mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-31 16:38:12 +00:00
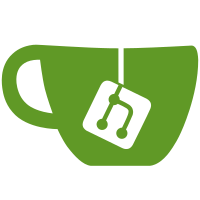
If used from KDB, the locked variants are prone to deadlocks (suppose we got to the debugger w/ the logbuf lock held). So, we have to implement a few routines that grab no logbuf lock. Yet we don't need these functions in modules, so we don't export them. Signed-off-by: Anton Vorontsov <anton.vorontsov@linaro.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
117 lines
2.9 KiB
C
117 lines
2.9 KiB
C
/*
|
|
* linux/include/kmsg_dump.h
|
|
*
|
|
* Copyright (C) 2009 Net Insight AB
|
|
*
|
|
* Author: Simon Kagstrom <simon.kagstrom@netinsight.net>
|
|
*
|
|
* This file is subject to the terms and conditions of the GNU General Public
|
|
* License. See the file COPYING in the main directory of this archive
|
|
* for more details.
|
|
*/
|
|
#ifndef _LINUX_KMSG_DUMP_H
|
|
#define _LINUX_KMSG_DUMP_H
|
|
|
|
#include <linux/errno.h>
|
|
#include <linux/list.h>
|
|
|
|
/*
|
|
* Keep this list arranged in rough order of priority. Anything listed after
|
|
* KMSG_DUMP_OOPS will not be logged by default unless printk.always_kmsg_dump
|
|
* is passed to the kernel.
|
|
*/
|
|
enum kmsg_dump_reason {
|
|
KMSG_DUMP_UNDEF,
|
|
KMSG_DUMP_PANIC,
|
|
KMSG_DUMP_OOPS,
|
|
KMSG_DUMP_EMERG,
|
|
KMSG_DUMP_RESTART,
|
|
KMSG_DUMP_HALT,
|
|
KMSG_DUMP_POWEROFF,
|
|
};
|
|
|
|
/**
|
|
* struct kmsg_dumper - kernel crash message dumper structure
|
|
* @list: Entry in the dumper list (private)
|
|
* @dump: Call into dumping code which will retrieve the data with
|
|
* through the record iterator
|
|
* @max_reason: filter for highest reason number that should be dumped
|
|
* @registered: Flag that specifies if this is already registered
|
|
*/
|
|
struct kmsg_dumper {
|
|
struct list_head list;
|
|
void (*dump)(struct kmsg_dumper *dumper, enum kmsg_dump_reason reason);
|
|
enum kmsg_dump_reason max_reason;
|
|
bool active;
|
|
bool registered;
|
|
|
|
/* private state of the kmsg iterator */
|
|
u32 cur_idx;
|
|
u32 next_idx;
|
|
u64 cur_seq;
|
|
u64 next_seq;
|
|
};
|
|
|
|
#ifdef CONFIG_PRINTK
|
|
void kmsg_dump(enum kmsg_dump_reason reason);
|
|
|
|
bool kmsg_dump_get_line_nolock(struct kmsg_dumper *dumper, bool syslog,
|
|
char *line, size_t size, size_t *len);
|
|
|
|
bool kmsg_dump_get_line(struct kmsg_dumper *dumper, bool syslog,
|
|
char *line, size_t size, size_t *len);
|
|
|
|
bool kmsg_dump_get_buffer(struct kmsg_dumper *dumper, bool syslog,
|
|
char *buf, size_t size, size_t *len);
|
|
|
|
void kmsg_dump_rewind_nolock(struct kmsg_dumper *dumper);
|
|
|
|
void kmsg_dump_rewind(struct kmsg_dumper *dumper);
|
|
|
|
int kmsg_dump_register(struct kmsg_dumper *dumper);
|
|
|
|
int kmsg_dump_unregister(struct kmsg_dumper *dumper);
|
|
#else
|
|
static inline void kmsg_dump(enum kmsg_dump_reason reason)
|
|
{
|
|
}
|
|
|
|
static inline bool kmsg_dump_get_line_nolock(struct kmsg_dumper *dumper,
|
|
bool syslog, const char *line,
|
|
size_t size, size_t *len)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
static inline bool kmsg_dump_get_line(struct kmsg_dumper *dumper, bool syslog,
|
|
const char *line, size_t size, size_t *len)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
static inline bool kmsg_dump_get_buffer(struct kmsg_dumper *dumper, bool syslog,
|
|
char *buf, size_t size, size_t *len)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
static inline void kmsg_dump_rewind_nolock(struct kmsg_dumper *dumper)
|
|
{
|
|
}
|
|
|
|
static inline void kmsg_dump_rewind(struct kmsg_dumper *dumper)
|
|
{
|
|
}
|
|
|
|
static inline int kmsg_dump_register(struct kmsg_dumper *dumper)
|
|
{
|
|
return -EINVAL;
|
|
}
|
|
|
|
static inline int kmsg_dump_unregister(struct kmsg_dumper *dumper)
|
|
{
|
|
return -EINVAL;
|
|
}
|
|
#endif
|
|
|
|
#endif /* _LINUX_KMSG_DUMP_H */
|