mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-28 07:13:34 +00:00
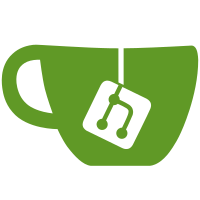
This patch updates dma_buf_vmap() and dma-buf's vmap callback to use struct dma_buf_map. The interfaces used to return a buffer address. This address now gets stored in an instance of the structure that is given as an additional argument. The functions return an errno code on errors. Users of the functions are updated accordingly. This is only an interface change. It is currently expected that dma-buf memory can be accessed with system memory load/store operations. v3: * update fastrpc driver (kernel test robot) v2: * always clear map parameter in dma_buf_vmap() (Daniel) * include dma-buf-heaps and i915 selftests (kernel test robot) Signed-off-by: Thomas Zimmermann <tzimmermann@suse.de> Acked-by: Sumit Semwal <sumit.semwal@linaro.org> Acked-by: Christian König <christian.koenig@amd.com> Acked-by: Daniel Vetter <daniel.vetter@ffwll.ch> Acked-by: Tomasz Figa <tfiga@chromium.org> Acked-by: Sam Ravnborg <sam@ravnborg.org> Link: https://patchwork.freedesktop.org/patch/msgid/20200925115601.23955-3-tzimmermann@suse.de
95 lines
2.4 KiB
C
95 lines
2.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* Pointer to dma-buf-mapped memory, plus helpers.
|
|
*/
|
|
|
|
#ifndef __DMA_BUF_MAP_H__
|
|
#define __DMA_BUF_MAP_H__
|
|
|
|
#include <linux/io.h>
|
|
|
|
/**
|
|
* struct dma_buf_map - Pointer to vmap'ed dma-buf memory.
|
|
* @vaddr_iomem: The buffer's address if in I/O memory
|
|
* @vaddr: The buffer's address if in system memory
|
|
* @is_iomem: True if the dma-buf memory is located in I/O
|
|
* memory, or false otherwise.
|
|
*/
|
|
struct dma_buf_map {
|
|
union {
|
|
void __iomem *vaddr_iomem;
|
|
void *vaddr;
|
|
};
|
|
bool is_iomem;
|
|
};
|
|
|
|
/**
|
|
* dma_buf_map_set_vaddr - Sets a dma-buf mapping structure to an address in system memory
|
|
* @map: The dma-buf mapping structure
|
|
* @vaddr: A system-memory address
|
|
*
|
|
* Sets the address and clears the I/O-memory flag.
|
|
*/
|
|
static inline void dma_buf_map_set_vaddr(struct dma_buf_map *map, void *vaddr)
|
|
{
|
|
map->vaddr = vaddr;
|
|
map->is_iomem = false;
|
|
}
|
|
|
|
/* API transition helper */
|
|
static inline bool dma_buf_map_is_vaddr(const struct dma_buf_map *map, const void *vaddr)
|
|
{
|
|
return !map->is_iomem && (map->vaddr == vaddr);
|
|
}
|
|
|
|
/**
|
|
* dma_buf_map_is_null - Tests for a dma-buf mapping to be NULL
|
|
* @map: The dma-buf mapping structure
|
|
*
|
|
* Depending on the state of struct dma_buf_map.is_iomem, tests if the
|
|
* mapping is NULL.
|
|
*
|
|
* Returns:
|
|
* True if the mapping is NULL, or false otherwise.
|
|
*/
|
|
static inline bool dma_buf_map_is_null(const struct dma_buf_map *map)
|
|
{
|
|
if (map->is_iomem)
|
|
return !map->vaddr_iomem;
|
|
return !map->vaddr;
|
|
}
|
|
|
|
/**
|
|
* dma_buf_map_is_set - Tests is the dma-buf mapping has been set
|
|
* @map: The dma-buf mapping structure
|
|
*
|
|
* Depending on the state of struct dma_buf_map.is_iomem, tests if the
|
|
* mapping has been set.
|
|
*
|
|
* Returns:
|
|
* True if the mapping is been set, or false otherwise.
|
|
*/
|
|
static inline bool dma_buf_map_is_set(const struct dma_buf_map *map)
|
|
{
|
|
return !dma_buf_map_is_null(map);
|
|
}
|
|
|
|
/**
|
|
* dma_buf_map_clear - Clears a dma-buf mapping structure
|
|
* @map: The dma-buf mapping structure
|
|
*
|
|
* Clears all fields to zero; including struct dma_buf_map.is_iomem. So
|
|
* mapping structures that were set to point to I/O memory are reset for
|
|
* system memory. Pointers are cleared to NULL. This is the default.
|
|
*/
|
|
static inline void dma_buf_map_clear(struct dma_buf_map *map)
|
|
{
|
|
if (map->is_iomem) {
|
|
map->vaddr_iomem = NULL;
|
|
map->is_iomem = false;
|
|
} else {
|
|
map->vaddr = NULL;
|
|
}
|
|
}
|
|
|
|
#endif /* __DMA_BUF_MAP_H__ */
|