mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-02 23:27:06 +00:00
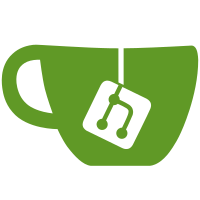
The number of entries in the rsrc node cache is limited to 512, which still seems unnecessarily large. Add per cache thresholds and set to to 32 for the rsrc node cache. Signed-off-by: Pavel Begunkov <asml.silence@gmail.com> Link: https://lore.kernel.org/r/d0cd538b944dac0bf878e276fc0199f21e6bccea.1680576071.git.asml.silence@gmail.com Signed-off-by: Jens Axboe <axboe@kernel.dk>
62 lines
1.3 KiB
C
62 lines
1.3 KiB
C
#ifndef IOU_ALLOC_CACHE_H
|
|
#define IOU_ALLOC_CACHE_H
|
|
|
|
/*
|
|
* Don't allow the cache to grow beyond this size.
|
|
*/
|
|
#define IO_ALLOC_CACHE_MAX 512
|
|
|
|
struct io_cache_entry {
|
|
struct io_wq_work_node node;
|
|
};
|
|
|
|
static inline bool io_alloc_cache_put(struct io_alloc_cache *cache,
|
|
struct io_cache_entry *entry)
|
|
{
|
|
if (cache->nr_cached < cache->max_cached) {
|
|
cache->nr_cached++;
|
|
wq_stack_add_head(&entry->node, &cache->list);
|
|
/* KASAN poisons object */
|
|
kasan_slab_free_mempool(entry);
|
|
return true;
|
|
}
|
|
return false;
|
|
}
|
|
|
|
static inline struct io_cache_entry *io_alloc_cache_get(struct io_alloc_cache *cache)
|
|
{
|
|
if (cache->list.next) {
|
|
struct io_cache_entry *entry;
|
|
|
|
entry = container_of(cache->list.next, struct io_cache_entry, node);
|
|
kasan_unpoison_range(entry, cache->elem_size);
|
|
cache->list.next = cache->list.next->next;
|
|
cache->nr_cached--;
|
|
return entry;
|
|
}
|
|
|
|
return NULL;
|
|
}
|
|
|
|
static inline void io_alloc_cache_init(struct io_alloc_cache *cache,
|
|
unsigned max_nr, size_t size)
|
|
{
|
|
cache->list.next = NULL;
|
|
cache->nr_cached = 0;
|
|
cache->max_cached = max_nr;
|
|
cache->elem_size = size;
|
|
}
|
|
|
|
static inline void io_alloc_cache_free(struct io_alloc_cache *cache,
|
|
void (*free)(struct io_cache_entry *))
|
|
{
|
|
while (1) {
|
|
struct io_cache_entry *entry = io_alloc_cache_get(cache);
|
|
|
|
if (!entry)
|
|
break;
|
|
free(entry);
|
|
}
|
|
cache->nr_cached = 0;
|
|
}
|
|
#endif
|