mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
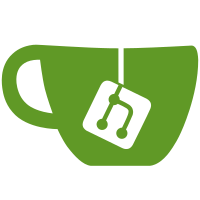
Since all users are now converted to the inline implementation, remove the out-of-line implementation entirely. Signed-off-by: Johannes Berg <johannes.berg@intel.com> Signed-off-by: David S. Miller <davem@davemloft.net>
45 lines
1.5 KiB
C
45 lines
1.5 KiB
C
#ifndef _LINUX_AVERAGE_H
|
|
#define _LINUX_AVERAGE_H
|
|
|
|
/* Exponentially weighted moving average (EWMA) */
|
|
|
|
#define DECLARE_EWMA(name, _factor, _weight) \
|
|
struct ewma_##name { \
|
|
unsigned long internal; \
|
|
}; \
|
|
static inline void ewma_##name##_init(struct ewma_##name *e) \
|
|
{ \
|
|
BUILD_BUG_ON(!__builtin_constant_p(_factor)); \
|
|
BUILD_BUG_ON(!__builtin_constant_p(_weight)); \
|
|
BUILD_BUG_ON_NOT_POWER_OF_2(_factor); \
|
|
BUILD_BUG_ON_NOT_POWER_OF_2(_weight); \
|
|
e->internal = 0; \
|
|
} \
|
|
static inline unsigned long \
|
|
ewma_##name##_read(struct ewma_##name *e) \
|
|
{ \
|
|
BUILD_BUG_ON(!__builtin_constant_p(_factor)); \
|
|
BUILD_BUG_ON(!__builtin_constant_p(_weight)); \
|
|
BUILD_BUG_ON_NOT_POWER_OF_2(_factor); \
|
|
BUILD_BUG_ON_NOT_POWER_OF_2(_weight); \
|
|
return e->internal >> ilog2(_factor); \
|
|
} \
|
|
static inline void ewma_##name##_add(struct ewma_##name *e, \
|
|
unsigned long val) \
|
|
{ \
|
|
unsigned long internal = ACCESS_ONCE(e->internal); \
|
|
unsigned long weight = ilog2(_weight); \
|
|
unsigned long factor = ilog2(_factor); \
|
|
\
|
|
BUILD_BUG_ON(!__builtin_constant_p(_factor)); \
|
|
BUILD_BUG_ON(!__builtin_constant_p(_weight)); \
|
|
BUILD_BUG_ON_NOT_POWER_OF_2(_factor); \
|
|
BUILD_BUG_ON_NOT_POWER_OF_2(_weight); \
|
|
\
|
|
ACCESS_ONCE(e->internal) = internal ? \
|
|
(((internal << weight) - internal) + \
|
|
(val << factor)) >> weight : \
|
|
(val << factor); \
|
|
}
|
|
|
|
#endif /* _LINUX_AVERAGE_H */
|