mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-13 14:14:37 +00:00
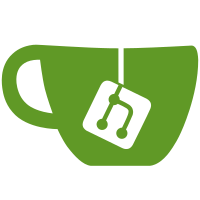
Add slot and channel count programming to hdmi_chmap object and move the chmap_ops to core. Use register_chmap_ops API to register for default ops. Override specific chmap ops in the driver. Signed-off-by: Subhransu S. Prusty <subhransu.s.prusty@intel.com> Signed-off-by: Vinod Koul <vinod.koul@intel.com> Signed-off-by: Takashi Iwai <tiwai@suse.de>
49 lines
1.3 KiB
C
49 lines
1.3 KiB
C
/*
|
|
* HDMI Channel map support helpers
|
|
*/
|
|
|
|
#include <sound/hda_chmap.h>
|
|
|
|
static int hdmi_pin_set_slot_channel(struct hdac_device *codec,
|
|
hda_nid_t pin_nid, int asp_slot, int channel)
|
|
{
|
|
return snd_hdac_codec_write(codec, pin_nid, 0,
|
|
AC_VERB_SET_HDMI_CHAN_SLOT,
|
|
(channel << 4) | asp_slot);
|
|
}
|
|
|
|
static int hdmi_pin_get_slot_channel(struct hdac_device *codec,
|
|
hda_nid_t pin_nid, int asp_slot)
|
|
{
|
|
return (snd_hdac_codec_read(codec, pin_nid, 0,
|
|
AC_VERB_GET_HDMI_CHAN_SLOT,
|
|
asp_slot) & 0xf0) >> 4;
|
|
}
|
|
|
|
static int hdmi_get_channel_count(struct hdac_device *codec, hda_nid_t cvt_nid)
|
|
{
|
|
return 1 + snd_hdac_codec_read(codec, cvt_nid, 0,
|
|
AC_VERB_GET_CVT_CHAN_COUNT, 0);
|
|
}
|
|
|
|
static void hdmi_set_channel_count(struct hdac_device *codec,
|
|
hda_nid_t cvt_nid, int chs)
|
|
{
|
|
if (chs != hdmi_get_channel_count(codec, cvt_nid))
|
|
snd_hdac_codec_write(codec, cvt_nid, 0,
|
|
AC_VERB_SET_CVT_CHAN_COUNT, chs - 1);
|
|
}
|
|
|
|
static const struct hdac_chmap_ops chmap_ops = {
|
|
.pin_get_slot_channel = hdmi_pin_get_slot_channel,
|
|
.pin_set_slot_channel = hdmi_pin_set_slot_channel,
|
|
.set_channel_count = hdmi_set_channel_count,
|
|
};
|
|
|
|
void snd_hdac_register_chmap_ops(struct hdac_device *hdac,
|
|
struct hdac_chmap *chmap)
|
|
{
|
|
chmap->ops = chmap_ops;
|
|
chmap->hdac = hdac;
|
|
}
|
|
EXPORT_SYMBOL_GPL(snd_hdac_register_chmap_ops);
|