mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 13:55:32 +00:00
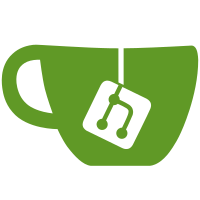
The dtpm table is an array of pointers, that forces the user of the table to define initdata along with the declaration of the table entry. It is more efficient to create an array of dtpm structure, so the declaration of the table entry can be done by initializing the different fields. Signed-off-by: Daniel Lezcano <daniel.lezcano@linaro.org> Reviewed-by: Lukasz Luba <lukasz.luba@arm.com> Link: https://lore.kernel.org/r/20210312130411.29833-3-daniel.lezcano@linaro.org
76 lines
1.6 KiB
C
76 lines
1.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* Copyright (C) 2020 Linaro Ltd
|
|
*
|
|
* Author: Daniel Lezcano <daniel.lezcano@linaro.org>
|
|
*/
|
|
#ifndef ___DTPM_H__
|
|
#define ___DTPM_H__
|
|
|
|
#include <linux/powercap.h>
|
|
|
|
#define MAX_DTPM_DESCR 8
|
|
#define MAX_DTPM_CONSTRAINTS 1
|
|
|
|
struct dtpm {
|
|
struct powercap_zone zone;
|
|
struct dtpm *parent;
|
|
struct list_head sibling;
|
|
struct list_head children;
|
|
struct dtpm_ops *ops;
|
|
unsigned long flags;
|
|
u64 power_limit;
|
|
u64 power_max;
|
|
u64 power_min;
|
|
int weight;
|
|
void *private;
|
|
};
|
|
|
|
struct dtpm_ops {
|
|
u64 (*set_power_uw)(struct dtpm *, u64);
|
|
u64 (*get_power_uw)(struct dtpm *);
|
|
int (*update_power_uw)(struct dtpm *);
|
|
void (*release)(struct dtpm *);
|
|
};
|
|
|
|
typedef int (*dtpm_init_t)(void);
|
|
|
|
struct dtpm_descr {
|
|
dtpm_init_t init;
|
|
};
|
|
|
|
/* Init section thermal table */
|
|
extern struct dtpm_descr __dtpm_table[];
|
|
extern struct dtpm_descr __dtpm_table_end[];
|
|
|
|
#define DTPM_TABLE_ENTRY(name, __init) \
|
|
static struct dtpm_descr __dtpm_table_entry_##name \
|
|
__used __section("__dtpm_table") = { \
|
|
.init = __init, \
|
|
}
|
|
|
|
#define DTPM_DECLARE(name, init) DTPM_TABLE_ENTRY(name, init)
|
|
|
|
#define for_each_dtpm_table(__dtpm) \
|
|
for (__dtpm = __dtpm_table; \
|
|
__dtpm < __dtpm_table_end; \
|
|
__dtpm++)
|
|
|
|
static inline struct dtpm *to_dtpm(struct powercap_zone *zone)
|
|
{
|
|
return container_of(zone, struct dtpm, zone);
|
|
}
|
|
|
|
int dtpm_update_power(struct dtpm *dtpm);
|
|
|
|
int dtpm_release_zone(struct powercap_zone *pcz);
|
|
|
|
struct dtpm *dtpm_alloc(struct dtpm_ops *ops);
|
|
|
|
void dtpm_unregister(struct dtpm *dtpm);
|
|
|
|
int dtpm_register(const char *name, struct dtpm *dtpm, struct dtpm *parent);
|
|
|
|
int dtpm_register_cpu(struct dtpm *parent);
|
|
|
|
#endif
|