mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-03 23:58:05 +00:00
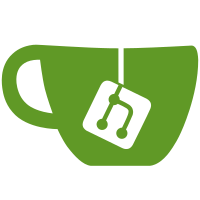
This provides valuable information for tracing performance problems. Since this change alters the interface for the python scripts, also adjust the script generation and the provided scripts. Signed-off-by: Joseph Schuchart <joseph.schuchart@tu-dresden.de> Acked-by: Thomas Ilsche <thomas.ilsche@tu-dresden.de> Cc: Ingo Molnar <mingo@redhat.com> Cc: Jiri Olsa <jolsa@redhat.com> Cc: Namhyung Kim <namhyung@gmail.com> Cc: Paul Mackerras <paulus@samba.org> Cc: Peter Zijlstra <a.p.zijlstra@chello.nl> Cc: Thomas Ilsche <thomas.ilsche@tu-dresden.de> Link: http://lkml.kernel.org/r/53BE7E1B.10503@tu-dresden.de Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
75 lines
1.7 KiB
Python
Executable file
75 lines
1.7 KiB
Python
Executable file
# Monitor the system for dropped packets and proudce a report of drop locations and counts
|
|
|
|
import os
|
|
import sys
|
|
|
|
sys.path.append(os.environ['PERF_EXEC_PATH'] + \
|
|
'/scripts/python/Perf-Trace-Util/lib/Perf/Trace')
|
|
|
|
from perf_trace_context import *
|
|
from Core import *
|
|
from Util import *
|
|
|
|
drop_log = {}
|
|
kallsyms = []
|
|
|
|
def get_kallsyms_table():
|
|
global kallsyms
|
|
|
|
try:
|
|
f = open("/proc/kallsyms", "r")
|
|
except:
|
|
return
|
|
|
|
for line in f:
|
|
loc = int(line.split()[0], 16)
|
|
name = line.split()[2]
|
|
kallsyms.append((loc, name))
|
|
kallsyms.sort()
|
|
|
|
def get_sym(sloc):
|
|
loc = int(sloc)
|
|
|
|
# Invariant: kallsyms[i][0] <= loc for all 0 <= i <= start
|
|
# kallsyms[i][0] > loc for all end <= i < len(kallsyms)
|
|
start, end = -1, len(kallsyms)
|
|
while end != start + 1:
|
|
pivot = (start + end) // 2
|
|
if loc < kallsyms[pivot][0]:
|
|
end = pivot
|
|
else:
|
|
start = pivot
|
|
|
|
# Now (start == -1 or kallsyms[start][0] <= loc)
|
|
# and (start == len(kallsyms) - 1 or loc < kallsyms[start + 1][0])
|
|
if start >= 0:
|
|
symloc, name = kallsyms[start]
|
|
return (name, loc - symloc)
|
|
else:
|
|
return (None, 0)
|
|
|
|
def print_drop_table():
|
|
print "%25s %25s %25s" % ("LOCATION", "OFFSET", "COUNT")
|
|
for i in drop_log.keys():
|
|
(sym, off) = get_sym(i)
|
|
if sym == None:
|
|
sym = i
|
|
print "%25s %25s %25s" % (sym, off, drop_log[i])
|
|
|
|
|
|
def trace_begin():
|
|
print "Starting trace (Ctrl-C to dump results)"
|
|
|
|
def trace_end():
|
|
print "Gathering kallsyms data"
|
|
get_kallsyms_table()
|
|
print_drop_table()
|
|
|
|
# called from perf, when it finds a correspoinding event
|
|
def skb__kfree_skb(name, context, cpu, sec, nsec, pid, comm, callchain,
|
|
skbaddr, location, protocol):
|
|
slocation = str(location)
|
|
try:
|
|
drop_log[slocation] = drop_log[slocation] + 1
|
|
except:
|
|
drop_log[slocation] = 1
|