mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 13:55:32 +00:00
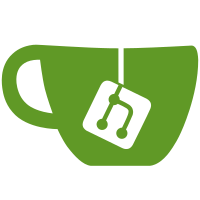
Some metric groups have metrics that don't have fully overlapping events, meaning that the group's events become unique event groups that may need to multiplex with each other. This can be particularly unfortunate when the groups wouldn't need to multiplex because there are sufficient hardware counters. Add a flag so that if recording a metric group then the metrics within the group needn't use groups for their events. The flag is added to Intel TopdownL1 and TopdownL2 metrics. Signed-off-by: Ian Rogers <irogers@google.com> Tested-by: Kan Liang <kan.liang@linux.intel.com> Cc: Adrian Hunter <adrian.hunter@intel.com> Cc: Ahmad Yasin <ahmad.yasin@intel.com> Cc: Alexander Shishkin <alexander.shishkin@linux.intel.com> Cc: Andi Kleen <ak@linux.intel.com> Cc: Athira Rajeev <atrajeev@linux.vnet.ibm.com> Cc: Caleb Biggers <caleb.biggers@intel.com> Cc: Edward Baker <edward.baker@intel.com> Cc: Florian Fischer <florian.fischer@muhq.space> Cc: Ingo Molnar <mingo@redhat.com> Cc: James Clark <james.clark@arm.com> Cc: Jiri Olsa <jolsa@kernel.org> Cc: John Garry <john.g.garry@oracle.com> Cc: Kajol Jain <kjain@linux.ibm.com> Cc: Kang Minchul <tegongkang@gmail.com> Cc: Leo Yan <leo.yan@linaro.org> Cc: Mark Rutland <mark.rutland@arm.com> Cc: Namhyung Kim <namhyung@kernel.org> Cc: Perry Taylor <perry.taylor@intel.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Ravi Bangoria <ravi.bangoria@amd.com> Cc: Rob Herring <robh@kernel.org> Cc: Samantha Alt <samantha.alt@intel.com> Cc: Stephane Eranian <eranian@google.com> Cc: Sumanth Korikkar <sumanthk@linux.ibm.com> Cc: Suzuki Poulouse <suzuki.poulose@arm.com> Cc: Thomas Richter <tmricht@linux.ibm.com> Cc: Tiezhu Yang <yangtiezhu@loongson.cn> Cc: Weilin Wang <weilin.wang@intel.com> Cc: Xing Zhengjun <zhengjun.xing@linux.intel.com> Cc: Yang Jihong <yangjihong1@huawei.com> Link: https://lore.kernel.org/r/20230502223851.2234828-4-irogers@google.com Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
95 lines
2.7 KiB
C
95 lines
2.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef PMU_EVENTS_H
|
|
#define PMU_EVENTS_H
|
|
|
|
#include <stdbool.h>
|
|
|
|
struct perf_pmu;
|
|
|
|
enum aggr_mode_class {
|
|
PerChip = 1,
|
|
PerCore
|
|
};
|
|
|
|
/**
|
|
* enum metric_event_groups - How events within a pmu_metric should be grouped.
|
|
*/
|
|
enum metric_event_groups {
|
|
/**
|
|
* @MetricGroupEvents: Default, group events within the metric.
|
|
*/
|
|
MetricGroupEvents = 0,
|
|
/**
|
|
* @MetricNoGroupEvents: Don't group events for the metric.
|
|
*/
|
|
MetricNoGroupEvents = 1,
|
|
/**
|
|
* @MetricNoGroupEventsNmi: Don't group events for the metric if the NMI
|
|
* watchdog is enabled.
|
|
*/
|
|
MetricNoGroupEventsNmi = 2,
|
|
/**
|
|
* @MetricNoGroupEventsSmt: Don't group events for the metric if SMT is
|
|
* enabled.
|
|
*/
|
|
MetricNoGroupEventsSmt = 3,
|
|
};
|
|
/*
|
|
* Describe each PMU event. Each CPU has a table of PMU events.
|
|
*/
|
|
struct pmu_event {
|
|
const char *name;
|
|
const char *compat;
|
|
const char *event;
|
|
const char *desc;
|
|
const char *topic;
|
|
const char *long_desc;
|
|
const char *pmu;
|
|
const char *unit;
|
|
bool perpkg;
|
|
bool deprecated;
|
|
};
|
|
|
|
struct pmu_metric {
|
|
const char *metric_name;
|
|
const char *metric_group;
|
|
const char *metric_expr;
|
|
const char *metric_threshold;
|
|
const char *unit;
|
|
const char *compat;
|
|
const char *desc;
|
|
const char *long_desc;
|
|
const char *metricgroup_no_group;
|
|
enum aggr_mode_class aggr_mode;
|
|
enum metric_event_groups event_grouping;
|
|
};
|
|
|
|
struct pmu_events_table;
|
|
struct pmu_metrics_table;
|
|
|
|
typedef int (*pmu_event_iter_fn)(const struct pmu_event *pe,
|
|
const struct pmu_events_table *table,
|
|
void *data);
|
|
|
|
typedef int (*pmu_metric_iter_fn)(const struct pmu_metric *pm,
|
|
const struct pmu_metrics_table *table,
|
|
void *data);
|
|
|
|
int pmu_events_table_for_each_event(const struct pmu_events_table *table, pmu_event_iter_fn fn,
|
|
void *data);
|
|
int pmu_metrics_table_for_each_metric(const struct pmu_metrics_table *table, pmu_metric_iter_fn fn,
|
|
void *data);
|
|
|
|
const struct pmu_events_table *perf_pmu__find_events_table(struct perf_pmu *pmu);
|
|
const struct pmu_metrics_table *perf_pmu__find_metrics_table(struct perf_pmu *pmu);
|
|
const struct pmu_events_table *find_core_events_table(const char *arch, const char *cpuid);
|
|
const struct pmu_metrics_table *find_core_metrics_table(const char *arch, const char *cpuid);
|
|
int pmu_for_each_core_event(pmu_event_iter_fn fn, void *data);
|
|
int pmu_for_each_core_metric(pmu_metric_iter_fn fn, void *data);
|
|
|
|
const struct pmu_events_table *find_sys_events_table(const char *name);
|
|
const struct pmu_metrics_table *find_sys_metrics_table(const char *name);
|
|
int pmu_for_each_sys_event(pmu_event_iter_fn fn, void *data);
|
|
int pmu_for_each_sys_metric(pmu_metric_iter_fn fn, void *data);
|
|
|
|
#endif
|