mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
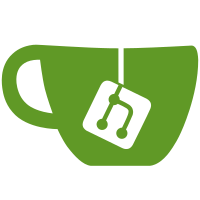
Any system call that takes a pointer argument on s390 requires a wrapper function to do a 31-to-64 zero-extension, these are currently generated in arch/s390/kernel/compat_wrapper.c. On arm64 and x86, we already generate similar wrappers for all system calls in the place of their definition, just for a different purpose (they load the arguments from pt_regs). We can do the same thing here, by adding an asm/syscall_wrapper.h file with a copy of all the relevant macros to override the generic version. Besides the addition of the compat entry point, these also rename the entry points with a __s390_ or __s390x_ prefix, similar to what we do on arm64 and x86. This in turn requires renaming a few things, and adding a proper ni_syscall() entry point. In order to still compile system call definitions that pass an loff_t argument, the __SC_COMPAT_CAST() macro checks for that and forces an -ENOSYS error, which was the best I could come up with. Those functions must obviously not get called from user space, but instead require hand-written compat_sys_*() handlers, which fortunately already exist. Link: https://lore.kernel.org/lkml/20190116131527.2071570-5-arnd@arndb.de Signed-off-by: Arnd Bergmann <arnd@arndb.de> Reviewed-by: Heiko Carstens <heiko.carstens@de.ibm.com> [heiko.carstens@de.ibm.com: compile fix for !CONFIG_COMPAT] Signed-off-by: Heiko Carstens <heiko.carstens@de.ibm.com> Signed-off-by: Martin Schwidefsky <schwidefsky@de.ibm.com>
102 lines
2.6 KiB
C
102 lines
2.6 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* S390 version
|
|
* Copyright IBM Corp. 1999, 2000
|
|
* Author(s): Martin Schwidefsky (schwidefsky@de.ibm.com),
|
|
* Thomas Spatzier (tspat@de.ibm.com)
|
|
*
|
|
* Derived from "arch/i386/kernel/sys_i386.c"
|
|
*
|
|
* This file contains various random system calls that
|
|
* have a non-standard calling sequence on the Linux/s390
|
|
* platform.
|
|
*/
|
|
|
|
#include <linux/errno.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/smp.h>
|
|
#include <linux/sem.h>
|
|
#include <linux/msg.h>
|
|
#include <linux/shm.h>
|
|
#include <linux/stat.h>
|
|
#include <linux/syscalls.h>
|
|
#include <linux/mman.h>
|
|
#include <linux/file.h>
|
|
#include <linux/utsname.h>
|
|
#include <linux/personality.h>
|
|
#include <linux/unistd.h>
|
|
#include <linux/ipc.h>
|
|
#include <linux/uaccess.h>
|
|
#include "entry.h"
|
|
|
|
/*
|
|
* Perform the mmap() system call. Linux for S/390 isn't able to handle more
|
|
* than 5 system call parameters, so this system call uses a memory block
|
|
* for parameter passing.
|
|
*/
|
|
|
|
struct s390_mmap_arg_struct {
|
|
unsigned long addr;
|
|
unsigned long len;
|
|
unsigned long prot;
|
|
unsigned long flags;
|
|
unsigned long fd;
|
|
unsigned long offset;
|
|
};
|
|
|
|
SYSCALL_DEFINE1(mmap2, struct s390_mmap_arg_struct __user *, arg)
|
|
{
|
|
struct s390_mmap_arg_struct a;
|
|
int error = -EFAULT;
|
|
|
|
if (copy_from_user(&a, arg, sizeof(a)))
|
|
goto out;
|
|
error = ksys_mmap_pgoff(a.addr, a.len, a.prot, a.flags, a.fd, a.offset);
|
|
out:
|
|
return error;
|
|
}
|
|
|
|
#ifdef CONFIG_SYSVIPC
|
|
/*
|
|
* sys_ipc() is the de-multiplexer for the SysV IPC calls.
|
|
*/
|
|
SYSCALL_DEFINE5(s390_ipc, uint, call, int, first, unsigned long, second,
|
|
unsigned long, third, void __user *, ptr)
|
|
{
|
|
if (call >> 16)
|
|
return -EINVAL;
|
|
/* The s390 sys_ipc variant has only five parameters instead of six
|
|
* like the generic variant. The only difference is the handling of
|
|
* the SEMTIMEDOP subcall where on s390 the third parameter is used
|
|
* as a pointer to a struct timespec where the generic variant uses
|
|
* the fifth parameter.
|
|
* Therefore we can call the generic variant by simply passing the
|
|
* third parameter also as fifth parameter.
|
|
*/
|
|
return ksys_ipc(call, first, second, third, ptr, third);
|
|
}
|
|
#endif /* CONFIG_SYSVIPC */
|
|
|
|
SYSCALL_DEFINE1(s390_personality, unsigned int, personality)
|
|
{
|
|
unsigned int ret = current->personality;
|
|
|
|
if (personality(current->personality) == PER_LINUX32 &&
|
|
personality(personality) == PER_LINUX)
|
|
personality |= PER_LINUX32;
|
|
|
|
if (personality != 0xffffffff)
|
|
set_personality(personality);
|
|
|
|
if (personality(ret) == PER_LINUX32)
|
|
ret &= ~PER_LINUX32;
|
|
|
|
return ret;
|
|
}
|
|
|
|
SYSCALL_DEFINE0(ni_syscall)
|
|
{
|
|
return -ENOSYS;
|
|
}
|