mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
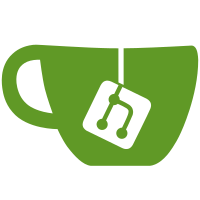
Pull x86/pti updates from Thomas Gleixner: "Another set of melted spectrum related changes: - Code simplifications and cleanups for RSB and retpolines. - Make the indirect calls in KVM speculation safe. - Whitelist CPUs which are known not to speculate from Meltdown and prepare for the new CPUID flag which tells the kernel that a CPU is not affected. - A less rigorous variant of the module retpoline check which merily warns when a non-retpoline protected module is loaded and reflects that fact in the sysfs file. - Prepare for Indirect Branch Prediction Barrier support. - Prepare for exposure of the Speculation Control MSRs to guests, so guest OSes which depend on those "features" can use them. Includes a blacklist of the broken microcodes. The actual exposure of the MSRs through KVM is still being worked on" * 'x86-pti-for-linus' of git://git.kernel.org/pub/scm/linux/kernel/git/tip/tip: x86/speculation: Simplify indirect_branch_prediction_barrier() x86/retpoline: Simplify vmexit_fill_RSB() x86/cpufeatures: Clean up Spectre v2 related CPUID flags x86/cpu/bugs: Make retpoline module warning conditional x86/bugs: Drop one "mitigation" from dmesg x86/nospec: Fix header guards names x86/alternative: Print unadorned pointers x86/speculation: Add basic IBPB (Indirect Branch Prediction Barrier) support x86/cpufeature: Blacklist SPEC_CTRL/PRED_CMD on early Spectre v2 microcodes x86/pti: Do not enable PTI on CPUs which are not vulnerable to Meltdown x86/msr: Add definitions for new speculation control MSRs x86/cpufeatures: Add AMD feature bits for Speculation Control x86/cpufeatures: Add Intel feature bits for Speculation Control x86/cpufeatures: Add CPUID_7_EDX CPUID leaf module/retpoline: Warn about missing retpoline in module KVM: VMX: Make indirect call speculation safe KVM: x86: Make indirect calls in emulator speculation safe
81 lines
2 KiB
C
81 lines
2 KiB
C
/*
|
|
* Routines to identify additional cpu features that are scattered in
|
|
* cpuid space.
|
|
*/
|
|
#include <linux/cpu.h>
|
|
|
|
#include <asm/pat.h>
|
|
#include <asm/processor.h>
|
|
|
|
#include <asm/apic.h>
|
|
|
|
struct cpuid_bit {
|
|
u16 feature;
|
|
u8 reg;
|
|
u8 bit;
|
|
u32 level;
|
|
u32 sub_leaf;
|
|
};
|
|
|
|
/* Please keep the leaf sorted by cpuid_bit.level for faster search. */
|
|
static const struct cpuid_bit cpuid_bits[] = {
|
|
{ X86_FEATURE_APERFMPERF, CPUID_ECX, 0, 0x00000006, 0 },
|
|
{ X86_FEATURE_EPB, CPUID_ECX, 3, 0x00000006, 0 },
|
|
{ X86_FEATURE_CAT_L3, CPUID_EBX, 1, 0x00000010, 0 },
|
|
{ X86_FEATURE_CAT_L2, CPUID_EBX, 2, 0x00000010, 0 },
|
|
{ X86_FEATURE_CDP_L3, CPUID_ECX, 2, 0x00000010, 1 },
|
|
{ X86_FEATURE_CDP_L2, CPUID_ECX, 2, 0x00000010, 2 },
|
|
{ X86_FEATURE_MBA, CPUID_EBX, 3, 0x00000010, 0 },
|
|
{ X86_FEATURE_HW_PSTATE, CPUID_EDX, 7, 0x80000007, 0 },
|
|
{ X86_FEATURE_CPB, CPUID_EDX, 9, 0x80000007, 0 },
|
|
{ X86_FEATURE_PROC_FEEDBACK, CPUID_EDX, 11, 0x80000007, 0 },
|
|
{ X86_FEATURE_SME, CPUID_EAX, 0, 0x8000001f, 0 },
|
|
{ 0, 0, 0, 0, 0 }
|
|
};
|
|
|
|
void init_scattered_cpuid_features(struct cpuinfo_x86 *c)
|
|
{
|
|
u32 max_level;
|
|
u32 regs[4];
|
|
const struct cpuid_bit *cb;
|
|
|
|
for (cb = cpuid_bits; cb->feature; cb++) {
|
|
|
|
/* Verify that the level is valid */
|
|
max_level = cpuid_eax(cb->level & 0xffff0000);
|
|
if (max_level < cb->level ||
|
|
max_level > (cb->level | 0xffff))
|
|
continue;
|
|
|
|
cpuid_count(cb->level, cb->sub_leaf, ®s[CPUID_EAX],
|
|
®s[CPUID_EBX], ®s[CPUID_ECX],
|
|
®s[CPUID_EDX]);
|
|
|
|
if (regs[cb->reg] & (1 << cb->bit))
|
|
set_cpu_cap(c, cb->feature);
|
|
}
|
|
}
|
|
|
|
u32 get_scattered_cpuid_leaf(unsigned int level, unsigned int sub_leaf,
|
|
enum cpuid_regs_idx reg)
|
|
{
|
|
const struct cpuid_bit *cb;
|
|
u32 cpuid_val = 0;
|
|
|
|
for (cb = cpuid_bits; cb->feature; cb++) {
|
|
|
|
if (level > cb->level)
|
|
continue;
|
|
|
|
if (level < cb->level)
|
|
break;
|
|
|
|
if (reg == cb->reg && sub_leaf == cb->sub_leaf) {
|
|
if (cpu_has(&boot_cpu_data, cb->feature))
|
|
cpuid_val |= BIT(cb->bit);
|
|
}
|
|
}
|
|
|
|
return cpuid_val;
|
|
}
|
|
EXPORT_SYMBOL_GPL(get_scattered_cpuid_leaf);
|