mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
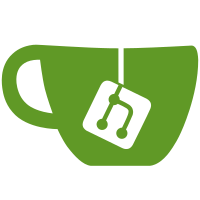
There is no option to perform 64bit integer sqrt on 32bit platform. Added stronger typed int_sqrt64 enables the 64bit calculations to be performed on 32bit platforms. Using same algorithm as int_sqrt() with strong typing provides enough precision also on 32bit platforms, but it sacrifices some performance. In case values are smaller than ULONG_MAX the standard int_sqrt is used for calculation to maximize the performance due to more native calculations. Signed-off-by: Crt Mori <cmo@melexis.com> Acked-by: Joe Perches <joe@perches.com> Signed-off-by: Jonathan Cameron <Jonathan.Cameron@huawei.com>
70 lines
1.1 KiB
C
70 lines
1.1 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (C) 2013 Davidlohr Bueso <davidlohr.bueso@hp.com>
|
|
*
|
|
* Based on the shift-and-subtract algorithm for computing integer
|
|
* square root from Guy L. Steele.
|
|
*/
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/export.h>
|
|
#include <linux/bitops.h>
|
|
|
|
/**
|
|
* int_sqrt - computes the integer square root
|
|
* @x: integer of which to calculate the sqrt
|
|
*
|
|
* Computes: floor(sqrt(x))
|
|
*/
|
|
unsigned long int_sqrt(unsigned long x)
|
|
{
|
|
unsigned long b, m, y = 0;
|
|
|
|
if (x <= 1)
|
|
return x;
|
|
|
|
m = 1UL << (__fls(x) & ~1UL);
|
|
while (m != 0) {
|
|
b = y + m;
|
|
y >>= 1;
|
|
|
|
if (x >= b) {
|
|
x -= b;
|
|
y += m;
|
|
}
|
|
m >>= 2;
|
|
}
|
|
|
|
return y;
|
|
}
|
|
EXPORT_SYMBOL(int_sqrt);
|
|
|
|
#if BITS_PER_LONG < 64
|
|
/**
|
|
* int_sqrt64 - strongly typed int_sqrt function when minimum 64 bit input
|
|
* is expected.
|
|
* @x: 64bit integer of which to calculate the sqrt
|
|
*/
|
|
u32 int_sqrt64(u64 x)
|
|
{
|
|
u64 b, m, y = 0;
|
|
|
|
if (x <= ULONG_MAX)
|
|
return int_sqrt((unsigned long) x);
|
|
|
|
m = 1ULL << (fls64(x) & ~1ULL);
|
|
while (m != 0) {
|
|
b = y + m;
|
|
y >>= 1;
|
|
|
|
if (x >= b) {
|
|
x -= b;
|
|
y += m;
|
|
}
|
|
m >>= 2;
|
|
}
|
|
|
|
return y;
|
|
}
|
|
EXPORT_SYMBOL(int_sqrt64);
|
|
#endif
|