mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-28 20:02:17 +00:00
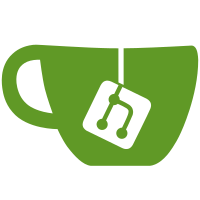
Current some drivers(like iscsi) call transport_register_device()
failed, they don't call transport_destroy_device() to release the
memory allocated in transport_setup_device(), because they don't
know what was done, it should be internal thing to release the
resource in register function. So fix this leak by calling destroy
function inside register function.
Fixes: 1da177e4c3
("Linux-2.6.12-rc2")
Signed-off-by: Yang Yingliang <yangyingliang@huawei.com>
Link: https://lore.kernel.org/r/20221110102307.3492557-1-yangyingliang@huawei.com
Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
107 lines
2.6 KiB
C
107 lines
2.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* transport_class.h - a generic container for all transport classes
|
|
*
|
|
* Copyright (c) 2005 - James Bottomley <James.Bottomley@steeleye.com>
|
|
*/
|
|
|
|
#ifndef _TRANSPORT_CLASS_H_
|
|
#define _TRANSPORT_CLASS_H_
|
|
|
|
#include <linux/device.h>
|
|
#include <linux/bug.h>
|
|
#include <linux/attribute_container.h>
|
|
|
|
struct transport_container;
|
|
|
|
struct transport_class {
|
|
struct class class;
|
|
int (*setup)(struct transport_container *, struct device *,
|
|
struct device *);
|
|
int (*configure)(struct transport_container *, struct device *,
|
|
struct device *);
|
|
int (*remove)(struct transport_container *, struct device *,
|
|
struct device *);
|
|
};
|
|
|
|
#define DECLARE_TRANSPORT_CLASS(cls, nm, su, rm, cfg) \
|
|
struct transport_class cls = { \
|
|
.class = { \
|
|
.name = nm, \
|
|
}, \
|
|
.setup = su, \
|
|
.remove = rm, \
|
|
.configure = cfg, \
|
|
}
|
|
|
|
|
|
struct anon_transport_class {
|
|
struct transport_class tclass;
|
|
struct attribute_container container;
|
|
};
|
|
|
|
#define DECLARE_ANON_TRANSPORT_CLASS(cls, mtch, cfg) \
|
|
struct anon_transport_class cls = { \
|
|
.tclass = { \
|
|
.configure = cfg, \
|
|
}, \
|
|
. container = { \
|
|
.match = mtch, \
|
|
}, \
|
|
}
|
|
|
|
#define class_to_transport_class(x) \
|
|
container_of(x, struct transport_class, class)
|
|
|
|
struct transport_container {
|
|
struct attribute_container ac;
|
|
const struct attribute_group *statistics;
|
|
};
|
|
|
|
#define attribute_container_to_transport_container(x) \
|
|
container_of(x, struct transport_container, ac)
|
|
|
|
void transport_remove_device(struct device *);
|
|
int transport_add_device(struct device *);
|
|
void transport_setup_device(struct device *);
|
|
void transport_configure_device(struct device *);
|
|
void transport_destroy_device(struct device *);
|
|
|
|
static inline int
|
|
transport_register_device(struct device *dev)
|
|
{
|
|
int ret;
|
|
|
|
transport_setup_device(dev);
|
|
ret = transport_add_device(dev);
|
|
if (ret)
|
|
transport_destroy_device(dev);
|
|
|
|
return ret;
|
|
}
|
|
|
|
static inline void
|
|
transport_unregister_device(struct device *dev)
|
|
{
|
|
transport_remove_device(dev);
|
|
transport_destroy_device(dev);
|
|
}
|
|
|
|
static inline int transport_container_register(struct transport_container *tc)
|
|
{
|
|
return attribute_container_register(&tc->ac);
|
|
}
|
|
|
|
static inline void transport_container_unregister(struct transport_container *tc)
|
|
{
|
|
if (unlikely(attribute_container_unregister(&tc->ac)))
|
|
BUG();
|
|
}
|
|
|
|
int transport_class_register(struct transport_class *);
|
|
int anon_transport_class_register(struct anon_transport_class *);
|
|
void transport_class_unregister(struct transport_class *);
|
|
void anon_transport_class_unregister(struct anon_transport_class *);
|
|
|
|
|
|
#endif
|