mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-19 17:11:03 +00:00
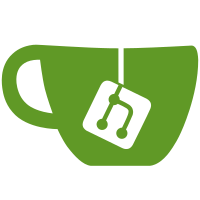
Syzbot reported two new paths to hit an internal WARNING using the
new virtio gso type VIRTIO_NET_HDR_GSO_UDP_L4.
RIP: 0010:skb_checksum_help+0x4a2/0x600 net/core/dev.c:3260
skb len=64521 gso_size=344
and
RIP: 0010:skb_warn_bad_offload+0x118/0x240 net/core/dev.c:3262
Older virtio types have historically had loose restrictions, leading
to many entirely impractical fuzzer generated packets causing
problems deep in the kernel stack. Ideally, we would have had strict
validation for all types from the start.
New virtio types can have tighter validation. Limit UDP GSO packets
inserted via virtio to the same limits imposed by the UDP_SEGMENT
socket interface:
1. must use checksum offload
2. checksum offload matches UDP header
3. no more segments than UDP_MAX_SEGMENTS
4. UDP GSO does not take modifier flags, notably SKB_GSO_TCP_ECN
Fixes: 860b7f27b8
("linux/virtio_net.h: Support USO offload in vnet header.")
Reported-by: syzbot+01cdbc31e9c0ae9b33ac@syzkaller.appspotmail.com
Closes: https://lore.kernel.org/netdev/0000000000005039270605eb0b7f@google.com/
Reported-by: syzbot+c99d835ff081ca30f986@syzkaller.appspotmail.com
Closes: https://lore.kernel.org/netdev/0000000000005426680605eb0b9f@google.com/
Signed-off-by: Willem de Bruijn <willemb@google.com>
Reviewed-by: Eric Dumazet <edumazet@google.com>
Acked-by: Jason Wang <jasowang@redhat.com>
Signed-off-by: David S. Miller <davem@davemloft.net>
232 lines
6.1 KiB
C
232 lines
6.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _LINUX_VIRTIO_NET_H
|
|
#define _LINUX_VIRTIO_NET_H
|
|
|
|
#include <linux/if_vlan.h>
|
|
#include <linux/udp.h>
|
|
#include <uapi/linux/tcp.h>
|
|
#include <uapi/linux/virtio_net.h>
|
|
|
|
static inline bool virtio_net_hdr_match_proto(__be16 protocol, __u8 gso_type)
|
|
{
|
|
switch (gso_type & ~VIRTIO_NET_HDR_GSO_ECN) {
|
|
case VIRTIO_NET_HDR_GSO_TCPV4:
|
|
return protocol == cpu_to_be16(ETH_P_IP);
|
|
case VIRTIO_NET_HDR_GSO_TCPV6:
|
|
return protocol == cpu_to_be16(ETH_P_IPV6);
|
|
case VIRTIO_NET_HDR_GSO_UDP:
|
|
case VIRTIO_NET_HDR_GSO_UDP_L4:
|
|
return protocol == cpu_to_be16(ETH_P_IP) ||
|
|
protocol == cpu_to_be16(ETH_P_IPV6);
|
|
default:
|
|
return false;
|
|
}
|
|
}
|
|
|
|
static inline int virtio_net_hdr_set_proto(struct sk_buff *skb,
|
|
const struct virtio_net_hdr *hdr)
|
|
{
|
|
if (skb->protocol)
|
|
return 0;
|
|
|
|
switch (hdr->gso_type & ~VIRTIO_NET_HDR_GSO_ECN) {
|
|
case VIRTIO_NET_HDR_GSO_TCPV4:
|
|
case VIRTIO_NET_HDR_GSO_UDP:
|
|
case VIRTIO_NET_HDR_GSO_UDP_L4:
|
|
skb->protocol = cpu_to_be16(ETH_P_IP);
|
|
break;
|
|
case VIRTIO_NET_HDR_GSO_TCPV6:
|
|
skb->protocol = cpu_to_be16(ETH_P_IPV6);
|
|
break;
|
|
default:
|
|
return -EINVAL;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static inline int virtio_net_hdr_to_skb(struct sk_buff *skb,
|
|
const struct virtio_net_hdr *hdr,
|
|
bool little_endian)
|
|
{
|
|
unsigned int gso_type = 0;
|
|
unsigned int thlen = 0;
|
|
unsigned int p_off = 0;
|
|
unsigned int ip_proto;
|
|
|
|
if (hdr->gso_type != VIRTIO_NET_HDR_GSO_NONE) {
|
|
switch (hdr->gso_type & ~VIRTIO_NET_HDR_GSO_ECN) {
|
|
case VIRTIO_NET_HDR_GSO_TCPV4:
|
|
gso_type = SKB_GSO_TCPV4;
|
|
ip_proto = IPPROTO_TCP;
|
|
thlen = sizeof(struct tcphdr);
|
|
break;
|
|
case VIRTIO_NET_HDR_GSO_TCPV6:
|
|
gso_type = SKB_GSO_TCPV6;
|
|
ip_proto = IPPROTO_TCP;
|
|
thlen = sizeof(struct tcphdr);
|
|
break;
|
|
case VIRTIO_NET_HDR_GSO_UDP:
|
|
gso_type = SKB_GSO_UDP;
|
|
ip_proto = IPPROTO_UDP;
|
|
thlen = sizeof(struct udphdr);
|
|
break;
|
|
case VIRTIO_NET_HDR_GSO_UDP_L4:
|
|
gso_type = SKB_GSO_UDP_L4;
|
|
ip_proto = IPPROTO_UDP;
|
|
thlen = sizeof(struct udphdr);
|
|
break;
|
|
default:
|
|
return -EINVAL;
|
|
}
|
|
|
|
if (hdr->gso_type & VIRTIO_NET_HDR_GSO_ECN)
|
|
gso_type |= SKB_GSO_TCP_ECN;
|
|
|
|
if (hdr->gso_size == 0)
|
|
return -EINVAL;
|
|
}
|
|
|
|
skb_reset_mac_header(skb);
|
|
|
|
if (hdr->flags & VIRTIO_NET_HDR_F_NEEDS_CSUM) {
|
|
u32 start = __virtio16_to_cpu(little_endian, hdr->csum_start);
|
|
u32 off = __virtio16_to_cpu(little_endian, hdr->csum_offset);
|
|
u32 needed = start + max_t(u32, thlen, off + sizeof(__sum16));
|
|
|
|
if (!pskb_may_pull(skb, needed))
|
|
return -EINVAL;
|
|
|
|
if (!skb_partial_csum_set(skb, start, off))
|
|
return -EINVAL;
|
|
|
|
p_off = skb_transport_offset(skb) + thlen;
|
|
if (!pskb_may_pull(skb, p_off))
|
|
return -EINVAL;
|
|
} else {
|
|
/* gso packets without NEEDS_CSUM do not set transport_offset.
|
|
* probe and drop if does not match one of the above types.
|
|
*/
|
|
if (gso_type && skb->network_header) {
|
|
struct flow_keys_basic keys;
|
|
|
|
if (!skb->protocol) {
|
|
__be16 protocol = dev_parse_header_protocol(skb);
|
|
|
|
if (!protocol)
|
|
virtio_net_hdr_set_proto(skb, hdr);
|
|
else if (!virtio_net_hdr_match_proto(protocol, hdr->gso_type))
|
|
return -EINVAL;
|
|
else
|
|
skb->protocol = protocol;
|
|
}
|
|
retry:
|
|
if (!skb_flow_dissect_flow_keys_basic(NULL, skb, &keys,
|
|
NULL, 0, 0, 0,
|
|
0)) {
|
|
/* UFO does not specify ipv4 or 6: try both */
|
|
if (gso_type & SKB_GSO_UDP &&
|
|
skb->protocol == htons(ETH_P_IP)) {
|
|
skb->protocol = htons(ETH_P_IPV6);
|
|
goto retry;
|
|
}
|
|
return -EINVAL;
|
|
}
|
|
|
|
p_off = keys.control.thoff + thlen;
|
|
if (!pskb_may_pull(skb, p_off) ||
|
|
keys.basic.ip_proto != ip_proto)
|
|
return -EINVAL;
|
|
|
|
skb_set_transport_header(skb, keys.control.thoff);
|
|
} else if (gso_type) {
|
|
p_off = thlen;
|
|
if (!pskb_may_pull(skb, p_off))
|
|
return -EINVAL;
|
|
}
|
|
}
|
|
|
|
if (hdr->gso_type != VIRTIO_NET_HDR_GSO_NONE) {
|
|
u16 gso_size = __virtio16_to_cpu(little_endian, hdr->gso_size);
|
|
unsigned int nh_off = p_off;
|
|
struct skb_shared_info *shinfo = skb_shinfo(skb);
|
|
|
|
switch (gso_type & ~SKB_GSO_TCP_ECN) {
|
|
case SKB_GSO_UDP:
|
|
/* UFO may not include transport header in gso_size. */
|
|
nh_off -= thlen;
|
|
break;
|
|
case SKB_GSO_UDP_L4:
|
|
if (!(hdr->flags & VIRTIO_NET_HDR_F_NEEDS_CSUM))
|
|
return -EINVAL;
|
|
if (skb->csum_offset != offsetof(struct udphdr, check))
|
|
return -EINVAL;
|
|
if (skb->len - p_off > gso_size * UDP_MAX_SEGMENTS)
|
|
return -EINVAL;
|
|
if (gso_type != SKB_GSO_UDP_L4)
|
|
return -EINVAL;
|
|
break;
|
|
}
|
|
|
|
/* Kernel has a special handling for GSO_BY_FRAGS. */
|
|
if (gso_size == GSO_BY_FRAGS)
|
|
return -EINVAL;
|
|
|
|
/* Too small packets are not really GSO ones. */
|
|
if (skb->len - nh_off > gso_size) {
|
|
shinfo->gso_size = gso_size;
|
|
shinfo->gso_type = gso_type;
|
|
|
|
/* Header must be checked, and gso_segs computed. */
|
|
shinfo->gso_type |= SKB_GSO_DODGY;
|
|
shinfo->gso_segs = 0;
|
|
}
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static inline int virtio_net_hdr_from_skb(const struct sk_buff *skb,
|
|
struct virtio_net_hdr *hdr,
|
|
bool little_endian,
|
|
bool has_data_valid,
|
|
int vlan_hlen)
|
|
{
|
|
memset(hdr, 0, sizeof(*hdr)); /* no info leak */
|
|
|
|
if (skb_is_gso(skb)) {
|
|
struct skb_shared_info *sinfo = skb_shinfo(skb);
|
|
|
|
/* This is a hint as to how much should be linear. */
|
|
hdr->hdr_len = __cpu_to_virtio16(little_endian,
|
|
skb_headlen(skb));
|
|
hdr->gso_size = __cpu_to_virtio16(little_endian,
|
|
sinfo->gso_size);
|
|
if (sinfo->gso_type & SKB_GSO_TCPV4)
|
|
hdr->gso_type = VIRTIO_NET_HDR_GSO_TCPV4;
|
|
else if (sinfo->gso_type & SKB_GSO_TCPV6)
|
|
hdr->gso_type = VIRTIO_NET_HDR_GSO_TCPV6;
|
|
else if (sinfo->gso_type & SKB_GSO_UDP_L4)
|
|
hdr->gso_type = VIRTIO_NET_HDR_GSO_UDP_L4;
|
|
else
|
|
return -EINVAL;
|
|
if (sinfo->gso_type & SKB_GSO_TCP_ECN)
|
|
hdr->gso_type |= VIRTIO_NET_HDR_GSO_ECN;
|
|
} else
|
|
hdr->gso_type = VIRTIO_NET_HDR_GSO_NONE;
|
|
|
|
if (skb->ip_summed == CHECKSUM_PARTIAL) {
|
|
hdr->flags = VIRTIO_NET_HDR_F_NEEDS_CSUM;
|
|
hdr->csum_start = __cpu_to_virtio16(little_endian,
|
|
skb_checksum_start_offset(skb) + vlan_hlen);
|
|
hdr->csum_offset = __cpu_to_virtio16(little_endian,
|
|
skb->csum_offset);
|
|
} else if (has_data_valid &&
|
|
skb->ip_summed == CHECKSUM_UNNECESSARY) {
|
|
hdr->flags = VIRTIO_NET_HDR_F_DATA_VALID;
|
|
} /* else everything is zero */
|
|
|
|
return 0;
|
|
}
|
|
|
|
#endif /* _LINUX_VIRTIO_NET_H */
|