mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-14 06:35:12 +00:00
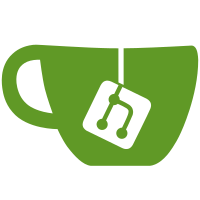
Having REQ_F_POLLED set doesn't guarantee that the request is
executed as a multishot from the polling path. Fortunately for us, if
the code thinks it's multishot issue when it's not, it can only ask to
skip completion so leaking the request. Use issue_flags to mark
multipoll issues.
Cc: stable@vger.kernel.org
Fixes: 390ed29b5e
("io_uring: add IORING_ACCEPT_MULTISHOT for accept")
Signed-off-by: Pavel Begunkov <asml.silence@gmail.com>
Link: https://lore.kernel.org/r/7700ac57653f2823e30b34dc74da68678c0c5f13.1668710222.git.asml.silence@gmail.com
Signed-off-by: Jens Axboe <axboe@kernel.dk>
100 lines
2.5 KiB
C
100 lines
2.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
#ifndef _LINUX_IO_URING_H
|
|
#define _LINUX_IO_URING_H
|
|
|
|
#include <linux/sched.h>
|
|
#include <linux/xarray.h>
|
|
#include <uapi/linux/io_uring.h>
|
|
|
|
enum io_uring_cmd_flags {
|
|
IO_URING_F_COMPLETE_DEFER = 1,
|
|
IO_URING_F_UNLOCKED = 2,
|
|
/* int's last bit, sign checks are usually faster than a bit test */
|
|
IO_URING_F_NONBLOCK = INT_MIN,
|
|
|
|
/* ctx state flags, for URING_CMD */
|
|
IO_URING_F_SQE128 = 4,
|
|
IO_URING_F_CQE32 = 8,
|
|
IO_URING_F_IOPOLL = 16,
|
|
|
|
/* the request is executed from poll, it should not be freed */
|
|
IO_URING_F_MULTISHOT = 32,
|
|
};
|
|
|
|
struct io_uring_cmd {
|
|
struct file *file;
|
|
const void *cmd;
|
|
union {
|
|
/* callback to defer completions to task context */
|
|
void (*task_work_cb)(struct io_uring_cmd *cmd);
|
|
/* used for polled completion */
|
|
void *cookie;
|
|
};
|
|
u32 cmd_op;
|
|
u32 flags;
|
|
u8 pdu[32]; /* available inline for free use */
|
|
};
|
|
|
|
#if defined(CONFIG_IO_URING)
|
|
int io_uring_cmd_import_fixed(u64 ubuf, unsigned long len, int rw,
|
|
struct iov_iter *iter, void *ioucmd);
|
|
void io_uring_cmd_done(struct io_uring_cmd *cmd, ssize_t ret, ssize_t res2);
|
|
void io_uring_cmd_complete_in_task(struct io_uring_cmd *ioucmd,
|
|
void (*task_work_cb)(struct io_uring_cmd *));
|
|
struct sock *io_uring_get_socket(struct file *file);
|
|
void __io_uring_cancel(bool cancel_all);
|
|
void __io_uring_free(struct task_struct *tsk);
|
|
void io_uring_unreg_ringfd(void);
|
|
const char *io_uring_get_opcode(u8 opcode);
|
|
|
|
static inline void io_uring_files_cancel(void)
|
|
{
|
|
if (current->io_uring) {
|
|
io_uring_unreg_ringfd();
|
|
__io_uring_cancel(false);
|
|
}
|
|
}
|
|
static inline void io_uring_task_cancel(void)
|
|
{
|
|
if (current->io_uring)
|
|
__io_uring_cancel(true);
|
|
}
|
|
static inline void io_uring_free(struct task_struct *tsk)
|
|
{
|
|
if (tsk->io_uring)
|
|
__io_uring_free(tsk);
|
|
}
|
|
#else
|
|
static inline int io_uring_cmd_import_fixed(u64 ubuf, unsigned long len, int rw,
|
|
struct iov_iter *iter, void *ioucmd)
|
|
{
|
|
return -EOPNOTSUPP;
|
|
}
|
|
static inline void io_uring_cmd_done(struct io_uring_cmd *cmd, ssize_t ret,
|
|
ssize_t ret2)
|
|
{
|
|
}
|
|
static inline void io_uring_cmd_complete_in_task(struct io_uring_cmd *ioucmd,
|
|
void (*task_work_cb)(struct io_uring_cmd *))
|
|
{
|
|
}
|
|
static inline struct sock *io_uring_get_socket(struct file *file)
|
|
{
|
|
return NULL;
|
|
}
|
|
static inline void io_uring_task_cancel(void)
|
|
{
|
|
}
|
|
static inline void io_uring_files_cancel(void)
|
|
{
|
|
}
|
|
static inline void io_uring_free(struct task_struct *tsk)
|
|
{
|
|
}
|
|
static inline const char *io_uring_get_opcode(u8 opcode)
|
|
{
|
|
return "";
|
|
}
|
|
#endif
|
|
|
|
#endif
|