mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-21 00:10:09 +00:00
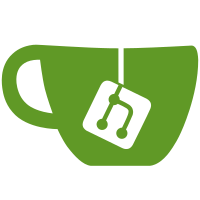
We expect no warnings to be issued when we specify __GFP_NOWARN, but currently in paths like alloc_pages() and kmalloc(), there are still some warnings printed, fix it. But for some warnings that report usage problems, we don't deal with them. If such warnings are printed, then we should fix the usage problems. Such as the following case: WARN_ON_ONCE((gfp_flags & __GFP_NOFAIL) && (order > 1)); [zhengqi.arch@bytedance.com: v2] Link: https://lkml.kernel.org/r/20220511061951.1114-1-zhengqi.arch@bytedance.com Link: https://lkml.kernel.org/r/20220510113809.80626-1-zhengqi.arch@bytedance.com Signed-off-by: Qi Zheng <zhengqi.arch@bytedance.com> Cc: Akinobu Mita <akinobu.mita@gmail.com> Cc: Vlastimil Babka <vbabka@suse.cz> Cc: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Cc: Jiri Slaby <jirislaby@kernel.org> Cc: Steven Rostedt (Google) <rostedt@goodmis.org> Signed-off-by: Andrew Morton <akpm@linux-foundation.org>
65 lines
1.4 KiB
C
65 lines
1.4 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
#include <linux/fault-inject.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/mm.h>
|
|
#include "slab.h"
|
|
|
|
static struct {
|
|
struct fault_attr attr;
|
|
bool ignore_gfp_reclaim;
|
|
bool cache_filter;
|
|
} failslab = {
|
|
.attr = FAULT_ATTR_INITIALIZER,
|
|
.ignore_gfp_reclaim = true,
|
|
.cache_filter = false,
|
|
};
|
|
|
|
bool __should_failslab(struct kmem_cache *s, gfp_t gfpflags)
|
|
{
|
|
/* No fault-injection for bootstrap cache */
|
|
if (unlikely(s == kmem_cache))
|
|
return false;
|
|
|
|
if (gfpflags & __GFP_NOFAIL)
|
|
return false;
|
|
|
|
if (failslab.ignore_gfp_reclaim &&
|
|
(gfpflags & __GFP_DIRECT_RECLAIM))
|
|
return false;
|
|
|
|
if (failslab.cache_filter && !(s->flags & SLAB_FAILSLAB))
|
|
return false;
|
|
|
|
if (gfpflags & __GFP_NOWARN)
|
|
failslab.attr.no_warn = true;
|
|
|
|
return should_fail(&failslab.attr, s->object_size);
|
|
}
|
|
|
|
static int __init setup_failslab(char *str)
|
|
{
|
|
return setup_fault_attr(&failslab.attr, str);
|
|
}
|
|
__setup("failslab=", setup_failslab);
|
|
|
|
#ifdef CONFIG_FAULT_INJECTION_DEBUG_FS
|
|
static int __init failslab_debugfs_init(void)
|
|
{
|
|
struct dentry *dir;
|
|
umode_t mode = S_IFREG | 0600;
|
|
|
|
dir = fault_create_debugfs_attr("failslab", NULL, &failslab.attr);
|
|
if (IS_ERR(dir))
|
|
return PTR_ERR(dir);
|
|
|
|
debugfs_create_bool("ignore-gfp-wait", mode, dir,
|
|
&failslab.ignore_gfp_reclaim);
|
|
debugfs_create_bool("cache-filter", mode, dir,
|
|
&failslab.cache_filter);
|
|
|
|
return 0;
|
|
}
|
|
|
|
late_initcall(failslab_debugfs_init);
|
|
|
|
#endif /* CONFIG_FAULT_INJECTION_DEBUG_FS */
|