mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-28 20:02:17 +00:00
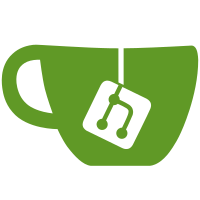
In preparation for hmem platform devices to be unregistered, stop using platform_device_add_resources() to convey the address range. The platform_device_add_resources() API causes an existing "Soft Reserved" iomem resource to be re-parented under an inserted platform device resource. When that platform device is deleted it removes the platform device resource and all children. Instead, it is sufficient to convey just the address range and let request_mem_region() insert resources to indicate the devices active in the range. This allows the "Soft Reserved" resource to be re-enumerated upon the next probe event. Reviewed-by: Jonathan Cameron <Jonathan.Cameron@huawei.com> Tested-by: Fan Ni <fan.ni@samsung.com> Reviewed-by: Dave Jiang <dave.jiang@intel.com> Link: https://lore.kernel.org/r/167602002217.1924368.7036275892522551624.stgit@dwillia2-xfh.jf.intel.com Signed-off-by: Dan Williams <dan.j.williams@intel.com>
63 lines
1.8 KiB
C
63 lines
1.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _MEMREGION_H_
|
|
#define _MEMREGION_H_
|
|
#include <linux/types.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/range.h>
|
|
#include <linux/bug.h>
|
|
|
|
struct memregion_info {
|
|
int target_node;
|
|
struct range range;
|
|
};
|
|
|
|
#ifdef CONFIG_MEMREGION
|
|
int memregion_alloc(gfp_t gfp);
|
|
void memregion_free(int id);
|
|
#else
|
|
static inline int memregion_alloc(gfp_t gfp)
|
|
{
|
|
return -ENOMEM;
|
|
}
|
|
static inline void memregion_free(int id)
|
|
{
|
|
}
|
|
#endif
|
|
|
|
/**
|
|
* cpu_cache_invalidate_memregion - drop any CPU cached data for
|
|
* memregions described by @res_desc
|
|
* @res_desc: one of the IORES_DESC_* types
|
|
*
|
|
* Perform cache maintenance after a memory event / operation that
|
|
* changes the contents of physical memory in a cache-incoherent manner.
|
|
* For example, device memory technologies like NVDIMM and CXL have
|
|
* device secure erase, and dynamic region provision that can replace
|
|
* the memory mapped to a given physical address.
|
|
*
|
|
* Limit the functionality to architectures that have an efficient way
|
|
* to writeback and invalidate potentially terabytes of address space at
|
|
* once. Note that this routine may or may not write back any dirty
|
|
* contents while performing the invalidation. It is only exported for
|
|
* the explicit usage of the NVDIMM and CXL modules in the 'DEVMEM'
|
|
* symbol namespace on bare platforms.
|
|
*
|
|
* Returns 0 on success or negative error code on a failure to perform
|
|
* the cache maintenance.
|
|
*/
|
|
#ifdef CONFIG_ARCH_HAS_CPU_CACHE_INVALIDATE_MEMREGION
|
|
int cpu_cache_invalidate_memregion(int res_desc);
|
|
bool cpu_cache_has_invalidate_memregion(void);
|
|
#else
|
|
static inline bool cpu_cache_has_invalidate_memregion(void)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
static inline int cpu_cache_invalidate_memregion(int res_desc)
|
|
{
|
|
WARN_ON_ONCE("CPU cache invalidation required");
|
|
return -ENXIO;
|
|
}
|
|
#endif
|
|
#endif /* _MEMREGION_H_ */
|