mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-31 16:38:12 +00:00
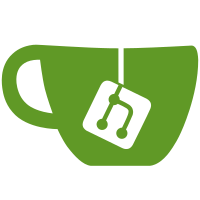
For spinlocks the type spinlock_t should be used instead of "struct spinlock". Use spinlock_t for spinlock's definition. Link: http://lkml.kernel.org/r/20190704153803.12739-3-bigeasy@linutronix.de Signed-off-by: Sebastian Andrzej Siewior <bigeasy@linutronix.de> Reviewed-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
52 lines
1.7 KiB
C
52 lines
1.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef __LINUX_VMPRESSURE_H
|
|
#define __LINUX_VMPRESSURE_H
|
|
|
|
#include <linux/mutex.h>
|
|
#include <linux/list.h>
|
|
#include <linux/workqueue.h>
|
|
#include <linux/gfp.h>
|
|
#include <linux/types.h>
|
|
#include <linux/cgroup.h>
|
|
#include <linux/eventfd.h>
|
|
|
|
struct vmpressure {
|
|
unsigned long scanned;
|
|
unsigned long reclaimed;
|
|
|
|
unsigned long tree_scanned;
|
|
unsigned long tree_reclaimed;
|
|
/* The lock is used to keep the scanned/reclaimed above in sync. */
|
|
spinlock_t sr_lock;
|
|
|
|
/* The list of vmpressure_event structs. */
|
|
struct list_head events;
|
|
/* Have to grab the lock on events traversal or modifications. */
|
|
struct mutex events_lock;
|
|
|
|
struct work_struct work;
|
|
};
|
|
|
|
struct mem_cgroup;
|
|
|
|
#ifdef CONFIG_MEMCG
|
|
extern void vmpressure(gfp_t gfp, struct mem_cgroup *memcg, bool tree,
|
|
unsigned long scanned, unsigned long reclaimed);
|
|
extern void vmpressure_prio(gfp_t gfp, struct mem_cgroup *memcg, int prio);
|
|
|
|
extern void vmpressure_init(struct vmpressure *vmpr);
|
|
extern void vmpressure_cleanup(struct vmpressure *vmpr);
|
|
extern struct vmpressure *memcg_to_vmpressure(struct mem_cgroup *memcg);
|
|
extern struct cgroup_subsys_state *vmpressure_to_css(struct vmpressure *vmpr);
|
|
extern int vmpressure_register_event(struct mem_cgroup *memcg,
|
|
struct eventfd_ctx *eventfd,
|
|
const char *args);
|
|
extern void vmpressure_unregister_event(struct mem_cgroup *memcg,
|
|
struct eventfd_ctx *eventfd);
|
|
#else
|
|
static inline void vmpressure(gfp_t gfp, struct mem_cgroup *memcg, bool tree,
|
|
unsigned long scanned, unsigned long reclaimed) {}
|
|
static inline void vmpressure_prio(gfp_t gfp, struct mem_cgroup *memcg,
|
|
int prio) {}
|
|
#endif /* CONFIG_MEMCG */
|
|
#endif /* __LINUX_VMPRESSURE_H */
|