mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-26 10:49:33 +00:00
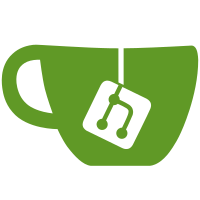
We keep having bug reports that when users build perf on their own, but they don't install some needed libraries such as libelf, libbfd/libibery. The perf can build, but it is missing important functionality. This patch provides a new option '-vv' for perf which will print the compiled-in status of libraries. The 'perf -vv' is mapped to 'perf version --build-options'. For example: $ ./perf -vv perf version 4.13.rc5.g6727c5 dwarf: [ on ] # HAVE_DWARF_SUPPORT dwarf_getlocations: [ on ] # HAVE_DWARF_GETLOCATIONS_SUPPORT glibc: [ on ] # HAVE_GLIBC_SUPPORT gtk2: [ on ] # HAVE_GTK2_SUPPORT libaudit: [ OFF ] # HAVE_LIBAUDIT_SUPPORT libbfd: [ on ] # HAVE_LIBBFD_SUPPORT libelf: [ on ] # HAVE_LIBELF_SUPPORT libnuma: [ on ] # HAVE_LIBNUMA_SUPPORT numa_num_possible_cpus: [ on ] # HAVE_LIBNUMA_SUPPORT libperl: [ on ] # HAVE_LIBPERL_SUPPORT libpython: [ on ] # HAVE_LIBPYTHON_SUPPORT libslang: [ on ] # HAVE_SLANG_SUPPORT libcrypto: [ on ] # HAVE_LIBCRYPTO_SUPPORT libunwind: [ on ] # HAVE_LIBUNWIND_SUPPORT libdw-dwarf-unwind: [ on ] # HAVE_DWARF_SUPPORT zlib: [ on ] # HAVE_ZLIB_SUPPORT lzma: [ on ] # HAVE_LZMA_SUPPORT get_cpuid: [ on ] # HAVE_AUXTRACE_SUPPORT bpf: [ on ] # HAVE_LIBBPF_SUPPORT v3: One bug is found in v2. It didn't process the option like '-vabc' correctly. Fix this bug. v2: Use a global variable version_verbose to record the number of 'v'. Signed-off-by: Jin Yao <yao.jin@linux.intel.com> Tested-by: Arnaldo Carvalho de Melo <acme@redhat.com> Acked-by: Jiri Olsa <jolsa@kernel.org> Cc: Alexander Shishkin <alexander.shishkin@linux.intel.com> Cc: Andi Kleen <ak@linux.intel.com> Cc: Jin Yao <yao.jin@intel.com> Cc: Kan Liang <kan.liang@intel.com> Cc: Peter Zijlstra <peterz@infradead.org> Link: http://lkml.kernel.org/r/1522402036-22915-6-git-send-email-yao.jin@linux.intel.com Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
90 lines
2.1 KiB
C
90 lines
2.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _PERF_PERF_H
|
|
#define _PERF_PERF_H
|
|
|
|
#include <time.h>
|
|
#include <stdbool.h>
|
|
#include <linux/types.h>
|
|
#include <linux/perf_event.h>
|
|
|
|
extern bool test_attr__enabled;
|
|
void test_attr__ready(void);
|
|
void test_attr__init(void);
|
|
void test_attr__open(struct perf_event_attr *attr, pid_t pid, int cpu,
|
|
int fd, int group_fd, unsigned long flags);
|
|
|
|
#define HAVE_ATTR_TEST
|
|
#include "perf-sys.h"
|
|
|
|
static inline unsigned long long rdclock(void)
|
|
{
|
|
struct timespec ts;
|
|
|
|
clock_gettime(CLOCK_MONOTONIC, &ts);
|
|
return ts.tv_sec * 1000000000ULL + ts.tv_nsec;
|
|
}
|
|
|
|
#define MAX_NR_CPUS 1024
|
|
|
|
extern const char *input_name;
|
|
extern bool perf_host, perf_guest;
|
|
extern const char perf_version_string[];
|
|
|
|
void pthread__unblock_sigwinch(void);
|
|
|
|
#include "util/target.h"
|
|
|
|
struct record_opts {
|
|
struct target target;
|
|
bool group;
|
|
bool inherit_stat;
|
|
bool no_buffering;
|
|
bool no_inherit;
|
|
bool no_inherit_set;
|
|
bool no_samples;
|
|
bool raw_samples;
|
|
bool sample_address;
|
|
bool sample_phys_addr;
|
|
bool sample_weight;
|
|
bool sample_time;
|
|
bool sample_time_set;
|
|
bool sample_cpu;
|
|
bool period;
|
|
bool period_set;
|
|
bool running_time;
|
|
bool full_auxtrace;
|
|
bool auxtrace_snapshot_mode;
|
|
bool record_namespaces;
|
|
bool record_switch_events;
|
|
bool all_kernel;
|
|
bool all_user;
|
|
bool tail_synthesize;
|
|
bool overwrite;
|
|
bool ignore_missing_thread;
|
|
bool strict_freq;
|
|
bool sample_id;
|
|
unsigned int freq;
|
|
unsigned int mmap_pages;
|
|
unsigned int auxtrace_mmap_pages;
|
|
unsigned int user_freq;
|
|
u64 branch_stack;
|
|
u64 sample_intr_regs;
|
|
u64 sample_user_regs;
|
|
u64 default_interval;
|
|
u64 user_interval;
|
|
size_t auxtrace_snapshot_size;
|
|
const char *auxtrace_snapshot_opts;
|
|
bool sample_transaction;
|
|
unsigned initial_delay;
|
|
bool use_clockid;
|
|
clockid_t clockid;
|
|
unsigned int proc_map_timeout;
|
|
};
|
|
|
|
struct option;
|
|
extern const char * const *record_usage;
|
|
extern struct option *record_options;
|
|
extern int version_verbose;
|
|
|
|
int record__parse_freq(const struct option *opt, const char *str, int unset);
|
|
#endif
|