mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
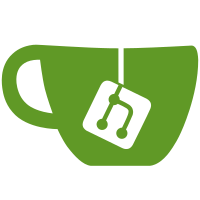
Many drivers (21 to be exact) create connectors that are always connected (for instance to an LVDS or DSI panel). Instead of forcing them to implement a dummy .detect() handler, make the callback optional and consider the connector as always connected in that case. Reviewed-by: Alex Deucher <alexander.deucher@amd.com> Acked-by: Maxime Ripard <maxime.ripard@free-electrons.com> Acked-by: Jyri Sarha <jsarha@ti.com> Acked-by: Jani Nikula <jani.nikula@intel.com> Acked-by: Philipp Zabel <p.zabel@pengutronix.de> Acked-by: Vincent Abriou <vincent.abriou@st.com> Acked-by: Alexey Brodkin <abrodkin@synopsys.com> Signed-off-by: Laurent Pinchart <laurent.pinchart+renesas@ideasonboard.com> [seanpaul fixed small conflict in rcar-du/rcar_du_lvdscon.c] Signed-off-by: Sean Paul <seanpaul@chromium.org>
121 lines
3.2 KiB
C
121 lines
3.2 KiB
C
/*
|
|
* ARC PGU DRM driver.
|
|
*
|
|
* Copyright (C) 2016 Synopsys, Inc. (www.synopsys.com)
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
*/
|
|
|
|
#include <drm/drm_crtc_helper.h>
|
|
#include <drm/drm_encoder_slave.h>
|
|
#include <drm/drm_atomic_helper.h>
|
|
|
|
#include "arcpgu.h"
|
|
|
|
#define XRES_DEF 640
|
|
#define YRES_DEF 480
|
|
|
|
#define XRES_MAX 8192
|
|
#define YRES_MAX 8192
|
|
|
|
|
|
struct arcpgu_drm_connector {
|
|
struct drm_connector connector;
|
|
struct drm_encoder_slave *encoder_slave;
|
|
};
|
|
|
|
static int arcpgu_drm_connector_get_modes(struct drm_connector *connector)
|
|
{
|
|
int count;
|
|
|
|
count = drm_add_modes_noedid(connector, XRES_MAX, YRES_MAX);
|
|
drm_set_preferred_mode(connector, XRES_DEF, YRES_DEF);
|
|
return count;
|
|
}
|
|
|
|
static void arcpgu_drm_connector_destroy(struct drm_connector *connector)
|
|
{
|
|
drm_connector_unregister(connector);
|
|
drm_connector_cleanup(connector);
|
|
}
|
|
|
|
static const struct drm_connector_helper_funcs
|
|
arcpgu_drm_connector_helper_funcs = {
|
|
.get_modes = arcpgu_drm_connector_get_modes,
|
|
};
|
|
|
|
static const struct drm_connector_funcs arcpgu_drm_connector_funcs = {
|
|
.dpms = drm_helper_connector_dpms,
|
|
.reset = drm_atomic_helper_connector_reset,
|
|
.fill_modes = drm_helper_probe_single_connector_modes,
|
|
.destroy = arcpgu_drm_connector_destroy,
|
|
.atomic_duplicate_state = drm_atomic_helper_connector_duplicate_state,
|
|
.atomic_destroy_state = drm_atomic_helper_connector_destroy_state,
|
|
};
|
|
|
|
static struct drm_encoder_funcs arcpgu_drm_encoder_funcs = {
|
|
.destroy = drm_encoder_cleanup,
|
|
};
|
|
|
|
int arcpgu_drm_sim_init(struct drm_device *drm, struct device_node *np)
|
|
{
|
|
struct arcpgu_drm_connector *arcpgu_connector;
|
|
struct drm_encoder_slave *encoder;
|
|
struct drm_connector *connector;
|
|
int ret;
|
|
|
|
encoder = devm_kzalloc(drm->dev, sizeof(*encoder), GFP_KERNEL);
|
|
if (encoder == NULL)
|
|
return -ENOMEM;
|
|
|
|
encoder->base.possible_crtcs = 1;
|
|
encoder->base.possible_clones = 0;
|
|
|
|
ret = drm_encoder_init(drm, &encoder->base, &arcpgu_drm_encoder_funcs,
|
|
DRM_MODE_ENCODER_VIRTUAL, NULL);
|
|
if (ret)
|
|
return ret;
|
|
|
|
arcpgu_connector = devm_kzalloc(drm->dev, sizeof(*arcpgu_connector),
|
|
GFP_KERNEL);
|
|
if (!arcpgu_connector) {
|
|
ret = -ENOMEM;
|
|
goto error_encoder_cleanup;
|
|
}
|
|
|
|
connector = &arcpgu_connector->connector;
|
|
drm_connector_helper_add(connector, &arcpgu_drm_connector_helper_funcs);
|
|
|
|
ret = drm_connector_init(drm, connector, &arcpgu_drm_connector_funcs,
|
|
DRM_MODE_CONNECTOR_VIRTUAL);
|
|
if (ret < 0) {
|
|
dev_err(drm->dev, "failed to initialize drm connector\n");
|
|
goto error_encoder_cleanup;
|
|
}
|
|
|
|
ret = drm_mode_connector_attach_encoder(connector, &encoder->base);
|
|
if (ret < 0) {
|
|
dev_err(drm->dev, "could not attach connector to encoder\n");
|
|
drm_connector_unregister(connector);
|
|
goto error_connector_cleanup;
|
|
}
|
|
|
|
arcpgu_connector->encoder_slave = encoder;
|
|
|
|
return 0;
|
|
|
|
error_connector_cleanup:
|
|
drm_connector_cleanup(connector);
|
|
|
|
error_encoder_cleanup:
|
|
drm_encoder_cleanup(&encoder->base);
|
|
return ret;
|
|
}
|