mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
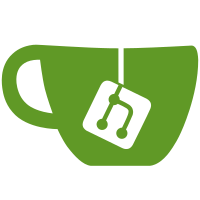
Pull vfs updates from Al Viro: "Assorted stuff; the biggest pile here is Christoph's ACL series. Plus assorted cleanups and fixes all over the place... There will be another pile later this week" * 'for-linus' of git://git.kernel.org/pub/scm/linux/kernel/git/viro/vfs: (43 commits) __dentry_path() fixes vfs: Remove second variable named error in __dentry_path vfs: Is mounted should be testing mnt_ns for NULL or error. Fix race when checking i_size on direct i/o read hfsplus: remove can_set_xattr nfsd: use get_acl and ->set_acl fs: remove generic_acl nfs: use generic posix ACL infrastructure for v3 Posix ACLs gfs2: use generic posix ACL infrastructure jfs: use generic posix ACL infrastructure xfs: use generic posix ACL infrastructure reiserfs: use generic posix ACL infrastructure ocfs2: use generic posix ACL infrastructure jffs2: use generic posix ACL infrastructure hfsplus: use generic posix ACL infrastructure f2fs: use generic posix ACL infrastructure ext2/3/4: use generic posix ACL infrastructure btrfs: use generic posix ACL infrastructure fs: make posix_acl_create more useful fs: make posix_acl_chmod more useful ...
144 lines
3.5 KiB
C
144 lines
3.5 KiB
C
/*
|
|
File: linux/posix_acl.h
|
|
|
|
(C) 2002 Andreas Gruenbacher, <a.gruenbacher@computer.org>
|
|
*/
|
|
|
|
|
|
#ifndef __LINUX_POSIX_ACL_H
|
|
#define __LINUX_POSIX_ACL_H
|
|
|
|
#include <linux/bug.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/rcupdate.h>
|
|
|
|
#define ACL_UNDEFINED_ID (-1)
|
|
|
|
/* a_type field in acl_user_posix_entry_t */
|
|
#define ACL_TYPE_ACCESS (0x8000)
|
|
#define ACL_TYPE_DEFAULT (0x4000)
|
|
|
|
/* e_tag entry in struct posix_acl_entry */
|
|
#define ACL_USER_OBJ (0x01)
|
|
#define ACL_USER (0x02)
|
|
#define ACL_GROUP_OBJ (0x04)
|
|
#define ACL_GROUP (0x08)
|
|
#define ACL_MASK (0x10)
|
|
#define ACL_OTHER (0x20)
|
|
|
|
/* permissions in the e_perm field */
|
|
#define ACL_READ (0x04)
|
|
#define ACL_WRITE (0x02)
|
|
#define ACL_EXECUTE (0x01)
|
|
//#define ACL_ADD (0x08)
|
|
//#define ACL_DELETE (0x10)
|
|
|
|
struct posix_acl_entry {
|
|
short e_tag;
|
|
unsigned short e_perm;
|
|
union {
|
|
kuid_t e_uid;
|
|
kgid_t e_gid;
|
|
};
|
|
};
|
|
|
|
struct posix_acl {
|
|
union {
|
|
atomic_t a_refcount;
|
|
struct rcu_head a_rcu;
|
|
};
|
|
unsigned int a_count;
|
|
struct posix_acl_entry a_entries[0];
|
|
};
|
|
|
|
#define FOREACH_ACL_ENTRY(pa, acl, pe) \
|
|
for(pa=(acl)->a_entries, pe=pa+(acl)->a_count; pa<pe; pa++)
|
|
|
|
|
|
/*
|
|
* Duplicate an ACL handle.
|
|
*/
|
|
static inline struct posix_acl *
|
|
posix_acl_dup(struct posix_acl *acl)
|
|
{
|
|
if (acl)
|
|
atomic_inc(&acl->a_refcount);
|
|
return acl;
|
|
}
|
|
|
|
/*
|
|
* Free an ACL handle.
|
|
*/
|
|
static inline void
|
|
posix_acl_release(struct posix_acl *acl)
|
|
{
|
|
if (acl && atomic_dec_and_test(&acl->a_refcount))
|
|
kfree_rcu(acl, a_rcu);
|
|
}
|
|
|
|
|
|
/* posix_acl.c */
|
|
|
|
extern void posix_acl_init(struct posix_acl *, int);
|
|
extern struct posix_acl *posix_acl_alloc(int, gfp_t);
|
|
extern int posix_acl_valid(const struct posix_acl *);
|
|
extern int posix_acl_permission(struct inode *, const struct posix_acl *, int);
|
|
extern struct posix_acl *posix_acl_from_mode(umode_t, gfp_t);
|
|
extern int posix_acl_equiv_mode(const struct posix_acl *, umode_t *);
|
|
extern int __posix_acl_create(struct posix_acl **, gfp_t, umode_t *);
|
|
extern int __posix_acl_chmod(struct posix_acl **, gfp_t, umode_t);
|
|
|
|
extern struct posix_acl *get_posix_acl(struct inode *, int);
|
|
extern int set_posix_acl(struct inode *, int, struct posix_acl *);
|
|
|
|
#ifdef CONFIG_FS_POSIX_ACL
|
|
extern int posix_acl_chmod(struct inode *, umode_t);
|
|
extern int posix_acl_create(struct inode *, umode_t *, struct posix_acl **,
|
|
struct posix_acl **);
|
|
|
|
extern int simple_set_acl(struct inode *, struct posix_acl *, int);
|
|
extern int simple_acl_create(struct inode *, struct inode *);
|
|
|
|
struct posix_acl **acl_by_type(struct inode *inode, int type);
|
|
struct posix_acl *get_cached_acl(struct inode *inode, int type);
|
|
struct posix_acl *get_cached_acl_rcu(struct inode *inode, int type);
|
|
void set_cached_acl(struct inode *inode, int type, struct posix_acl *acl);
|
|
void forget_cached_acl(struct inode *inode, int type);
|
|
void forget_all_cached_acls(struct inode *inode);
|
|
|
|
static inline void cache_no_acl(struct inode *inode)
|
|
{
|
|
inode->i_acl = NULL;
|
|
inode->i_default_acl = NULL;
|
|
}
|
|
#else
|
|
static inline int posix_acl_chmod(struct inode *inode, umode_t mode)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
#define simple_set_acl NULL
|
|
|
|
static inline int simple_acl_create(struct inode *dir, struct inode *inode)
|
|
{
|
|
return 0;
|
|
}
|
|
static inline void cache_no_acl(struct inode *inode)
|
|
{
|
|
}
|
|
|
|
static inline int posix_acl_create(struct inode *inode, umode_t *mode,
|
|
struct posix_acl **default_acl, struct posix_acl **acl)
|
|
{
|
|
*default_acl = *acl = NULL;
|
|
return 0;
|
|
}
|
|
|
|
static inline void forget_all_cached_acls(struct inode *inode)
|
|
{
|
|
}
|
|
#endif /* CONFIG_FS_POSIX_ACL */
|
|
|
|
struct posix_acl *get_acl(struct inode *inode, int type);
|
|
|
|
#endif /* __LINUX_POSIX_ACL_H */
|