mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 08:58:07 +00:00
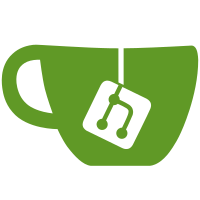
According to the comments in include/linux/init.h: "Pointers to __devexit functions must use __devexit_p(function_name), the wrapper will insert either the function_name or NULL, depending on the config options." We have __devexit annotation for wm8782_remove(), thus add __devexit_p at necessary place. Signed-off-by: Axel Lin <axel.lin@gmail.com> Signed-off-by: Mark Brown <broonie@opensource.wolfsonmicro.com>
80 lines
2 KiB
C
80 lines
2 KiB
C
/*
|
|
* sound/soc/codecs/wm8782.c
|
|
* simple, strap-pin configured 24bit 2ch ADC
|
|
*
|
|
* Copyright: 2011 Raumfeld GmbH
|
|
* Author: Johannes Stezenbach <js@sig21.net>
|
|
*
|
|
* based on ad73311.c
|
|
* Copyright: Analog Device Inc.
|
|
* Author: Cliff Cai <cliff.cai@analog.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by the
|
|
* Free Software Foundation; either version 2 of the License, or (at your
|
|
* option) any later version.
|
|
*/
|
|
|
|
#include <linux/init.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/module.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/device.h>
|
|
#include <sound/core.h>
|
|
#include <sound/pcm.h>
|
|
#include <sound/ac97_codec.h>
|
|
#include <sound/initval.h>
|
|
#include <sound/soc.h>
|
|
|
|
static struct snd_soc_dai_driver wm8782_dai = {
|
|
.name = "wm8782",
|
|
.capture = {
|
|
.stream_name = "Capture",
|
|
.channels_min = 2,
|
|
.channels_max = 2,
|
|
/* For configurations with FSAMPEN=0 */
|
|
.rates = SNDRV_PCM_RATE_8000_48000,
|
|
.formats = SNDRV_PCM_FMTBIT_S16_LE |
|
|
SNDRV_PCM_FMTBIT_S20_3LE |
|
|
SNDRV_PCM_FMTBIT_S24_LE,
|
|
},
|
|
};
|
|
|
|
static struct snd_soc_codec_driver soc_codec_dev_wm8782;
|
|
|
|
static __devinit int wm8782_probe(struct platform_device *pdev)
|
|
{
|
|
return snd_soc_register_codec(&pdev->dev,
|
|
&soc_codec_dev_wm8782, &wm8782_dai, 1);
|
|
}
|
|
|
|
static int __devexit wm8782_remove(struct platform_device *pdev)
|
|
{
|
|
snd_soc_unregister_codec(&pdev->dev);
|
|
return 0;
|
|
}
|
|
|
|
static struct platform_driver wm8782_codec_driver = {
|
|
.driver = {
|
|
.name = "wm8782",
|
|
.owner = THIS_MODULE,
|
|
},
|
|
.probe = wm8782_probe,
|
|
.remove = __devexit_p(wm8782_remove),
|
|
};
|
|
|
|
static int __init wm8782_init(void)
|
|
{
|
|
return platform_driver_register(&wm8782_codec_driver);
|
|
}
|
|
module_init(wm8782_init);
|
|
|
|
static void __exit wm8782_exit(void)
|
|
{
|
|
platform_driver_unregister(&wm8782_codec_driver);
|
|
}
|
|
module_exit(wm8782_exit);
|
|
|
|
MODULE_DESCRIPTION("ASoC WM8782 driver");
|
|
MODULE_AUTHOR("Johannes Stezenbach <js@sig21.net>");
|
|
MODULE_LICENSE("GPL");
|