mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-22 02:20:40 +00:00
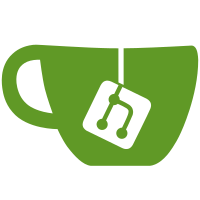
Based on 1 normalized pattern(s): this program is free software you can distribute it and or modify it under the terms of the gnu general public license version 2 as published by the free software foundation this program is distributed in the hope it will be useful but without any warranty without even the implied warranty of merchantability or fitness for a particular purpose see the gnu general public license for more details you should have received a copy of the gnu general public license along with this program if not write to the free software foundation inc 59 temple place suite 330 boston ma 02111 1307 usa extracted by the scancode license scanner the SPDX license identifier GPL-2.0-only has been chosen to replace the boilerplate/reference in 32 file(s). Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Reviewed-by: Allison Randal <allison@lohutok.net> Reviewed-by: Richard Fontana <rfontana@redhat.com> Reviewed-by: Steve Winslow <swinslow@gmail.com> Reviewed-by: Alexios Zavras <alexios.zavras@intel.com> Cc: linux-spdx@vger.kernel.org Link: https://lkml.kernel.org/r/20190528170026.531157061@linutronix.de Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
88 lines
2.1 KiB
C
88 lines
2.1 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* Setting up the clock on MSP SOCs. No RTC typically.
|
|
*
|
|
* Carsten Langgaard, carstenl@mips.com
|
|
* Copyright (C) 1999,2000 MIPS Technologies, Inc. All rights reserved.
|
|
*
|
|
* ########################################################################
|
|
*
|
|
* ########################################################################
|
|
*/
|
|
|
|
#include <linux/init.h>
|
|
#include <linux/kernel_stat.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/spinlock.h>
|
|
#include <linux/ptrace.h>
|
|
|
|
#include <asm/cevt-r4k.h>
|
|
#include <asm/mipsregs.h>
|
|
#include <asm/time.h>
|
|
|
|
#include <msp_prom.h>
|
|
#include <msp_int.h>
|
|
#include <msp_regs.h>
|
|
|
|
#define get_current_vpe() \
|
|
((read_c0_tcbind() >> TCBIND_CURVPE_SHIFT) & TCBIND_CURVPE)
|
|
|
|
static struct irqaction timer_vpe1;
|
|
static int tim_installed;
|
|
|
|
void __init plat_time_init(void)
|
|
{
|
|
char *endp, *s;
|
|
unsigned long cpu_rate = 0;
|
|
|
|
if (cpu_rate == 0) {
|
|
s = prom_getenv("clkfreqhz");
|
|
cpu_rate = simple_strtoul(s, &endp, 10);
|
|
if (endp != NULL && *endp != 0) {
|
|
printk(KERN_ERR
|
|
"Clock rate in Hz parse error: %s\n", s);
|
|
cpu_rate = 0;
|
|
}
|
|
}
|
|
|
|
if (cpu_rate == 0) {
|
|
s = prom_getenv("clkfreq");
|
|
cpu_rate = 1000 * simple_strtoul(s, &endp, 10);
|
|
if (endp != NULL && *endp != 0) {
|
|
printk(KERN_ERR
|
|
"Clock rate in MHz parse error: %s\n", s);
|
|
cpu_rate = 0;
|
|
}
|
|
}
|
|
|
|
if (cpu_rate == 0) {
|
|
#if defined(CONFIG_PMC_MSP7120_EVAL) \
|
|
|| defined(CONFIG_PMC_MSP7120_GW)
|
|
cpu_rate = 400000000;
|
|
#elif defined(CONFIG_PMC_MSP7120_FPGA)
|
|
cpu_rate = 25000000;
|
|
#else
|
|
cpu_rate = 150000000;
|
|
#endif
|
|
printk(KERN_ERR
|
|
"Failed to determine CPU clock rate, "
|
|
"assuming %ld hz ...\n", cpu_rate);
|
|
}
|
|
|
|
printk(KERN_WARNING "Clock rate set to %ld\n", cpu_rate);
|
|
|
|
/* timer frequency is 1/2 clock rate */
|
|
mips_hpt_frequency = cpu_rate/2;
|
|
}
|
|
|
|
unsigned int get_c0_compare_int(void)
|
|
{
|
|
/* MIPS_MT modes may want timer for second VPE */
|
|
if ((get_current_vpe()) && !tim_installed) {
|
|
memcpy(&timer_vpe1, &c0_compare_irqaction, sizeof(timer_vpe1));
|
|
setup_irq(MSP_INT_VPE1_TIMER, &timer_vpe1);
|
|
tim_installed++;
|
|
}
|
|
|
|
return get_current_vpe() ? MSP_INT_VPE1_TIMER : MSP_INT_VPE0_TIMER;
|
|
}
|