mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 13:55:32 +00:00
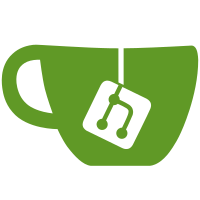
Clean up remaining headers that are specific to liblockdep but lived in
the shared header directory. These are all unused after the liblockdep
code was removed in commit 7246f4dcac
("tools/lib/lockdep: drop
liblockdep").
Note that there are still headers that were originally created for
liblockdep, that still have liblockdep references, but they are used by
other tools/ code at this point.
Signed-off-by: Sasha Levin <sashal@kernel.org>
Cc: Ingo Molnar <mingo@kernel.org>
Cc: Peter Zijlstra <peterz@infradead.org>
Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
40 lines
1.2 KiB
C
40 lines
1.2 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef __LINUX_SPINLOCK_H_
|
|
#define __LINUX_SPINLOCK_H_
|
|
|
|
#include <pthread.h>
|
|
#include <stdbool.h>
|
|
|
|
#define spinlock_t pthread_mutex_t
|
|
#define DEFINE_SPINLOCK(x) pthread_mutex_t x = PTHREAD_MUTEX_INITIALIZER
|
|
#define __SPIN_LOCK_UNLOCKED(x) (pthread_mutex_t)PTHREAD_MUTEX_INITIALIZER
|
|
#define spin_lock_init(x) pthread_mutex_init(x, NULL)
|
|
|
|
#define spin_lock(x) pthread_mutex_lock(x)
|
|
#define spin_unlock(x) pthread_mutex_unlock(x)
|
|
#define spin_lock_bh(x) pthread_mutex_lock(x)
|
|
#define spin_unlock_bh(x) pthread_mutex_unlock(x)
|
|
#define spin_lock_irq(x) pthread_mutex_lock(x)
|
|
#define spin_unlock_irq(x) pthread_mutex_unlock(x)
|
|
#define spin_lock_irqsave(x, f) (void)f, pthread_mutex_lock(x)
|
|
#define spin_unlock_irqrestore(x, f) (void)f, pthread_mutex_unlock(x)
|
|
|
|
#define arch_spinlock_t pthread_mutex_t
|
|
#define __ARCH_SPIN_LOCK_UNLOCKED PTHREAD_MUTEX_INITIALIZER
|
|
|
|
static inline void arch_spin_lock(arch_spinlock_t *mutex)
|
|
{
|
|
pthread_mutex_lock(mutex);
|
|
}
|
|
|
|
static inline void arch_spin_unlock(arch_spinlock_t *mutex)
|
|
{
|
|
pthread_mutex_unlock(mutex);
|
|
}
|
|
|
|
static inline bool arch_spin_is_locked(arch_spinlock_t *mutex)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
#endif
|