mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 21:57:43 +00:00
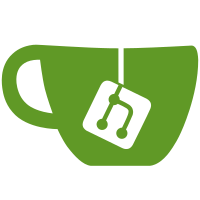
commit 3bd4abc07a
upstream.
In the event that random_get_entropy() can't access a cycle counter or
similar, falling back to returning 0 is suboptimal. Instead, fallback
to calling random_get_entropy_fallback(), which isn't extremely high
precision or guaranteed to be entropic, but is certainly better than
returning zero all the time.
If CONFIG_X86_TSC=n, then it's possible for the kernel to run on systems
without RDTSC, such as 486 and certain 586, so the fallback code is only
required for that case.
As well, fix up both the new function and the get_cycles() function from
which it was derived to use cpu_feature_enabled() rather than
boot_cpu_has(), and use !IS_ENABLED() instead of #ifndef.
Signed-off-by: Jason A. Donenfeld <Jason@zx2c4.com>
Reviewed-by: Thomas Gleixner <tglx@linutronix.de>
Cc: Thomas Gleixner <tglx@linutronix.de>
Cc: Arnd Bergmann <arnd@arndb.de>
Cc: Borislav Petkov <bp@alien8.de>
Cc: x86@kernel.org
Signed-off-by: Jason A. Donenfeld <Jason@zx2c4.com>
Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
75 lines
2 KiB
C
75 lines
2 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* x86 TSC related functions
|
|
*/
|
|
#ifndef _ASM_X86_TSC_H
|
|
#define _ASM_X86_TSC_H
|
|
|
|
#include <asm/processor.h>
|
|
|
|
#define NS_SCALE 10 /* 2^10, carefully chosen */
|
|
#define US_SCALE 32 /* 2^32, arbitralrily chosen */
|
|
|
|
/*
|
|
* Standard way to access the cycle counter.
|
|
*/
|
|
typedef unsigned long long cycles_t;
|
|
|
|
extern unsigned int cpu_khz;
|
|
extern unsigned int tsc_khz;
|
|
|
|
extern void disable_TSC(void);
|
|
|
|
static inline cycles_t get_cycles(void)
|
|
{
|
|
if (!IS_ENABLED(CONFIG_X86_TSC) &&
|
|
!cpu_feature_enabled(X86_FEATURE_TSC))
|
|
return 0;
|
|
return rdtsc();
|
|
}
|
|
#define get_cycles get_cycles
|
|
|
|
extern struct system_counterval_t convert_art_to_tsc(u64 art);
|
|
extern struct system_counterval_t convert_art_ns_to_tsc(u64 art_ns);
|
|
|
|
extern void tsc_early_init(void);
|
|
extern void tsc_init(void);
|
|
extern unsigned long calibrate_delay_is_known(void);
|
|
extern void mark_tsc_unstable(char *reason);
|
|
extern int unsynchronized_tsc(void);
|
|
extern int check_tsc_unstable(void);
|
|
extern void mark_tsc_async_resets(char *reason);
|
|
extern unsigned long native_calibrate_cpu_early(void);
|
|
extern unsigned long native_calibrate_tsc(void);
|
|
extern unsigned long long native_sched_clock_from_tsc(u64 tsc);
|
|
|
|
extern int tsc_clocksource_reliable;
|
|
#ifdef CONFIG_X86_TSC
|
|
extern bool tsc_async_resets;
|
|
#else
|
|
# define tsc_async_resets false
|
|
#endif
|
|
|
|
/*
|
|
* Boot-time check whether the TSCs are synchronized across
|
|
* all CPUs/cores:
|
|
*/
|
|
#ifdef CONFIG_X86_TSC
|
|
extern bool tsc_store_and_check_tsc_adjust(bool bootcpu);
|
|
extern void tsc_verify_tsc_adjust(bool resume);
|
|
extern void check_tsc_sync_source(int cpu);
|
|
extern void check_tsc_sync_target(void);
|
|
#else
|
|
static inline bool tsc_store_and_check_tsc_adjust(bool bootcpu) { return false; }
|
|
static inline void tsc_verify_tsc_adjust(bool resume) { }
|
|
static inline void check_tsc_sync_source(int cpu) { }
|
|
static inline void check_tsc_sync_target(void) { }
|
|
#endif
|
|
|
|
extern int notsc_setup(char *);
|
|
extern void tsc_save_sched_clock_state(void);
|
|
extern void tsc_restore_sched_clock_state(void);
|
|
|
|
unsigned long cpu_khz_from_msr(void);
|
|
|
|
#endif /* _ASM_X86_TSC_H */
|