mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
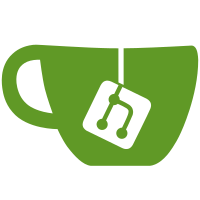
Currently the stack supports time stamping in PHY devices. However, there are newer, non-PHY devices that can snoop an MII bus and provide time stamps. In order to support such devices, this patch introduces a new interface to be used by both PHY and non-PHY devices. In addition, the one and only user of the old PHY time stamping API is converted to the new interface. Signed-off-by: Richard Cochran <richardcochran@gmail.com> Reviewed-by: Andrew Lunn <andrew@lunn.ch> Signed-off-by: David S. Miller <davem@davemloft.net>
71 lines
1.5 KiB
C
71 lines
1.5 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* PTP 1588 clock support - support for timestamping in PHY devices
|
|
*
|
|
* Copyright (C) 2010 OMICRON electronics GmbH
|
|
*/
|
|
#include <linux/errqueue.h>
|
|
#include <linux/phy.h>
|
|
#include <linux/ptp_classify.h>
|
|
#include <linux/skbuff.h>
|
|
#include <linux/export.h>
|
|
|
|
static unsigned int classify(const struct sk_buff *skb)
|
|
{
|
|
if (likely(skb->dev && skb->dev->phydev &&
|
|
skb->dev->phydev->mii_ts))
|
|
return ptp_classify_raw(skb);
|
|
else
|
|
return PTP_CLASS_NONE;
|
|
}
|
|
|
|
void skb_clone_tx_timestamp(struct sk_buff *skb)
|
|
{
|
|
struct mii_timestamper *mii_ts;
|
|
struct sk_buff *clone;
|
|
unsigned int type;
|
|
|
|
if (!skb->sk)
|
|
return;
|
|
|
|
type = classify(skb);
|
|
if (type == PTP_CLASS_NONE)
|
|
return;
|
|
|
|
mii_ts = skb->dev->phydev->mii_ts;
|
|
if (likely(mii_ts->txtstamp)) {
|
|
clone = skb_clone_sk(skb);
|
|
if (!clone)
|
|
return;
|
|
mii_ts->txtstamp(mii_ts, clone, type);
|
|
}
|
|
}
|
|
EXPORT_SYMBOL_GPL(skb_clone_tx_timestamp);
|
|
|
|
bool skb_defer_rx_timestamp(struct sk_buff *skb)
|
|
{
|
|
struct mii_timestamper *mii_ts;
|
|
unsigned int type;
|
|
|
|
if (!skb->dev || !skb->dev->phydev || !skb->dev->phydev->mii_ts)
|
|
return false;
|
|
|
|
if (skb_headroom(skb) < ETH_HLEN)
|
|
return false;
|
|
|
|
__skb_push(skb, ETH_HLEN);
|
|
|
|
type = ptp_classify_raw(skb);
|
|
|
|
__skb_pull(skb, ETH_HLEN);
|
|
|
|
if (type == PTP_CLASS_NONE)
|
|
return false;
|
|
|
|
mii_ts = skb->dev->phydev->mii_ts;
|
|
if (likely(mii_ts->rxtstamp))
|
|
return mii_ts->rxtstamp(mii_ts, skb, type);
|
|
|
|
return false;
|
|
}
|
|
EXPORT_SYMBOL_GPL(skb_defer_rx_timestamp);
|