq,
|
|
struct list_head *pagepool);
|
|
|
|
/* prototypes for specific algorithms */
|
|
int z_erofs_lzma_decompress(struct z_erofs_decompress_req *rq,
|
|
struct list_head *pagepool);
|
|
#endif
|