mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
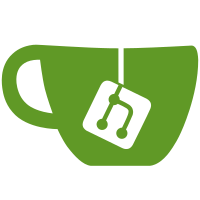
The driver was originally written with the assumption that a different API must be used for DMA-related functions if the device is PCIe based or if not. Since Xillybus' driver supports devices on a PCIe bus (with xillybus_pcie) as well as connected directly to the processor (with xillybus_of), it originally used wrapper functions that ensure that a different API is used for each. This patch eliminates the said wrapper functions, as all use the same dma_* API now. This is most notable by the code deleted in xillybus_pcie.c and xillybus_of.c. It also eliminates the OF driver's check for a "dma-coherent" attribute in the device's OF entry, since this is taken care of by the kernel's implementation of dma_sync_single_for_*(). There is however still need for one wrapper function, which is merged from xillybus_pcie.c and xillybus_of.c into xillybus_core.c: The call to dma_map_single() is wrapped by a function that uses the Managed Device (devres) framework, in the absence of a relevant function in the current kernel's API. Suggested-by: Christophe JAILLET <christophe.jaillet@wanadoo.fr> Suggested-by: Arnd Bergmann <arnd@arndb.de> Reviewed-by: Arnd Bergmann <arnd@arndb.de> Signed-off-by: Eli Billauer <eli.billauer@gmail.com> Link: https://lore.kernel.org/r/20210929094442.46383-1-eli.billauer@gmail.com Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
127 lines
3.1 KiB
C
127 lines
3.1 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* linux/drivers/misc/xillybus_pcie.c
|
|
*
|
|
* Copyright 2011 Xillybus Ltd, http://xillybus.com
|
|
*
|
|
* Driver for the Xillybus FPGA/host framework using PCI Express.
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/pci.h>
|
|
#include <linux/slab.h>
|
|
#include "xillybus.h"
|
|
|
|
MODULE_DESCRIPTION("Xillybus driver for PCIe");
|
|
MODULE_AUTHOR("Eli Billauer, Xillybus Ltd.");
|
|
MODULE_ALIAS("xillybus_pcie");
|
|
MODULE_LICENSE("GPL v2");
|
|
|
|
#define PCI_DEVICE_ID_XILLYBUS 0xebeb
|
|
|
|
#define PCI_VENDOR_ID_ACTEL 0x11aa
|
|
#define PCI_VENDOR_ID_LATTICE 0x1204
|
|
|
|
static const char xillyname[] = "xillybus_pcie";
|
|
|
|
static const struct pci_device_id xillyids[] = {
|
|
{PCI_DEVICE(PCI_VENDOR_ID_XILINX, PCI_DEVICE_ID_XILLYBUS)},
|
|
{PCI_DEVICE(PCI_VENDOR_ID_ALTERA, PCI_DEVICE_ID_XILLYBUS)},
|
|
{PCI_DEVICE(PCI_VENDOR_ID_ACTEL, PCI_DEVICE_ID_XILLYBUS)},
|
|
{PCI_DEVICE(PCI_VENDOR_ID_LATTICE, PCI_DEVICE_ID_XILLYBUS)},
|
|
{ /* End: all zeroes */ }
|
|
};
|
|
|
|
static int xilly_probe(struct pci_dev *pdev,
|
|
const struct pci_device_id *ent)
|
|
{
|
|
struct xilly_endpoint *endpoint;
|
|
int rc;
|
|
|
|
endpoint = xillybus_init_endpoint(&pdev->dev);
|
|
|
|
if (!endpoint)
|
|
return -ENOMEM;
|
|
|
|
pci_set_drvdata(pdev, endpoint);
|
|
|
|
endpoint->owner = THIS_MODULE;
|
|
|
|
rc = pcim_enable_device(pdev);
|
|
if (rc) {
|
|
dev_err(endpoint->dev,
|
|
"pcim_enable_device() failed. Aborting.\n");
|
|
return rc;
|
|
}
|
|
|
|
/* L0s has caused packet drops. No power saving, thank you. */
|
|
|
|
pci_disable_link_state(pdev, PCIE_LINK_STATE_L0S);
|
|
|
|
if (!(pci_resource_flags(pdev, 0) & IORESOURCE_MEM)) {
|
|
dev_err(endpoint->dev,
|
|
"Incorrect BAR configuration. Aborting.\n");
|
|
return -ENODEV;
|
|
}
|
|
|
|
rc = pcim_iomap_regions(pdev, 0x01, xillyname);
|
|
if (rc) {
|
|
dev_err(endpoint->dev,
|
|
"pcim_iomap_regions() failed. Aborting.\n");
|
|
return rc;
|
|
}
|
|
|
|
endpoint->registers = pcim_iomap_table(pdev)[0];
|
|
|
|
pci_set_master(pdev);
|
|
|
|
/* Set up a single MSI interrupt */
|
|
if (pci_enable_msi(pdev)) {
|
|
dev_err(endpoint->dev,
|
|
"Failed to enable MSI interrupts. Aborting.\n");
|
|
return -ENODEV;
|
|
}
|
|
rc = devm_request_irq(&pdev->dev, pdev->irq, xillybus_isr, 0,
|
|
xillyname, endpoint);
|
|
if (rc) {
|
|
dev_err(endpoint->dev,
|
|
"Failed to register MSI handler. Aborting.\n");
|
|
return -ENODEV;
|
|
}
|
|
|
|
/*
|
|
* Some (old and buggy?) hardware drops 64-bit addressed PCIe packets,
|
|
* even when the PCIe driver claims that a 64-bit mask is OK. On the
|
|
* other hand, on some architectures, 64-bit addressing is mandatory.
|
|
* So go for the 64-bit mask only when failing is the other option.
|
|
*/
|
|
|
|
if (!dma_set_mask(&pdev->dev, DMA_BIT_MASK(32))) {
|
|
endpoint->dma_using_dac = 0;
|
|
} else if (!dma_set_mask(&pdev->dev, DMA_BIT_MASK(64))) {
|
|
endpoint->dma_using_dac = 1;
|
|
} else {
|
|
dev_err(endpoint->dev, "Failed to set DMA mask. Aborting.\n");
|
|
return -ENODEV;
|
|
}
|
|
|
|
return xillybus_endpoint_discovery(endpoint);
|
|
}
|
|
|
|
static void xilly_remove(struct pci_dev *pdev)
|
|
{
|
|
struct xilly_endpoint *endpoint = pci_get_drvdata(pdev);
|
|
|
|
xillybus_endpoint_remove(endpoint);
|
|
}
|
|
|
|
MODULE_DEVICE_TABLE(pci, xillyids);
|
|
|
|
static struct pci_driver xillybus_driver = {
|
|
.name = xillyname,
|
|
.id_table = xillyids,
|
|
.probe = xilly_probe,
|
|
.remove = xilly_remove,
|
|
};
|
|
|
|
module_pci_driver(xillybus_driver);
|