mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 08:58:07 +00:00
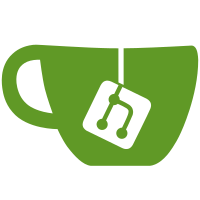
These helpers are only used for remapping the ISA I/O base. Replace the mapping side with a remap_isa_range helper in isa-bridge.c that hard codes all the known arguments, and just remove __iounmap_at in favour of open coding it in the only caller. Signed-off-by: Christoph Hellwig <hch@lst.de> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Acked-by: Peter Zijlstra (Intel) <peterz@infradead.org> Cc: Christian Borntraeger <borntraeger@de.ibm.com> Cc: Christophe Leroy <christophe.leroy@c-s.fr> Cc: Daniel Vetter <daniel.vetter@ffwll.ch> Cc: David Airlie <airlied@linux.ie> Cc: Gao Xiang <xiang@kernel.org> Cc: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Cc: Haiyang Zhang <haiyangz@microsoft.com> Cc: Johannes Weiner <hannes@cmpxchg.org> Cc: "K. Y. Srinivasan" <kys@microsoft.com> Cc: Laura Abbott <labbott@redhat.com> Cc: Mark Rutland <mark.rutland@arm.com> Cc: Michael Kelley <mikelley@microsoft.com> Cc: Minchan Kim <minchan@kernel.org> Cc: Nitin Gupta <ngupta@vflare.org> Cc: Robin Murphy <robin.murphy@arm.com> Cc: Sakari Ailus <sakari.ailus@linux.intel.com> Cc: Stephen Hemminger <sthemmin@microsoft.com> Cc: Sumit Semwal <sumit.semwal@linaro.org> Cc: Wei Liu <wei.liu@kernel.org> Cc: Benjamin Herrenschmidt <benh@kernel.crashing.org> Cc: Catalin Marinas <catalin.marinas@arm.com> Cc: Heiko Carstens <heiko.carstens@de.ibm.com> Cc: Paul Mackerras <paulus@ozlabs.org> Cc: Vasily Gorbik <gor@linux.ibm.com> Cc: Will Deacon <will@kernel.org> Link: http://lkml.kernel.org/r/20200414131348.444715-8-hch@lst.de Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
65 lines
1.6 KiB
C
65 lines
1.6 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
|
|
#include <linux/io.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/vmalloc.h>
|
|
|
|
void __iomem *__ioremap_caller(phys_addr_t addr, unsigned long size,
|
|
pgprot_t prot, void *caller)
|
|
{
|
|
phys_addr_t paligned, offset;
|
|
void __iomem *ret;
|
|
int err;
|
|
|
|
/* We don't support the 4K PFN hack with ioremap */
|
|
if (pgprot_val(prot) & H_PAGE_4K_PFN)
|
|
return NULL;
|
|
|
|
/*
|
|
* Choose an address to map it to. Once the vmalloc system is running,
|
|
* we use it. Before that, we map using addresses going up from
|
|
* ioremap_bot. vmalloc will use the addresses from IOREMAP_BASE
|
|
* through ioremap_bot.
|
|
*/
|
|
paligned = addr & PAGE_MASK;
|
|
offset = addr & ~PAGE_MASK;
|
|
size = PAGE_ALIGN(addr + size) - paligned;
|
|
|
|
if (size == 0 || paligned == 0)
|
|
return NULL;
|
|
|
|
if (slab_is_available())
|
|
return do_ioremap(paligned, offset, size, prot, caller);
|
|
|
|
pr_warn("ioremap() called early from %pS. Use early_ioremap() instead\n", caller);
|
|
|
|
err = early_ioremap_range(ioremap_bot, paligned, size, prot);
|
|
if (err)
|
|
return NULL;
|
|
|
|
ret = (void __iomem *)ioremap_bot + offset;
|
|
ioremap_bot += size;
|
|
|
|
return ret;
|
|
}
|
|
|
|
/*
|
|
* Unmap an IO region and remove it from vmalloc'd list.
|
|
* Access to IO memory should be serialized by driver.
|
|
*/
|
|
void iounmap(volatile void __iomem *token)
|
|
{
|
|
void *addr;
|
|
|
|
if (!slab_is_available())
|
|
return;
|
|
|
|
addr = (void *)((unsigned long __force)PCI_FIX_ADDR(token) & PAGE_MASK);
|
|
|
|
if ((unsigned long)addr < ioremap_bot) {
|
|
pr_warn("Attempt to iounmap early bolted mapping at 0x%p\n", addr);
|
|
return;
|
|
}
|
|
vunmap(addr);
|
|
}
|
|
EXPORT_SYMBOL(iounmap);
|