mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-11 19:19:42 +00:00
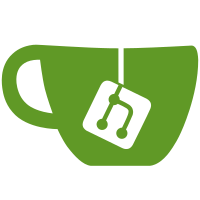
Device drivers use get_device_system_crosststamp() to produce precise system/device cross-timestamps. The PHC clock and ALSA interfaces, for example, make the cross-timestamps available to user applications. On Intel platforms, get_device_system_crosststamp() requires a TSC value derived from ART (Always Running Timer) to compute the monotonic raw and realtime system timestamps. Starting with Intel Goldmont platforms, the PCIe root complex supports the PTM time sync protocol. PTM requires all timestamps to be in units of nanoseconds. The Intel root complex hardware propagates system time derived from ART in units of nanoseconds performing the conversion as follows: ART_NS = ART * 1e9 / <crystal frequency> When user software requests a cross-timestamp, the system timestamps (generally read from device registers) must be converted to TSC by the driver software as follows: TSC = ART_NS * TSC_KHZ / 1e6 This is valid when CPU feature flag X86_FEATURE_TSC_KNOWN_FREQ is set indicating that tsc_khz is derived from CPUID[15H]. Drivers should check whether this flag is set before conversion to TSC is attempted. Suggested-by: Christopher S. Hall <christopher.s.hall@intel.com> Signed-off-by: Rajvi Jingar <rajvi.jingar@intel.com> Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Cc: peterz@infradead.org Link: https://lkml.kernel.org/r/1520530116-4925-1-git-send-email-rajvi.jingar@intel.com
75 lines
2 KiB
C
75 lines
2 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* x86 TSC related functions
|
|
*/
|
|
#ifndef _ASM_X86_TSC_H
|
|
#define _ASM_X86_TSC_H
|
|
|
|
#include <asm/processor.h>
|
|
|
|
#define NS_SCALE 10 /* 2^10, carefully chosen */
|
|
#define US_SCALE 32 /* 2^32, arbitralrily chosen */
|
|
|
|
/*
|
|
* Standard way to access the cycle counter.
|
|
*/
|
|
typedef unsigned long long cycles_t;
|
|
|
|
extern unsigned int cpu_khz;
|
|
extern unsigned int tsc_khz;
|
|
|
|
extern void disable_TSC(void);
|
|
|
|
static inline cycles_t get_cycles(void)
|
|
{
|
|
#ifndef CONFIG_X86_TSC
|
|
if (!boot_cpu_has(X86_FEATURE_TSC))
|
|
return 0;
|
|
#endif
|
|
|
|
return rdtsc();
|
|
}
|
|
|
|
extern struct system_counterval_t convert_art_to_tsc(u64 art);
|
|
extern struct system_counterval_t convert_art_ns_to_tsc(u64 art_ns);
|
|
|
|
extern void tsc_early_delay_calibrate(void);
|
|
extern void tsc_init(void);
|
|
extern void mark_tsc_unstable(char *reason);
|
|
extern int unsynchronized_tsc(void);
|
|
extern int check_tsc_unstable(void);
|
|
extern void mark_tsc_async_resets(char *reason);
|
|
extern unsigned long native_calibrate_cpu(void);
|
|
extern unsigned long native_calibrate_tsc(void);
|
|
extern unsigned long long native_sched_clock_from_tsc(u64 tsc);
|
|
|
|
extern int tsc_clocksource_reliable;
|
|
#ifdef CONFIG_X86_TSC
|
|
extern bool tsc_async_resets;
|
|
#else
|
|
# define tsc_async_resets false
|
|
#endif
|
|
|
|
/*
|
|
* Boot-time check whether the TSCs are synchronized across
|
|
* all CPUs/cores:
|
|
*/
|
|
#ifdef CONFIG_X86_TSC
|
|
extern bool tsc_store_and_check_tsc_adjust(bool bootcpu);
|
|
extern void tsc_verify_tsc_adjust(bool resume);
|
|
extern void check_tsc_sync_source(int cpu);
|
|
extern void check_tsc_sync_target(void);
|
|
#else
|
|
static inline bool tsc_store_and_check_tsc_adjust(bool bootcpu) { return false; }
|
|
static inline void tsc_verify_tsc_adjust(bool resume) { }
|
|
static inline void check_tsc_sync_source(int cpu) { }
|
|
static inline void check_tsc_sync_target(void) { }
|
|
#endif
|
|
|
|
extern int notsc_setup(char *);
|
|
extern void tsc_save_sched_clock_state(void);
|
|
extern void tsc_restore_sched_clock_state(void);
|
|
|
|
unsigned long cpu_khz_from_msr(void);
|
|
|
|
#endif /* _ASM_X86_TSC_H */
|