mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-03 15:47:36 +00:00
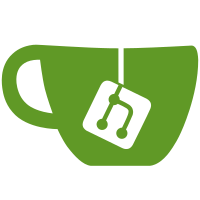
Currently most platforms define pmd_pgtable() as pmd_page() duplicating the same code all over. Instead just define a default value i.e pmd_page() for pmd_pgtable() and let platforms override when required via <asm/pgtable.h>. All the existing platform that override pmd_pgtable() have been moved into their respective <asm/pgtable.h> header in order to precede before the new generic definition. This makes it much cleaner with reduced code. Link: https://lkml.kernel.org/r/1623646133-20306-1-git-send-email-anshuman.khandual@arm.com Signed-off-by: Anshuman Khandual <anshuman.khandual@arm.com> Acked-by: Geert Uytterhoeven <geert@linux-m68k.org> Acked-by: Mike Rapoport <rppt@linux.ibm.com> Cc: Nick Hu <nickhu@andestech.com> Cc: Richard Henderson <rth@twiddle.net> Cc: Vineet Gupta <vgupta@synopsys.com> Cc: Catalin Marinas <catalin.marinas@arm.com> Cc: Will Deacon <will@kernel.org> Cc: Guo Ren <guoren@kernel.org> Cc: Brian Cain <bcain@codeaurora.org> Cc: Geert Uytterhoeven <geert@linux-m68k.org> Cc: Michal Simek <monstr@monstr.eu> Cc: Thomas Bogendoerfer <tsbogend@alpha.franken.de> Cc: Ley Foon Tan <ley.foon.tan@intel.com> Cc: Jonas Bonn <jonas@southpole.se> Cc: Stefan Kristiansson <stefan.kristiansson@saunalahti.fi> Cc: Stafford Horne <shorne@gmail.com> Cc: "James E.J. Bottomley" <James.Bottomley@HansenPartnership.com> Cc: Michael Ellerman <mpe@ellerman.id.au> Cc: Christophe Leroy <christophe.leroy@csgroup.eu> Cc: Paul Walmsley <paul.walmsley@sifive.com> Cc: Palmer Dabbelt <palmer@dabbelt.com> Cc: Heiko Carstens <hca@linux.ibm.com> Cc: Yoshinori Sato <ysato@users.sourceforge.jp> Cc: "David S. Miller" <davem@davemloft.net> Cc: Jeff Dike <jdike@addtoit.com> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: Chris Zankel <chris@zankel.net> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
77 lines
1.8 KiB
C
77 lines
1.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _SPARC_PGALLOC_H
|
|
#define _SPARC_PGALLOC_H
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/pgtable.h>
|
|
|
|
#include <asm/pgtsrmmu.h>
|
|
#include <asm/vaddrs.h>
|
|
#include <asm/page.h>
|
|
|
|
struct page;
|
|
|
|
void *srmmu_get_nocache(int size, int align);
|
|
void srmmu_free_nocache(void *addr, int size);
|
|
|
|
extern struct resource sparc_iomap;
|
|
|
|
pgd_t *get_pgd_fast(void);
|
|
static inline void free_pgd_fast(pgd_t *pgd)
|
|
{
|
|
srmmu_free_nocache(pgd, SRMMU_PGD_TABLE_SIZE);
|
|
}
|
|
|
|
#define pgd_free(mm, pgd) free_pgd_fast(pgd)
|
|
#define pgd_alloc(mm) get_pgd_fast()
|
|
|
|
static inline void pud_set(pud_t * pudp, pmd_t * pmdp)
|
|
{
|
|
unsigned long pa = __nocache_pa(pmdp);
|
|
|
|
set_pte((pte_t *)pudp, __pte((SRMMU_ET_PTD | (pa >> 4))));
|
|
}
|
|
|
|
#define pud_populate(MM, PGD, PMD) pud_set(PGD, PMD)
|
|
|
|
static inline pmd_t *pmd_alloc_one(struct mm_struct *mm,
|
|
unsigned long address)
|
|
{
|
|
return srmmu_get_nocache(SRMMU_PMD_TABLE_SIZE,
|
|
SRMMU_PMD_TABLE_SIZE);
|
|
}
|
|
|
|
static inline void free_pmd_fast(pmd_t * pmd)
|
|
{
|
|
srmmu_free_nocache(pmd, SRMMU_PMD_TABLE_SIZE);
|
|
}
|
|
|
|
#define pmd_free(mm, pmd) free_pmd_fast(pmd)
|
|
#define __pmd_free_tlb(tlb, pmd, addr) pmd_free((tlb)->mm, pmd)
|
|
|
|
#define pmd_populate(mm, pmd, pte) pmd_set(pmd, pte)
|
|
|
|
void pmd_set(pmd_t *pmdp, pte_t *ptep);
|
|
#define pmd_populate_kernel pmd_populate
|
|
|
|
pgtable_t pte_alloc_one(struct mm_struct *mm);
|
|
|
|
static inline pte_t *pte_alloc_one_kernel(struct mm_struct *mm)
|
|
{
|
|
return srmmu_get_nocache(SRMMU_PTE_TABLE_SIZE,
|
|
SRMMU_PTE_TABLE_SIZE);
|
|
}
|
|
|
|
|
|
static inline void free_pte_fast(pte_t *pte)
|
|
{
|
|
srmmu_free_nocache(pte, SRMMU_PTE_TABLE_SIZE);
|
|
}
|
|
|
|
#define pte_free_kernel(mm, pte) free_pte_fast(pte)
|
|
|
|
void pte_free(struct mm_struct * mm, pgtable_t pte);
|
|
#define __pte_free_tlb(tlb, pte, addr) pte_free((tlb)->mm, pte)
|
|
|
|
#endif /* _SPARC_PGALLOC_H */
|