mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-23 17:30:02 +00:00
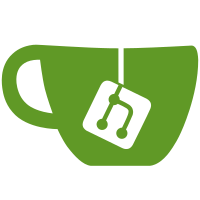
The replacement of <asm/pgrable.h> with <linux/pgtable.h> made the include of the latter in the middle of asm includes. Fix this up with the aid of the below script and manual adjustments here and there. import sys import re if len(sys.argv) is not 3: print "USAGE: %s <file> <header>" % (sys.argv[0]) sys.exit(1) hdr_to_move="#include <linux/%s>" % sys.argv[2] moved = False in_hdrs = False with open(sys.argv[1], "r") as f: lines = f.readlines() for _line in lines: line = _line.rstrip(' ') if line == hdr_to_move: continue if line.startswith("#include <linux/"): in_hdrs = True elif not moved and in_hdrs: moved = True print hdr_to_move print line Signed-off-by: Mike Rapoport <rppt@linux.ibm.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Cc: Arnd Bergmann <arnd@arndb.de> Cc: Borislav Petkov <bp@alien8.de> Cc: Brian Cain <bcain@codeaurora.org> Cc: Catalin Marinas <catalin.marinas@arm.com> Cc: Chris Zankel <chris@zankel.net> Cc: "David S. Miller" <davem@davemloft.net> Cc: Geert Uytterhoeven <geert@linux-m68k.org> Cc: Greentime Hu <green.hu@gmail.com> Cc: Greg Ungerer <gerg@linux-m68k.org> Cc: Guan Xuetao <gxt@pku.edu.cn> Cc: Guo Ren <guoren@kernel.org> Cc: Heiko Carstens <heiko.carstens@de.ibm.com> Cc: Helge Deller <deller@gmx.de> Cc: Ingo Molnar <mingo@redhat.com> Cc: Ley Foon Tan <ley.foon.tan@intel.com> Cc: Mark Salter <msalter@redhat.com> Cc: Matthew Wilcox <willy@infradead.org> Cc: Matt Turner <mattst88@gmail.com> Cc: Max Filippov <jcmvbkbc@gmail.com> Cc: Michael Ellerman <mpe@ellerman.id.au> Cc: Michal Simek <monstr@monstr.eu> Cc: Nick Hu <nickhu@andestech.com> Cc: Paul Walmsley <paul.walmsley@sifive.com> Cc: Richard Weinberger <richard@nod.at> Cc: Rich Felker <dalias@libc.org> Cc: Russell King <linux@armlinux.org.uk> Cc: Stafford Horne <shorne@gmail.com> Cc: Thomas Bogendoerfer <tsbogend@alpha.franken.de> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: Tony Luck <tony.luck@intel.com> Cc: Vincent Chen <deanbo422@gmail.com> Cc: Vineet Gupta <vgupta@synopsys.com> Cc: Will Deacon <will@kernel.org> Cc: Yoshinori Sato <ysato@users.sourceforge.jp> Link: http://lkml.kernel.org/r/20200514170327.31389-4-rppt@kernel.org Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
241 lines
5.3 KiB
C
241 lines
5.3 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* Copyright (C) 2001 Dave Engebretsen, IBM Corporation
|
|
* Copyright (C) 2003 Anton Blanchard <anton@au.ibm.com>, IBM
|
|
*
|
|
* RTAS specific routines for PCI.
|
|
*
|
|
* Based on code from pci.c, chrp_pci.c and pSeries_pci.c
|
|
*/
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/threads.h>
|
|
#include <linux/pci.h>
|
|
#include <linux/string.h>
|
|
#include <linux/init.h>
|
|
#include <linux/pgtable.h>
|
|
|
|
#include <asm/io.h>
|
|
#include <asm/irq.h>
|
|
#include <asm/prom.h>
|
|
#include <asm/machdep.h>
|
|
#include <asm/pci-bridge.h>
|
|
#include <asm/iommu.h>
|
|
#include <asm/rtas.h>
|
|
#include <asm/mpic.h>
|
|
#include <asm/ppc-pci.h>
|
|
#include <asm/eeh.h>
|
|
|
|
/* RTAS tokens */
|
|
static int read_pci_config;
|
|
static int write_pci_config;
|
|
static int ibm_read_pci_config;
|
|
static int ibm_write_pci_config;
|
|
|
|
static inline int config_access_valid(struct pci_dn *dn, int where)
|
|
{
|
|
if (where < 256)
|
|
return 1;
|
|
if (where < 4096 && dn->pci_ext_config_space)
|
|
return 1;
|
|
|
|
return 0;
|
|
}
|
|
|
|
int rtas_read_config(struct pci_dn *pdn, int where, int size, u32 *val)
|
|
{
|
|
int returnval = -1;
|
|
unsigned long buid, addr;
|
|
int ret;
|
|
|
|
if (!pdn)
|
|
return PCIBIOS_DEVICE_NOT_FOUND;
|
|
if (!config_access_valid(pdn, where))
|
|
return PCIBIOS_BAD_REGISTER_NUMBER;
|
|
#ifdef CONFIG_EEH
|
|
if (pdn->edev && pdn->edev->pe &&
|
|
(pdn->edev->pe->state & EEH_PE_CFG_BLOCKED))
|
|
return PCIBIOS_SET_FAILED;
|
|
#endif
|
|
|
|
addr = rtas_config_addr(pdn->busno, pdn->devfn, where);
|
|
buid = pdn->phb->buid;
|
|
if (buid) {
|
|
ret = rtas_call(ibm_read_pci_config, 4, 2, &returnval,
|
|
addr, BUID_HI(buid), BUID_LO(buid), size);
|
|
} else {
|
|
ret = rtas_call(read_pci_config, 2, 2, &returnval, addr, size);
|
|
}
|
|
*val = returnval;
|
|
|
|
if (ret)
|
|
return PCIBIOS_DEVICE_NOT_FOUND;
|
|
|
|
return PCIBIOS_SUCCESSFUL;
|
|
}
|
|
|
|
static int rtas_pci_read_config(struct pci_bus *bus,
|
|
unsigned int devfn,
|
|
int where, int size, u32 *val)
|
|
{
|
|
struct pci_dn *pdn;
|
|
int ret;
|
|
|
|
*val = 0xFFFFFFFF;
|
|
|
|
pdn = pci_get_pdn_by_devfn(bus, devfn);
|
|
|
|
/* Validity of pdn is checked in here */
|
|
ret = rtas_read_config(pdn, where, size, val);
|
|
if (*val == EEH_IO_ERROR_VALUE(size) &&
|
|
eeh_dev_check_failure(pdn_to_eeh_dev(pdn)))
|
|
return PCIBIOS_DEVICE_NOT_FOUND;
|
|
|
|
return ret;
|
|
}
|
|
|
|
int rtas_write_config(struct pci_dn *pdn, int where, int size, u32 val)
|
|
{
|
|
unsigned long buid, addr;
|
|
int ret;
|
|
|
|
if (!pdn)
|
|
return PCIBIOS_DEVICE_NOT_FOUND;
|
|
if (!config_access_valid(pdn, where))
|
|
return PCIBIOS_BAD_REGISTER_NUMBER;
|
|
#ifdef CONFIG_EEH
|
|
if (pdn->edev && pdn->edev->pe &&
|
|
(pdn->edev->pe->state & EEH_PE_CFG_BLOCKED))
|
|
return PCIBIOS_SET_FAILED;
|
|
#endif
|
|
|
|
addr = rtas_config_addr(pdn->busno, pdn->devfn, where);
|
|
buid = pdn->phb->buid;
|
|
if (buid) {
|
|
ret = rtas_call(ibm_write_pci_config, 5, 1, NULL, addr,
|
|
BUID_HI(buid), BUID_LO(buid), size, (ulong) val);
|
|
} else {
|
|
ret = rtas_call(write_pci_config, 3, 1, NULL, addr, size, (ulong)val);
|
|
}
|
|
|
|
if (ret)
|
|
return PCIBIOS_DEVICE_NOT_FOUND;
|
|
|
|
return PCIBIOS_SUCCESSFUL;
|
|
}
|
|
|
|
static int rtas_pci_write_config(struct pci_bus *bus,
|
|
unsigned int devfn,
|
|
int where, int size, u32 val)
|
|
{
|
|
struct pci_dn *pdn;
|
|
|
|
pdn = pci_get_pdn_by_devfn(bus, devfn);
|
|
|
|
/* Validity of pdn is checked in here. */
|
|
return rtas_write_config(pdn, where, size, val);
|
|
}
|
|
|
|
static struct pci_ops rtas_pci_ops = {
|
|
.read = rtas_pci_read_config,
|
|
.write = rtas_pci_write_config,
|
|
};
|
|
|
|
static int is_python(struct device_node *dev)
|
|
{
|
|
const char *model = of_get_property(dev, "model", NULL);
|
|
|
|
if (model && strstr(model, "Python"))
|
|
return 1;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void python_countermeasures(struct device_node *dev)
|
|
{
|
|
struct resource registers;
|
|
void __iomem *chip_regs;
|
|
volatile u32 val;
|
|
|
|
if (of_address_to_resource(dev, 0, ®isters)) {
|
|
printk(KERN_ERR "Can't get address for Python workarounds !\n");
|
|
return;
|
|
}
|
|
|
|
/* Python's register file is 1 MB in size. */
|
|
chip_regs = ioremap(registers.start & ~(0xfffffUL), 0x100000);
|
|
|
|
/*
|
|
* Firmware doesn't always clear this bit which is critical
|
|
* for good performance - Anton
|
|
*/
|
|
|
|
#define PRG_CL_RESET_VALID 0x00010000
|
|
|
|
val = in_be32(chip_regs + 0xf6030);
|
|
if (val & PRG_CL_RESET_VALID) {
|
|
printk(KERN_INFO "Python workaround: ");
|
|
val &= ~PRG_CL_RESET_VALID;
|
|
out_be32(chip_regs + 0xf6030, val);
|
|
/*
|
|
* We must read it back for changes to
|
|
* take effect
|
|
*/
|
|
val = in_be32(chip_regs + 0xf6030);
|
|
printk("reg0: %x\n", val);
|
|
}
|
|
|
|
iounmap(chip_regs);
|
|
}
|
|
|
|
void __init init_pci_config_tokens(void)
|
|
{
|
|
read_pci_config = rtas_token("read-pci-config");
|
|
write_pci_config = rtas_token("write-pci-config");
|
|
ibm_read_pci_config = rtas_token("ibm,read-pci-config");
|
|
ibm_write_pci_config = rtas_token("ibm,write-pci-config");
|
|
}
|
|
|
|
unsigned long get_phb_buid(struct device_node *phb)
|
|
{
|
|
struct resource r;
|
|
|
|
if (ibm_read_pci_config == -1)
|
|
return 0;
|
|
if (of_address_to_resource(phb, 0, &r))
|
|
return 0;
|
|
return r.start;
|
|
}
|
|
|
|
static int phb_set_bus_ranges(struct device_node *dev,
|
|
struct pci_controller *phb)
|
|
{
|
|
const __be32 *bus_range;
|
|
unsigned int len;
|
|
|
|
bus_range = of_get_property(dev, "bus-range", &len);
|
|
if (bus_range == NULL || len < 2 * sizeof(int)) {
|
|
return 1;
|
|
}
|
|
|
|
phb->first_busno = be32_to_cpu(bus_range[0]);
|
|
phb->last_busno = be32_to_cpu(bus_range[1]);
|
|
|
|
return 0;
|
|
}
|
|
|
|
int rtas_setup_phb(struct pci_controller *phb)
|
|
{
|
|
struct device_node *dev = phb->dn;
|
|
|
|
if (is_python(dev))
|
|
python_countermeasures(dev);
|
|
|
|
if (phb_set_bus_ranges(dev, phb))
|
|
return 1;
|
|
|
|
phb->ops = &rtas_pci_ops;
|
|
phb->buid = get_phb_buid(dev);
|
|
|
|
return 0;
|
|
}
|