mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-31 16:38:12 +00:00
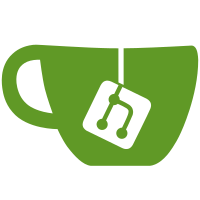
The rcu/sync code currently does a special check for being in an RCU read-side critical section. With RCU consolidating flavors and the generic helper added earlier in this series, this check is no longer need. This commit switches to the generic helper, saving a couple of lines of code. Cc: Oleg Nesterov <oleg@redhat.com> Acked-by: Oleg Nesterov <oleg@redhat.com> Signed-off-by: Joel Fernandes (Google) <joel@joelfernandes.org> Signed-off-by: Paul E. McKenney <paulmck@linux.ibm.com>
54 lines
1.5 KiB
C
54 lines
1.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* RCU-based infrastructure for lightweight reader-writer locking
|
|
*
|
|
* Copyright (c) 2015, Red Hat, Inc.
|
|
*
|
|
* Author: Oleg Nesterov <oleg@redhat.com>
|
|
*/
|
|
|
|
#ifndef _LINUX_RCU_SYNC_H_
|
|
#define _LINUX_RCU_SYNC_H_
|
|
|
|
#include <linux/wait.h>
|
|
#include <linux/rcupdate.h>
|
|
|
|
/* Structure to mediate between updaters and fastpath-using readers. */
|
|
struct rcu_sync {
|
|
int gp_state;
|
|
int gp_count;
|
|
wait_queue_head_t gp_wait;
|
|
|
|
struct rcu_head cb_head;
|
|
};
|
|
|
|
/**
|
|
* rcu_sync_is_idle() - Are readers permitted to use their fastpaths?
|
|
* @rsp: Pointer to rcu_sync structure to use for synchronization
|
|
*
|
|
* Returns true if readers are permitted to use their fastpaths. Must be
|
|
* invoked within some flavor of RCU read-side critical section.
|
|
*/
|
|
static inline bool rcu_sync_is_idle(struct rcu_sync *rsp)
|
|
{
|
|
RCU_LOCKDEP_WARN(!rcu_read_lock_any_held(),
|
|
"suspicious rcu_sync_is_idle() usage");
|
|
return !READ_ONCE(rsp->gp_state); /* GP_IDLE */
|
|
}
|
|
|
|
extern void rcu_sync_init(struct rcu_sync *);
|
|
extern void rcu_sync_enter_start(struct rcu_sync *);
|
|
extern void rcu_sync_enter(struct rcu_sync *);
|
|
extern void rcu_sync_exit(struct rcu_sync *);
|
|
extern void rcu_sync_dtor(struct rcu_sync *);
|
|
|
|
#define __RCU_SYNC_INITIALIZER(name) { \
|
|
.gp_state = 0, \
|
|
.gp_count = 0, \
|
|
.gp_wait = __WAIT_QUEUE_HEAD_INITIALIZER(name.gp_wait), \
|
|
}
|
|
|
|
#define DEFINE_RCU_SYNC(name) \
|
|
struct rcu_sync name = __RCU_SYNC_INITIALIZER(name)
|
|
|
|
#endif /* _LINUX_RCU_SYNC_H_ */
|