mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-23 09:19:51 +00:00
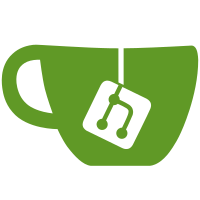
For powerpc64, redefine the ppc_inst type so both word and prefixed instructions can be represented. On powerpc32 the type will remain the same. Update places which had assumed instructions to be 4 bytes long. Signed-off-by: Jordan Niethe <jniethe5@gmail.com> Reviewed-by: Alistair Popple <alistair@popple.id.au> [mpe: Rework the get_user_inst() macros to be parameterised, and don't assign to the dest if an error occurred. Use CONFIG_PPC64 not __powerpc64__ in a few places. Address other comments from Christophe. Fix some sparse complaints.] Signed-off-by: Michael Ellerman <mpe@ellerman.id.au> Link: https://lore.kernel.org/r/20200506034050.24806-24-jniethe5@gmail.com
73 lines
1.4 KiB
C
73 lines
1.4 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* Copyright 2020, IBM Corporation.
|
|
*/
|
|
|
|
#include <linux/uaccess.h>
|
|
#include <asm/disassemble.h>
|
|
#include <asm/inst.h>
|
|
#include <asm/ppc-opcode.h>
|
|
|
|
#ifdef CONFIG_PPC64
|
|
int probe_user_read_inst(struct ppc_inst *inst,
|
|
struct ppc_inst __user *nip)
|
|
{
|
|
unsigned int val, suffix;
|
|
int err;
|
|
|
|
err = probe_user_read(&val, nip, sizeof(val));
|
|
if (err)
|
|
return err;
|
|
if (get_op(val) == OP_PREFIX) {
|
|
err = probe_user_read(&suffix, (void __user *)nip + 4, 4);
|
|
*inst = ppc_inst_prefix(val, suffix);
|
|
} else {
|
|
*inst = ppc_inst(val);
|
|
}
|
|
return err;
|
|
}
|
|
|
|
int probe_kernel_read_inst(struct ppc_inst *inst,
|
|
struct ppc_inst *src)
|
|
{
|
|
unsigned int val, suffix;
|
|
int err;
|
|
|
|
err = probe_kernel_read(&val, src, sizeof(val));
|
|
if (err)
|
|
return err;
|
|
if (get_op(val) == OP_PREFIX) {
|
|
err = probe_kernel_read(&suffix, (void *)src + 4, 4);
|
|
*inst = ppc_inst_prefix(val, suffix);
|
|
} else {
|
|
*inst = ppc_inst(val);
|
|
}
|
|
return err;
|
|
}
|
|
#else /* !CONFIG_PPC64 */
|
|
int probe_user_read_inst(struct ppc_inst *inst,
|
|
struct ppc_inst __user *nip)
|
|
{
|
|
unsigned int val;
|
|
int err;
|
|
|
|
err = probe_user_read(&val, nip, sizeof(val));
|
|
if (!err)
|
|
*inst = ppc_inst(val);
|
|
|
|
return err;
|
|
}
|
|
|
|
int probe_kernel_read_inst(struct ppc_inst *inst,
|
|
struct ppc_inst *src)
|
|
{
|
|
unsigned int val;
|
|
int err;
|
|
|
|
err = probe_kernel_read(&val, src, sizeof(val));
|
|
if (!err)
|
|
*inst = ppc_inst(val);
|
|
|
|
return err;
|
|
}
|
|
#endif /* CONFIG_PPC64 */
|