mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-21 10:01:00 +00:00
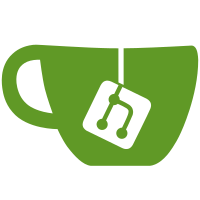
Instead of only passing the test case name and ID, pass the entire current test case down to the plugins. This change allows plugins to start accepting commands and directives from the test cases themselves, for greater flexibility in testing. Signed-off-by: Lucas Bates <lucasb@mojatatu.com> Signed-off-by: David S. Miller <davem@davemloft.net>
74 lines
2.4 KiB
Python
74 lines
2.4 KiB
Python
#!/usr/bin/env python3
|
|
|
|
class TdcPlugin:
|
|
def __init__(self):
|
|
super().__init__()
|
|
print(' -- {}.__init__'.format(self.sub_class))
|
|
|
|
def pre_suite(self, testcount, testidlist):
|
|
'''run commands before test_runner goes into a test loop'''
|
|
self.testcount = testcount
|
|
self.testidlist = testidlist
|
|
if self.args.verbose > 1:
|
|
print(' -- {}.pre_suite'.format(self.sub_class))
|
|
|
|
def post_suite(self, index):
|
|
'''run commands after test_runner completes the test loop
|
|
index is the last ordinal number of test that was attempted'''
|
|
if self.args.verbose > 1:
|
|
print(' -- {}.post_suite'.format(self.sub_class))
|
|
|
|
def pre_case(self, caseinfo, test_skip):
|
|
'''run commands before test_runner does one test'''
|
|
if self.args.verbose > 1:
|
|
print(' -- {}.pre_case'.format(self.sub_class))
|
|
self.args.caseinfo = caseinfo
|
|
self.args.test_skip = test_skip
|
|
|
|
def post_case(self):
|
|
'''run commands after test_runner does one test'''
|
|
if self.args.verbose > 1:
|
|
print(' -- {}.post_case'.format(self.sub_class))
|
|
|
|
def pre_execute(self):
|
|
'''run command before test-runner does the execute step'''
|
|
if self.args.verbose > 1:
|
|
print(' -- {}.pre_execute'.format(self.sub_class))
|
|
|
|
def post_execute(self):
|
|
'''run command after test-runner does the execute step'''
|
|
if self.args.verbose > 1:
|
|
print(' -- {}.post_execute'.format(self.sub_class))
|
|
|
|
def adjust_command(self, stage, command):
|
|
'''adjust the command'''
|
|
if self.args.verbose > 1:
|
|
print(' -- {}.adjust_command {}'.format(self.sub_class, stage))
|
|
|
|
# if stage == 'pre':
|
|
# pass
|
|
# elif stage == 'setup':
|
|
# pass
|
|
# elif stage == 'execute':
|
|
# pass
|
|
# elif stage == 'verify':
|
|
# pass
|
|
# elif stage == 'teardown':
|
|
# pass
|
|
# elif stage == 'post':
|
|
# pass
|
|
# else:
|
|
# pass
|
|
|
|
return command
|
|
|
|
def add_args(self, parser):
|
|
'''Get the plugin args from the command line'''
|
|
self.argparser = parser
|
|
return self.argparser
|
|
|
|
def check_args(self, args, remaining):
|
|
'''Check that the args are set correctly'''
|
|
self.args = args
|
|
if self.args.verbose > 1:
|
|
print(' -- {}.check_args'.format(self.sub_class))
|