mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-28 20:02:17 +00:00
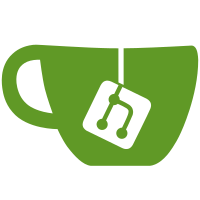
6ab428604f
("cgroup: Implement DEBUG_CGROUP_REF") added a config option which forces cgroup refcnt functions to be not inlined so that they can be kprobed for debugging. However, it forgot export them when the config is enabled breaking modules which make use of css reference counting. Fix it by adding CGROUP_REF_EXPORT() macro to cgroup_refcnt.h which is defined to EXPORT_SYMBOL_GPL when CONFIG_DEBUG_CGROUP_REF is set. Signed-off-by: Tejun Heo <tj@kernel.org> Fixes:6ab428604f
("cgroup: Implement DEBUG_CGROUP_REF")
96 lines
2.6 KiB
C
96 lines
2.6 KiB
C
/**
|
|
* css_get - obtain a reference on the specified css
|
|
* @css: target css
|
|
*
|
|
* The caller must already have a reference.
|
|
*/
|
|
CGROUP_REF_FN_ATTRS
|
|
void css_get(struct cgroup_subsys_state *css)
|
|
{
|
|
if (!(css->flags & CSS_NO_REF))
|
|
percpu_ref_get(&css->refcnt);
|
|
}
|
|
CGROUP_REF_EXPORT(css_get)
|
|
|
|
/**
|
|
* css_get_many - obtain references on the specified css
|
|
* @css: target css
|
|
* @n: number of references to get
|
|
*
|
|
* The caller must already have a reference.
|
|
*/
|
|
CGROUP_REF_FN_ATTRS
|
|
void css_get_many(struct cgroup_subsys_state *css, unsigned int n)
|
|
{
|
|
if (!(css->flags & CSS_NO_REF))
|
|
percpu_ref_get_many(&css->refcnt, n);
|
|
}
|
|
CGROUP_REF_EXPORT(css_get_many)
|
|
|
|
/**
|
|
* css_tryget - try to obtain a reference on the specified css
|
|
* @css: target css
|
|
*
|
|
* Obtain a reference on @css unless it already has reached zero and is
|
|
* being released. This function doesn't care whether @css is on or
|
|
* offline. The caller naturally needs to ensure that @css is accessible
|
|
* but doesn't have to be holding a reference on it - IOW, RCU protected
|
|
* access is good enough for this function. Returns %true if a reference
|
|
* count was successfully obtained; %false otherwise.
|
|
*/
|
|
CGROUP_REF_FN_ATTRS
|
|
bool css_tryget(struct cgroup_subsys_state *css)
|
|
{
|
|
if (!(css->flags & CSS_NO_REF))
|
|
return percpu_ref_tryget(&css->refcnt);
|
|
return true;
|
|
}
|
|
CGROUP_REF_EXPORT(css_tryget)
|
|
|
|
/**
|
|
* css_tryget_online - try to obtain a reference on the specified css if online
|
|
* @css: target css
|
|
*
|
|
* Obtain a reference on @css if it's online. The caller naturally needs
|
|
* to ensure that @css is accessible but doesn't have to be holding a
|
|
* reference on it - IOW, RCU protected access is good enough for this
|
|
* function. Returns %true if a reference count was successfully obtained;
|
|
* %false otherwise.
|
|
*/
|
|
CGROUP_REF_FN_ATTRS
|
|
bool css_tryget_online(struct cgroup_subsys_state *css)
|
|
{
|
|
if (!(css->flags & CSS_NO_REF))
|
|
return percpu_ref_tryget_live(&css->refcnt);
|
|
return true;
|
|
}
|
|
CGROUP_REF_EXPORT(css_tryget_online)
|
|
|
|
/**
|
|
* css_put - put a css reference
|
|
* @css: target css
|
|
*
|
|
* Put a reference obtained via css_get() and css_tryget_online().
|
|
*/
|
|
CGROUP_REF_FN_ATTRS
|
|
void css_put(struct cgroup_subsys_state *css)
|
|
{
|
|
if (!(css->flags & CSS_NO_REF))
|
|
percpu_ref_put(&css->refcnt);
|
|
}
|
|
CGROUP_REF_EXPORT(css_put)
|
|
|
|
/**
|
|
* css_put_many - put css references
|
|
* @css: target css
|
|
* @n: number of references to put
|
|
*
|
|
* Put references obtained via css_get() and css_tryget_online().
|
|
*/
|
|
CGROUP_REF_FN_ATTRS
|
|
void css_put_many(struct cgroup_subsys_state *css, unsigned int n)
|
|
{
|
|
if (!(css->flags & CSS_NO_REF))
|
|
percpu_ref_put_many(&css->refcnt, n);
|
|
}
|
|
CGROUP_REF_EXPORT(css_put_many)
|